簡単な方法: 対象を一つづつ処理するのであれば、
sg.one_line_progress_meterを使うと楽できます。
python
1
2with open(filepath) as infile:
3 for line in infile:
4 if not sg.one_line_progress_meter(...):
5 break # 中断
6 # ファイル処理
ここから、ステップアップとして
- 進捗ダイアログ以外のUI操作も可能にする -> timeout イベントを使う
- 複数のファイルを同時に処理する -> スレッドを使う
等が考えられます。
以下は timeout で処理するデモ(スレッドは使ってません)
工夫が必要な点:
- ファイルの処理を「ジェネレータ」として実装
timeout イベント内で next() で読み出すことで、
1ステップづつ実行が実現できます
- window.read の timeout パラメータは、必要なときのみ設定
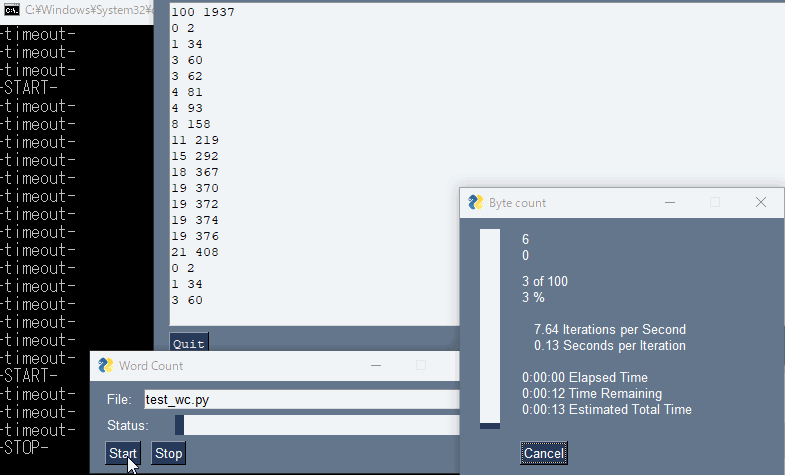
python
1
2from contextlib import closing
3import PySimpleGUI as sg
4
5layout = [
6 [sg.Text("File:"), sg.Input(__file__, key="-INPUT-FILE-")],
7 [sg.Text("Status:"),
8 sg.ProgressBar(key="-PROCESSING-", size=(30, 20), max_value=100)],
9 [sg.Button("Start", key="-START-"), sg.Button("Stop", key="-STOP-")],
10]
11
12
13
14def gen_bytes_count(filepath):
15 import os
16 # Total Bytes
17 filesize = os.stat(filepath).st_size
18 count = 0
19
20 # NOTE: バイト数カウントのためバイナリで開いてます
21 # テキストで開いた場合、文字数 != バイト数(ファイルサイズ) と一致しません
22 with open(filepath, "rb") as infile:
23 for line in infile:
24 count += len(line)
25 yield (int(100*count/filesize), count)
26
27task = None
28options = {}
29timeout_options = {"timeout":100, "timeout_key":"-timeout-"}
30
31def task_cancel():
32 global task
33 task = None
34 options.clear()
35
36def task_start(filepath):
37 global task
38 task = gen_bytes_count(filepath)
39 options.update(timeout_options)
40
41
42with closing(sg.Window('Bytes Count', layout)) as window:
43
44 while True:
45 event, values = window.read(**options)
46 # print(event)
47 if not event:
48 break
49 elif event == "-STOP-":
50 task_cancel()
51 elif event == "-START-":
52 task_start(values["-INPUT-FILE-"])
53 elif event == "-timeout-":
54 if not task: # 処理するファイルが無い時
55 continue
56
57 info = next(task, None)
58 if not info: # ファイル処理が終わった時
59 task_cancel()
60 continue
61
62 status, count = info
63 window["-PROCESSING-"].update_bar(status)
64 sg.Print(status, count)
65 ret = sg.OneLineProgressMeter(
66 'Byte count', status, 100, "", '{}'.format(count))
67 if not ret: # 進捗ダイアログの Cancel が押された時
68 task_cancel()
69 continue
70
71
バッドをするには、ログインかつ
こちらの条件を満たす必要があります。
2020/05/29 00:48