QGraphcisScene に描画する場合、描画するオブジェクトを QGraphicsItem として作成する必要があります。
手順
- QGraphicsItem を継承したクラスを作成する。
- paint(self, painter, option, widget) をオーバーライドする。
- paint() 関数に渡ってくる painter オブジェクトで描画する。
サンプルコード
python
1import sys
2
3import numpy as np
4from PyQt5.QtWidgets import *
5from PyQt5.QtGui import *
6from PyQt5.QtCore import *
7
8
9class StarGraphicsItem(QGraphicsItem):
10 def __init__(self, center, radius, parent=None):
11 super().__init__(parent)
12 self.center = center
13 self.radius = radius
14
15 def paint(self, painter, option, widget):
16 painter.setRenderHint(QPainter.Antialiasing)
17 # ペンとブラシを設定する。
18 painter.setPen(QPen(Qt.black, 3))
19 painter.setBrush(Qt.yellow)
20
21 # ポリゴンを描画する。
22 poly = self.createShape()
23 painter.drawPolygon(poly)
24
25 def createShape(self):
26 '''星を表すポリゴンを生成する。
27 '''
28 # 点を生成する。
29 num_points = 11
30 r = self.radius * np.where(np.arange(num_points) % 2 == 0, 0.5, 1.) # 半径
31 theta = np.linspace(0, 2 * np.pi, num_points) # 角度
32 xs = self.center[0] + r * np.sin(theta) # x 座標
33 ys = self.center[1] + r * np.cos(theta) # y 座標
34
35 # QPolygonF に格納する。
36 polygon = QPolygonF()
37 [polygon.append(QPointF(x, y)) for x, y in zip(xs, ys)]
38
39 return polygon
40
41 def boundingRect(self):
42 '''この図形を囲む矩形を返す。
43 '''
44
45 return QRectF(self.center[0] - self.radius,
46 self.center[1] - self.radius,
47 self.radius * 2, self.radius * 2)
48
49
50class MainWindow(QMainWindow):
51 def __init__(self, parent=None):
52 super().__init__(parent)
53 self.setGeometry(100, 100, 500, 500)
54
55 # シーンを作成する。
56 starItem = StarGraphicsItem(center=(100, 100), radius=100)
57 self.scene = QGraphicsScene()
58 self.scene.addItem(starItem)
59
60 # QGraphicsView
61 graphicView = QGraphicsView()
62 graphicView.setScene(self.scene)
63
64 # Central Widget
65 central_widget = QWidget()
66 self.setCentralWidget(central_widget)
67
68 # Layout
69 layout = QVBoxLayout()
70 layout.addWidget(graphicView)
71 central_widget.setLayout(layout)
72
73
74if __name__ == '__main__':
75 app = QApplication(sys.argv)
76 main_window = MainWindow()
77
78 main_window.show()
79 sys.exit(app.exec_())
80
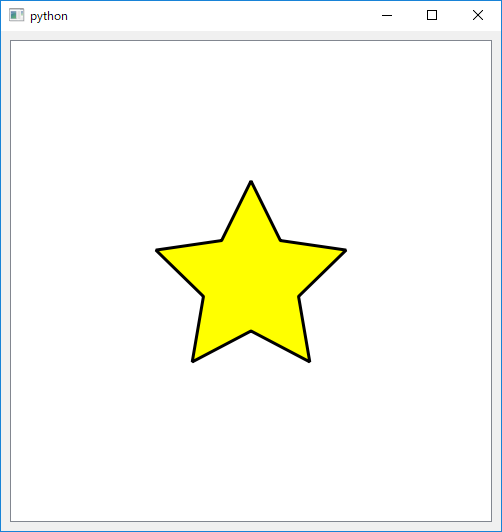