リファレンスを検索してみたところ、ILogHandlerのページに独自のログハンドラーを作って特殊な動作をさせる例が載っていました。
これを利用して追加メッセージを出せないかと思い、例をまねて下記のようなスクリプトを作ってプロジェクト内の適当な場所に入れておき...
C#
1using System;
2using System.Collections.Generic;
3using UnityEngine;
4using Object = UnityEngine.Object;
5
6public class CustomLogHandler : ILogHandler
7{
8 // ここに捕捉したい例外と表示したい文章を登録しておく
9 private static readonly Dictionary<Type, string> Remarks = new Dictionary<Type, string>
10 {
11 {typeof(NullReferenceException), "セットされていないオブジェクトがあります"},
12 {typeof(IndexOutOfRangeException), "インデックスが配列の境界外です"}
13 };
14
15 // デフォルトのログハンドラーをとっておく
16 private readonly ILogHandler defaultLogHandler = Debug.unityLogger.logHandler;
17
18 // ゲームのロード時にログハンドラーをこのカスタムログハンドラーに差し替える
19 [RuntimeInitializeOnLoadMethod]
20 private static void OnLoad()
21 {
22 Debug.unityLogger.logHandler = new CustomLogHandler();
23 }
24
25 // LogFormatはデフォルトのログハンドラーでそのまま出力する
26 public void LogFormat(LogType logType, Object context, string format, params object[] args)
27 {
28 this.defaultLogHandler.LogFormat(logType, context, format, args);
29 }
30
31 // LogExceptionはデフォルトのログハンドラーでそのまま出力したあと、
32 // もし例外に対応する文章があればLogFormatでそれを出力する
33 public void LogException(Exception exception, Object context)
34 {
35 this.defaultLogHandler.LogException(exception, context);
36 if (Remarks.TryGetValue(exception.GetType(), out var remark))
37 {
38 this.defaultLogHandler.LogFormat(LogType.Exception, context, remark);
39 }
40 }
41}
下記のようなスクリプトで例外を発生させたところ...
C#
1using UnityEngine;
2
3public class DummyScript : MonoBehaviour
4{
5 private void Start()
6 {
7 ((Transform)null).position = Vector3.one;
8 }
9}
下図のように、例外メッセージに続いて追加のメッセージが出力されました。
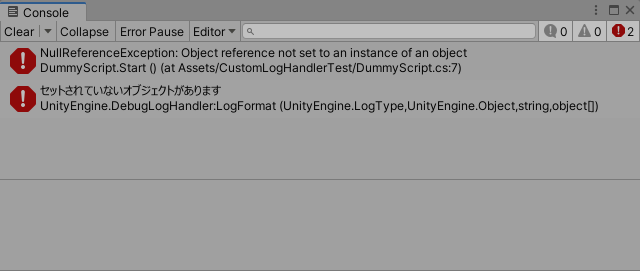