質問編集履歴
3
修正
test
CHANGED
File without changes
|
test
CHANGED
@@ -23,100 +23,7 @@
|
|
23
23
|
Failed copying input tensor from /job:localhost/replica:0/task:0/device:CPU:0 to /job:localhost/replica:0/task:0/device:GPU:0 in order to run _EagerConst: Dst tensor is not initialized.
|
24
24
|
```
|
25
25
|
|
26
|
-
### 該当のソースコード
|
27
26
|
|
28
|
-
```python
|
29
|
-
import tensorflow as tf
|
30
|
-
from tensorflow.keras.layers import Conv2D, Activation, BatchNormalization, Dropout, Flatten, Dense
|
31
|
-
from tensorflow.keras import Model, layers
|
32
|
-
from keras.layers import Input
|
33
|
-
from keras.layers import Conv2D, MaxPooling2D, Input, Conv2DTranspose, concatenate, BatchNormalization, UpSampling2D
|
34
|
-
import matplotlib.pyplot as plt
|
35
|
-
import glob
|
36
|
-
import os
|
37
|
-
from PIL import Image,ImageOps
|
38
|
-
from keras.preprocessing import image
|
39
|
-
import numpy as np
|
40
|
-
import gc
|
41
|
-
import re
|
42
|
-
import pathlib
|
43
|
-
|
44
|
-
#データセットの読み込み
|
45
|
-
Combined_dir = "C:\\Users\\...\\source\\repos\\U-Net\\Combine_image"
|
46
|
-
Mask_dir = "C:\\Users\\...\\source\\repos\\U-Net\\Combine_GT"
|
47
|
-
|
48
|
-
def atoi(text):#名前順にソート
|
49
|
-
return int(text) if text.isdigit() else text
|
50
|
-
def natural_keys(text):
|
51
|
-
return [ atoi(c) for c in re.split(r'(\d+)', text) ]
|
52
|
-
|
53
|
-
images=[]
|
54
|
-
files = sorted(glob.glob(os.path.join(Combined_dir, '*.png')),key=natural_keys)
|
55
|
-
for file in files:
|
56
|
-
img = Image.open(file).convert('RGB')
|
57
|
-
img = np.asarray(img)
|
58
|
-
images.append(img)
|
59
|
-
Combine = np.asarray(images)
|
60
|
-
|
61
|
-
images=[]
|
62
|
-
files = sorted(glob.glob(os.path.join(Mask_dir, '*.png')),key=natural_keys)
|
63
|
-
for file in files:
|
64
|
-
img = Image.open(file).convert('L')
|
65
|
-
img = np.asarray(img)
|
66
|
-
images.append(img)
|
67
|
-
Mask = np.asarray(images)
|
68
|
-
|
69
|
-
#テストと訓練に分割
|
70
|
-
#Combine = Combine[..., tf.newaxis]
|
71
|
-
Mask = Mask[..., tf.newaxis]#tf.newaxisは説明変数(次元を追加するもの)
|
72
|
-
|
73
|
-
train_Combine0 = Combine[0:16000]#訓練データ(0:16000)
|
74
|
-
train_Mask0 = Mask[0:16000]
|
75
|
-
|
76
|
-
test_Combine = Combine[16000:18900]#訓練データ(16000):合計(18900)
|
77
|
-
test_Mask = Mask[16000:18900]
|
78
|
-
|
79
|
-
train_ds0 = np.asarray(train_Combine0)#配列を生成
|
80
|
-
train_gt0 = np.asarray(train_Mask0)
|
81
|
-
test_ds = np.asarray(test_Combine)
|
82
|
-
test_gt = np.asarray(test_Mask)
|
83
|
-
|
84
|
-
train_ds0 = train_ds0.astype('float32')/255.0#計算の都合上、入力を 0〜1の範囲の数値にした方が良い→データ型をfloatに変換したのち、255で割る
|
85
|
-
train_gt0 = train_gt0.astype('float32')/255.0
|
86
|
-
test_ds = test_ds.astype('float32')/255.0
|
87
|
-
test_gt = test_gt.astype('float32')/255.0
|
88
|
-
|
89
|
-
|
90
|
-
#パラメータ(エポックとバッチ)
|
91
|
-
epochs =70#epochsはデータを何周するか
|
92
|
-
epoch_num=list(range(1,epochs+1))
|
93
|
-
train_loss_num=[]
|
94
|
-
test_loss_num=[]
|
95
|
-
|
96
|
-
train_ds = tf.data.Dataset.from_tensor_slices((train_ds0,train_gt0)).batch(60)#tf.data.Dataset.from_tensor_slices().batch()を用いてバッチ化(一定量のデータを集め、一括処理する)
|
97
|
-
test_ds = tf.data.Dataset.from_tensor_slices((test_ds,test_gt)).batch(30)
|
98
|
-
print(train_ds)
|
99
|
-
print(test_ds)
|
100
|
-
|
101
|
-
|
102
|
-
|
103
|
-
|
104
|
-
|
105
|
-
#U-Netの実装
|
106
|
-
def unet(size=(128,128,3)):#画像サイズ
|
107
|
-
x=Input(size)
|
108
|
-
inputs=x
|
109
|
-
|
110
|
-
#エンコーダ
|
111
|
-
conv1=Conv2D(64, (3, 3) , activation = 'relu', padding = 'same')(x)#3*3convolution(畳み込み)、フィルタ(カーネル)64、padding(サイズ保持)
|
112
|
-
conv1=Conv2D(64, (3, 3) , activation = 'relu', padding = 'same')(conv1)#二回反復
|
113
|
-
down1=MaxPooling2D(pool_size=(2, 2), strides=None)(conv1)#サイズ2*2の最大プーリング層(入力画像内の2*2の領域で最大の数値を出力)
|
114
|
-
|
115
|
-
conv2=Conv2D(128, (3, 3) , activation = 'relu', padding = 'same')(down1)#(活性化関数)ReLU関数は入力値が負であれば0、正であれば1
|
116
|
-
conv2=Conv2D(128, (3, 3) , activation = 'relu', padding = 'same')(conv2)
|
117
|
-
down2=MaxPooling2D((2, 2), strides=2)(conv2)#2*2 max pooling と stride(各次元方向に1つ隣の要素に移動するために必要なバイト数)2
|
118
|
-
#以下省略
|
119
|
-
```
|
120
27
|
|
121
28
|
### 試したこと
|
122
29
|
|
2
情報の追加
test
CHANGED
File without changes
|
test
CHANGED
@@ -9,6 +9,9 @@
|
|
9
9
|
画像18900枚を使って、Unetのモデルを作っています。
|
10
10
|
エポック数やバッチサイズの変更で解決できるのでしょうか。
|
11
11
|
GPUなどの知識が乏しいため、どのように対処すれば良いのか全く分かりません。
|
12
|
+
|
13
|
+
専用GPUには余裕がありそうなのにエラーのままです
|
14
|
+
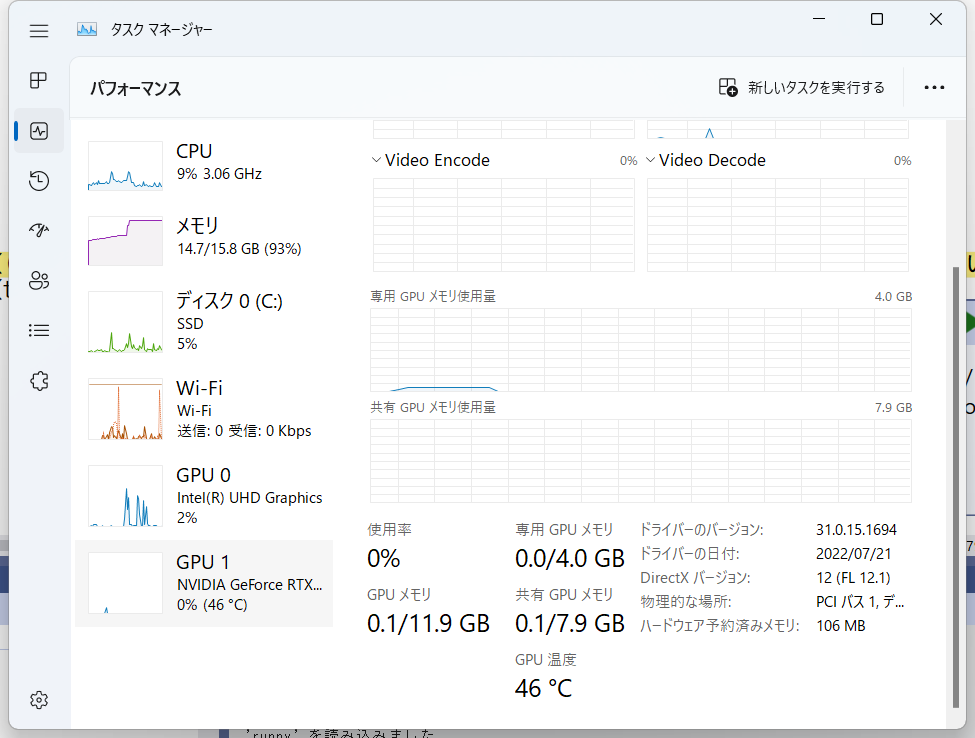
|
12
15
|
|
13
16
|
### 実現したいこと
|
14
17
|
|
1
タイトルの変更
test
CHANGED
@@ -1 +1 @@
|
|
1
|
-
GPU
|
1
|
+
GPUのエラー??を解決したい
|
test
CHANGED
File without changes
|