Template
をC#コードで作るなら、FrameworkElementFactory
で組み立てる必要があります(他にも文字列からロードするとかリソースから取ってくる等あります)
FrameworkElementFactory - Google 検索
xml
1<Window
2 x:Class="Qca70slvq1tnn38.MainWindow"
3 xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
4 xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
5 Width="800"
6 Height="450">
7 <Grid>
8 <TabControl x:Name="tabControl" FontSize="24">
9 <TabItem Header="1">
10 <!--<Grid Width="1920" Height="1080">-->
11 <Grid>
12 <ListBox>
13 <ListBox.ItemsPanel>
14 <ItemsPanelTemplate>
15 <UniformGrid Columns="2" />
16 </ItemsPanelTemplate>
17 </ListBox.ItemsPanel>
18 <ListBoxItem>Content0</ListBoxItem>
19 <ListBoxItem>Content1</ListBoxItem>
20 <ListBoxItem>Content2</ListBoxItem>
21 </ListBox>
22 </Grid>
23 </TabItem>
24 </TabControl>
25 </Grid>
26</Window>
cs
1using System.Windows;
2using System.Windows.Controls;
3using System.Windows.Controls.Primitives;
4
5namespace Qca70slvq1tnn38;
6
7
8public partial class MainWindow : Window
9{
10 public MainWindow()
11 {
12 InitializeComponent();
13
14
15 var template = new ItemsPanelTemplate();
16 var factory = new FrameworkElementFactory(typeof(UniformGrid));
17 factory.SetValue(UniformGrid.ColumnsProperty, 2);
18 template.VisualTree = factory;
19
20 var list = new ListBox() { ItemsPanel = template, };
21 for (var i = 0; i < 3; i++)
22 {
23 list.Items.Add(new ListViewItem() { Content = $"Content{i}", });
24 }
25
26 var grid = new Grid();// { Width = 1920, Height = 1080, };
27 grid.Children.Add(list);
28 tabControl.Items.Add(new TabItem { Header = "2", Content = grid, });
29 }
30}
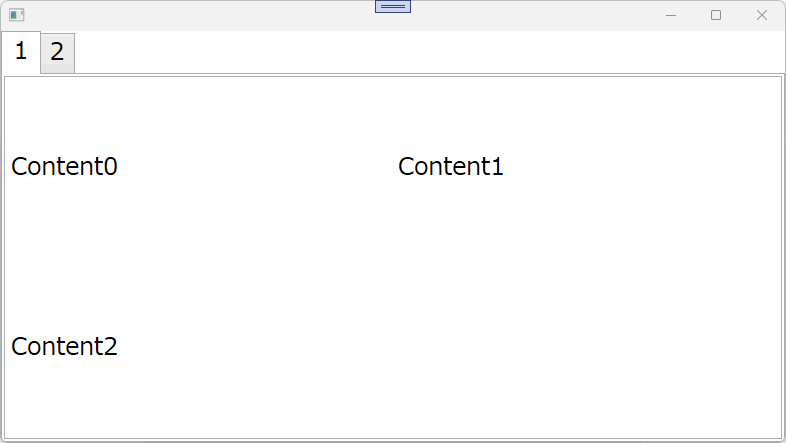
ですがめんどくさいし嬉しくもないので、もっとWPFっぽい手法に変更できないでしょうか?
xml
1<Window
2 x:Class="Qca70slvq1tnn38.MainWindow"
3 xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
4 xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
5 Width="800"
6 Height="450">
7 <DockPanel>
8 <StackPanel DockPanel.Dock="Top" Orientation="Horizontal">
9 <Button Command="{Binding AddTabCommand}" Content="Add Tab" />
10 <Button Command="{Binding Tabs/AddContentCommand}" Content="Add Content" />
11 </StackPanel>
12
13 <TabControl
14 DisplayMemberPath="Header"
15 FontSize="24"
16 IsSynchronizedWithCurrentItem="True"
17 ItemsSource="{Binding Tabs}">
18
19 <TabControl.ContentTemplate>
20 <DataTemplate>
21 <Grid>
22 <ListBox ItemsSource="{Binding Contents}">
23 <ListBox.ItemsPanel>
24 <ItemsPanelTemplate>
25 <UniformGrid Columns="2" />
26 </ItemsPanelTemplate>
27 </ListBox.ItemsPanel>
28 </ListBox>
29 </Grid>
30 </DataTemplate>
31 </TabControl.ContentTemplate>
32 </TabControl>
33 </DockPanel>
34</Window>
cs
1using System.Collections.ObjectModel;
2using System.Windows;
3using CommunityToolkit.Mvvm.Input;
4
5namespace Qca70slvq1tnn38;
6
7
8public partial class ViewModel
9{
10 public ObservableCollection<TabViewModel> Tabs { get; }
11
12 public ViewModel()
13 {
14 // なんか読み込んだりしたテイ
15 Tabs = new()
16 {
17 new()
18 {
19 Header = "1",
20 Contents = new(new string[] {"Content0", "Content1", "Content2", }),
21 },
22 new()
23 {
24 Header = "2",
25 Contents = new(new string[] {"Content0", "Content1", "Content2", }),
26 },
27 };
28 }
29
30 // 動的に追加したり
31 [RelayCommand] private void AddTab() => Tabs.Add(new() { Header = $"{Tabs.Count + 1}", Contents = new(), });
32}
33
34public partial class TabViewModel
35{
36 public required string Header { get; init; }
37 public required ObservableCollection<string> Contents { get; init; }
38
39 [RelayCommand] private void AddContent() => Contents.Add($"Content{Contents.Count}");
40}
41
42public partial class MainWindow : Window
43{
44 public MainWindow()
45 {
46 InitializeComponent();
47 DataContext = new ViewModel();
48 }
49}
NuGet Gallery | CommunityToolkit.Mvvm 8.2.2
RelayCommand 属性 - .NET Community Toolkit | Microsoft Learn
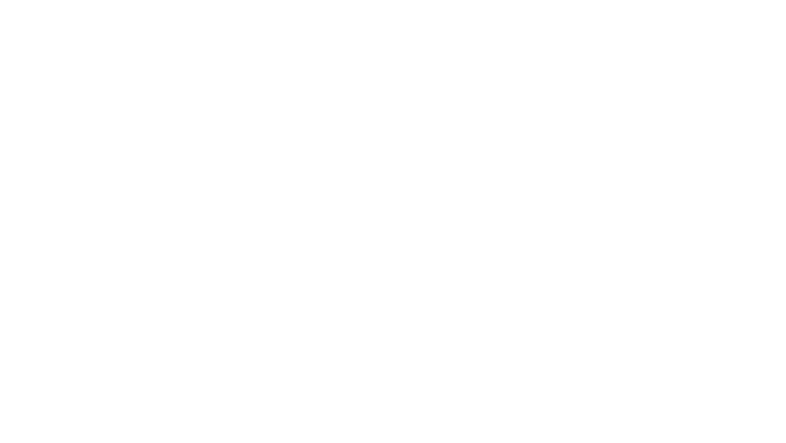
バッドをするには、ログインかつ
こちらの条件を満たす必要があります。
2023/10/31 00:47