検出対象を予め子の群としてまとめておき、親オブジェクトを取得。
親オブジェクトから子の情報を取得し、各子オブジェクトの座標と検出元とするオブジェクトとの
位置を比較し、距離を割り出す。その中で最も距離が近いオブジェクトを戻り値とする。
この検出方法の利点はCollider等の範囲で入っている物を検出すると、検出対象が範囲内に複数個ある時、結局距離で比較する必要が生まれる。
対して座標で比較するので、戻り値は必ず一つとなる。
(ただし最も近いオブジェクトが同じ座標に複数個ある場合は、最初に検出したオブジェクトが戻り値となる)
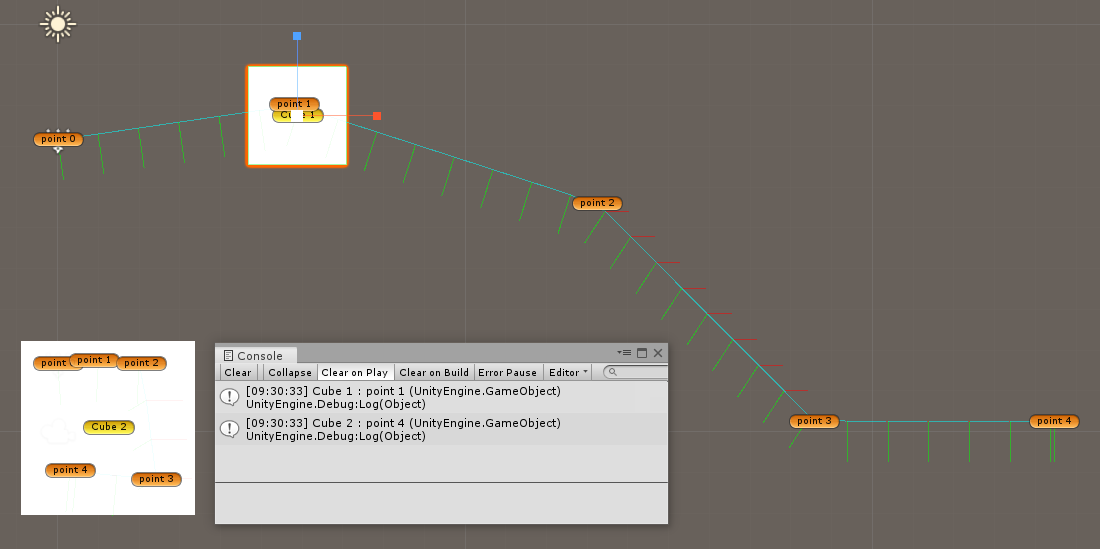
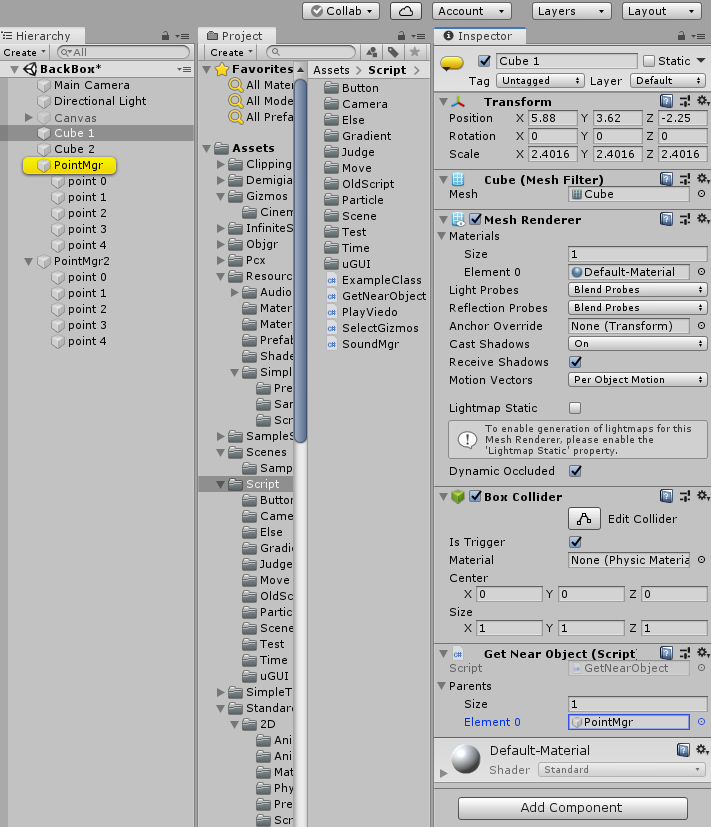
GetChild.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class GetChild : MonoBehaviour
{
public GameObject[] Parent{get; set;}
public List<GameObject> Panels{get; set;}
public virtual void GetAllChild()
{
Panels = new List<GameObject>();
foreach(GameObject p in Parent)
{
foreach(Transform child in p.transform)
{
Panels.Add(child.gameObject);
}
}
}
}
GetNearObject.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class GetNearObject : GetChild
{
public GameObject[] Parents;
private GameObject nearOb;
public override void GetAllChild()
{
Parent = Parents;
base.GetAllChild();
}
void Awake()
{
GetAllChild();
nearOb = nearObject();
Debug.Log(gameObject.name + " : " + nearOb);
}
GameObject nearObject()
{
float tmpDis = 0; //距離用一時変数
float nearDis = 0; //最も近いオブジェクトの距離
//string nearObjName = ""; //オブジェクト名称
GameObject targetObj = null; //オブジェクト
foreach(GameObject obs in Panels)
{
tmpDis = Vector3.Distance(obs.transform.position, transform.position);
//オブジェクトの距離が近いか、距離0であればオブジェクト名を取得
//一時変数に距離を格納
if (nearDis == 0 || nearDis > tmpDis){
nearDis = tmpDis;
//nearObjName = obs.name;
targetObj = obs;
}
}
return targetObj;
}
}