実装イメージ的には以下の様なことでしょうか。
もしイメージが違ったら回答スルーして頂いて大丈夫です。
実装イメージ
赤い立方体の周りを、青い球体が円形に移動しているイメージ。
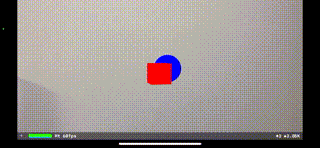
実装概要
ARKit SceneKit かわいいアニメーション・レシピ・ブック
↑こちらの参考記事内にある、「オブジェクトの中心の回転と、回転軸をずらす場合を同時に表示するとこういうイメージ」という実装方法を参考にすることで実現できそうですが、いかがでしょうか。
(中華料理屋とかにある、中華テーブルの回転をイメージすると想像付きやすいかもしれません)
今回実装した手順
こちらで実装した大まかな手順は以下です。
1、回転の軸となる空ノードを生成
2、回転移動している様に見せる球体ノードを生成(今回のイメージだと、青い球体)
3、球体ノードを、軸となる空ノードのChiledNodeに指定
※ChiledNodeに指定する際、回転移動用ノードの軸をずらす
4、空ノード自体を回転させると、球体ノードが円形に移動している様に見えるようになる
サンプルコード
アクションの処理自体は詳しくないので、追加質問あった際にはお答えできないです。すみません。
Swift
1import UIKit
2import SceneKit
3import ARKit
4
5class ViewController: UIViewController, ARSCNViewDelegate {
6
7 @IBOutlet var sceneView: ARSCNView!
8
9 override func viewDidLoad() {
10 super.viewDidLoad()
11
12 sceneView.delegate = self
13 sceneView.showsStatistics = true
14 sceneView.scene = SCNScene();
15
16 // 回転の中心に表示する、赤い立方体ノード生成
17 let box = SCNBox(width: 0.01, height: 0.01, length: 0.01, chamferRadius: 0)
18 let boxNode = SCNNode(geometry: box)
19 boxNode.name = "cube"
20 boxNode.geometry?.firstMaterial?.diffuse.contents = UIColor.red
21 boxNode.position = SCNVector3(0, 0, 0)
22 sceneView.scene.rootNode.addChildNode(boxNode)
23
24 // 回転の軸となる、空のノード生成
25 let emptyNode = SCNNode()
26 sceneView.scene.rootNode.addChildNode(emptyNode)
27
28 // 赤い球体ノード生成
29 let sphere = SCNSphere(radius: 0.01)
30 let sphereNode = SCNNode(geometry: sphere)
31 sphereNode.name = "sphere"
32 sphereNode.geometry?.firstMaterial?.diffuse.contents = UIColor.blue
33
34 // 空のノードに、赤い球体ノード追加
35 emptyNode.addChildNode(sphereNode)
36 emptyNode.position = SCNVector3(0, 0, 0)
37 sphereNode.position = SCNVector3(0.05, 0, 0)
38
39 // 空のノードを回転させることで、赤い球体が円形に回転してる様に見せる
40 emptyNode.runAction(SCNAction.repeatForever(SCNAction.rotateBy(x: 0, y: 1, z: 0, duration: 0.5)))
41 }
42
43 override func viewWillAppear(_ animated: Bool) {
44 super.viewWillAppear(animated)
45
46 let configuration = ARWorldTrackingConfiguration()
47 sceneView.session.run(configuration)
48 }
49
50 override func viewWillDisappear(_ animated: Bool) {
51 super.viewWillDisappear(animated)
52
53 sceneView.session.pause()
54 }
55
56
57 func session(_ session: ARSession, didFailWithError error: Error) {
58 // Present an error message to the user
59
60 }
61
62 func sessionWasInterrupted(_ session: ARSession) {
63 // Inform the user that the session has been interrupted, for example, by presenting an overlay
64
65 }
66
67 func sessionInterruptionEnded(_ session: ARSession) {
68 // Reset tracking and/or remove existing anchors if consistent tracking is required
69
70 }
71}
バッドをするには、ログインかつ
こちらの条件を満たす必要があります。
2021/05/03 09:21