Main.xaml内で読み込んでいるChildArea.xamlのViewModel内のLoadedコマンド
パラメータにDataContext(MainViewModel)を設定したいです。
実際にやりたいことはパラメータに渡したいのではなく、MainViewModel
のインスタンスが欲しいだけですよね?
単にこれでいいのではないでしょうか。
cs
1public class ChildAreaViewModel : BindableBase
2{
3 private MainViewModel _mainViewModel;
4
5 public ChildAreaViewModel(MainViewModel mainViewModel)
6 {
7 _mainViewModel = mainViewModel;
8 }
コードビハインドでDataContextを設定するようにしたり
Main.xaml内で、DataContextを明示して設定するようにしてみましたが
そうすると、ChildViewModelのLoadedコマンドが呼ばれなくなりました…。
DataContext
はひとつしか持てないので、AutoWireViewModel
しているViewに違うDataContext
を設定したら当然動かなくなりますよね。
MainViewModel
をRegisterSingleton
すれば上記で十分です。
シングルトンにしたくない場合は、TimerTrigger
に変更したら取れました(ぶっちゃけクソっぽいが^^;
xml
1 <i:TimerTrigger EventName="Loaded" MillisecondsPerTick="100" TotalTicks="1">
2 <i:InvokeCommandAction Command="{Binding LoadedCommand}" CommandParameter="{Binding DataContext, ElementName=MainView}" />
3 </i:TimerTrigger>
100の根拠はありません(手元では1でも0でも!! 取れました)
TimerTrigger クラス (Microsoft.Expression.Interactivity.Core) | Microsoft Learn
xml:MainWindow.xaml
1<Window
2 x:Class="Q07l0vpm2ryc05t.Views.MainWindow"
3 xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
4 xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
5 xmlns:prism="http://prismlibrary.com/"
6 Width="525"
7 Height="350"
8 prism:ViewModelLocator.AutoWireViewModel="True">
9 <DockPanel>
10 <StackPanel DockPanel.Dock="Top" Orientation="Horizontal">
11 <Button Command="{Binding NavigateCommand}" CommandParameter="ViewA">Navigate ViewA</Button>
12 <Button Command="{Binding NavigateCommand}" CommandParameter="Main">Navigate Main</Button>
13 <Button Command="{Binding ShowDialogCommand}">Show MainDialog</Button>
14 </StackPanel>
15 <ContentControl prism:RegionManager.RegionName="ContentRegion" />
16 </DockPanel>
17</Window>
cs:MainWindowViewModel.cs
1using Prism.Mvvm;
2using Prism.Regions;
3using Prism.Services.Dialogs;
4using Q07l0vpm2ryc05t.Views;
5using Reactive.Bindings;
6
7namespace Q07l0vpm2ryc05t.ViewModels;
8
9public class MainWindowViewModel : BindableBase
10{
11 public ReactiveCommand<string> NavigateCommand { get; }
12 public ReactiveCommand ShowDialogCommand { get; }
13
14 public MainWindowViewModel(IRegionManager regionManager, IDialogService dialogService)
15 {
16 NavigateCommand = new ReactiveCommand<string>()
17 .WithSubscribe(x => regionManager.RequestNavigate("ContentRegion", x));
18
19 ShowDialogCommand = new ReactiveCommand()
20 .WithSubscribe(() => dialogService.ShowDialog(nameof(Main)));
21 }
22}
xml:Main.xaml
1<UserControl
2 x:Class="Q07l0vpm2ryc05t.Views.Main"
3 xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
4 xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
5 xmlns:TestArea="clr-namespace:Q07l0vpm2ryc05t.Views"
6 xmlns:prism="http://prismlibrary.com/"
7 x:Name="MainView"
8 prism:ViewModelLocator.AutoWireViewModel="True">
9 <StackPanel>
10 <TextBlock Text="Main" />
11 <TextBlock Text="{Binding HashCode}" />
12 <GroupBox Margin="5">
13 <TestArea:ChildArea />
14 </GroupBox>
15 </StackPanel>
16</UserControl>
cs:MainViewModel.cs
1using System;
2using Prism.Mvvm;
3using Prism.Services.Dialogs;
4
5namespace Q07l0vpm2ryc05t.ViewModels;
6
7public class MainViewModel : BindableBase, IDialogAware
8{
9 public string HashCode => $"MainViewModel.HashCode:{GetHashCode()}";
10
11 public string Title => "MainDialog";
12 public event Action<IDialogResult> RequestClose;
13
14 public MainViewModel() { }
15
16 public bool CanCloseDialog() => true;
17 public void OnDialogClosed() { }
18 public void OnDialogOpened(IDialogParameters parameters) { }
19}
xml:ChildArea.xaml
1<UserControl
2 x:Class="Q07l0vpm2ryc05t.Views.ChildArea"
3 xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
4 xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
5 xmlns:i="http://schemas.microsoft.com/xaml/behaviors"
6 xmlns:prism="http://prismlibrary.com/"
7 x:Name="ChildAreaView"
8 prism:ViewModelLocator.AutoWireViewModel="True">
9 <i:Interaction.Triggers>
10
11 <!--<i:EventTrigger EventName="Loaded">
12 <i:InvokeCommandAction Command="{Binding LoadedCommand}" CommandParameter="{Binding DataContext, ElementName=MainView}" />
13 </i:EventTrigger>-->
14
15 <i:TimerTrigger
16 EventName="Loaded"
17 MillisecondsPerTick="0"
18 TotalTicks="1">
19 <i:InvokeCommandAction Command="{Binding LoadedCommand}" CommandParameter="{Binding DataContext, ElementName=MainView}" />
20 </i:TimerTrigger>
21
22 </i:Interaction.Triggers>
23
24 <StackPanel>
25 <TextBlock Text="ChildArea" />
26 <TextBlock Text="{Binding HashCode}" />
27 </StackPanel>
28</UserControl>
cs:ChildAreaViewModel.cs
1using Prism.Mvvm;
2using Reactive.Bindings;
3
4namespace Q07l0vpm2ryc05t.ViewModels;
5
6public class ChildAreaViewModel : BindableBase
7{
8 public string HashCode => $"MainViewModel.HashCode:{_mainViewModel?.GetHashCode()}";
9 public ReactiveCommand<object> LoadedCommand { get; }
10
11 private MainViewModel _mainViewModel;
12
13 public ChildAreaViewModel(MainViewModel mainViewModel)
14 {
15 // RegisterSingletonでいいならこれだけ
16 //_mainViewModel = mainViewModel;
17
18 LoadedCommand = new ReactiveCommand<object>().WithSubscribe(Loaded);
19 }
20
21 private void Loaded(object mainViewModel)
22 {
23 _mainViewModel = mainViewModel as MainViewModel;
24 RaisePropertyChanged(nameof(HashCode));
25 }
26}
cs:App.xaml.cs
1using System.Windows;
2using Prism.Ioc;
3using Q07l0vpm2ryc05t.ViewModels;
4using Q07l0vpm2ryc05t.Views;
5
6namespace Q07l0vpm2ryc05t;
7
8public partial class App
9{
10 protected override Window CreateShell() => Container.Resolve<MainWindow>();
11
12 protected override void RegisterTypes(IContainerRegistry containerRegistry)
13 {
14 //containerRegistry.RegisterSingleton<MainViewModel>();
15
16 containerRegistry.RegisterForNavigation<ViewA>();
17 containerRegistry.RegisterForNavigation<Main>();
18
19 containerRegistry.RegisterDialog<Main>();
20 }
21}
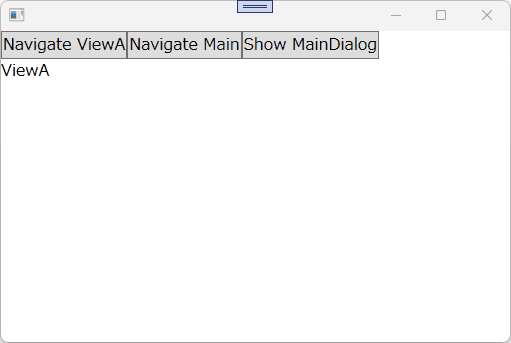
バッドをするには、ログインかつ
こちらの条件を満たす必要があります。
2024/07/21 10:30
2024/07/21 10:42
2024/07/21 11:38
2024/07/21 11:44
2024/07/21 13:06
2024/07/21 13:46
2024/07/21 14:17
2024/07/21 15:19
2024/07/21 15:23
2024/07/22 09:44
2024/08/05 07:24
2024/08/30 12:22