やり方
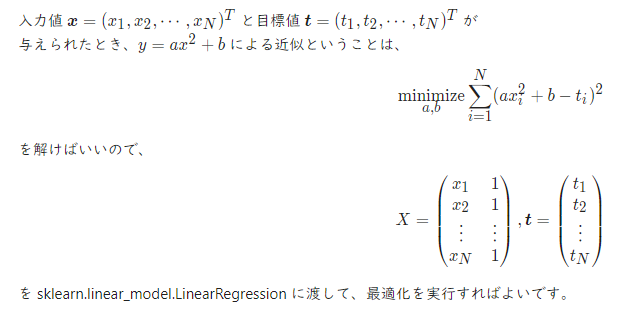
最小二乗法は sklearn.linear_model.LinearRegression または numpy.linalg.lstsq を使う。
参考
サンプルコード
python
1import matplotlib.pyplot as plt
2import numpy as np
3from sklearn.linear_model import LinearRegression
4
5np.random.seed(0)
6
7a, b = 1.2, 2 # 真のパラメータ
8
9
10def make_dataset(n, noise=False):
11 x = np.linspace(-3, 3, n)
12 y = a * x ** 2 + b
13 if noise:
14 y += np.random.normal(0, 0.5, n)
15
16 return x, y
17
18
19# 訓練集合を作成する。
20x_train, y_train = make_dataset(10, noise=True)
21x, y = make_dataset(100)
22
23
24def to_polynominal_feature(x):
25 return np.column_stack([x ** 2, x ** 0]) # [x^2, 1]
26
27
28X_train = to_polynominal_feature(x_train)
29X = to_polynominal_feature(x)
30
31model = LinearRegression(fit_intercept=False).fit(X_train, y_train)
32
33# 最小二乗法の解
34a_sol, b_sol = model.coef_
35
36# 近似した曲線
37y_pred = model.predict(X)
38
39# 描画する。
40fig, ax = plt.subplots()
41
42ax.scatter(x_train, y_train, s=50, fc="none", ec="b", label="train")
43ax.plot(x, y_pred, "r", label=fr"${a_sol:.2f} x^2 + {b_sol:.2f}$")
44ax.plot(x, y, "g", label=fr"${a:.2f} x^2 + {b:.2f}$")
45ax.legend()
46ax.grid()
47
48plt.show()
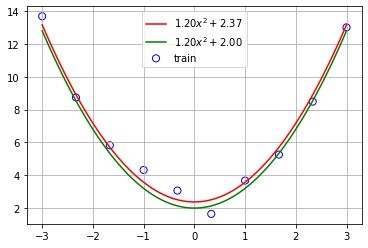
バッドをするには、ログインかつ
こちらの条件を満たす必要があります。