Jetpack Compose を使っていないのでイマイチピンと来ませんが、試しに作ってみたものは動作しています。
kotlin
1import android.os.Bundle
2import android.util.Log
3import androidx.activity.ComponentActivity
4import androidx.activity.compose.setContent
5import androidx.compose.foundation.layout.*
6import androidx.compose.foundation.lazy.*
7import androidx.compose.material3.*
8import androidx.compose.runtime.*
9import androidx.compose.ui.Modifier
10import androidx.compose.ui.graphics.Color
11import androidx.compose.ui.text.TextStyle
12import androidx.compose.ui.text.font.FontWeight
13import androidx.compose.ui.unit.*
14import androidx.lifecycle.viewmodel.compose.viewModel
15import java.time.LocalTime
16import java.util.UUID
17
18class MainActivity : ComponentActivity() {
19 override fun onCreate(savedInstanceState: Bundle?) {
20 super.onCreate(savedInstanceState)
21 setContent {
22 Column {
23 AlarmSetScreen()
24 HomeScreen()
25 }
26 }
27 }
28}
29
30@Composable
31fun AlarmSetScreen(
32 viewModel: AlarmViewModel = viewModel()
33) {
34 Button(
35 onClick = {
36 val newCard = CardData(
37 id = UUID.randomUUID().toString(),
38 isParent = true,
39 childId = null,
40 alarmTime = LocalTime.now(),//time,
41 switchValue = false
42 )
43 viewModel.addCard(newCard)
44// navController.navigate("homeScreen")
45 },
46 modifier = Modifier
47 .padding(16.dp)
48 .height(60.dp)
49 .fillMaxWidth(),
50 colors = ButtonDefaults.buttonColors(containerColor = Color.Cyan),
51 ) {
52 Text(
53 "セットする",
54 style = TextStyle(
55 fontSize = 20.sp,
56 fontWeight = FontWeight.Bold
57 )
58 )
59 }
60}
61
62@Composable
63fun HomeScreen(
64 alarmViewModel: AlarmViewModel = viewModel()
65) {
66 val cards = alarmViewModel.cards.collectAsState()
67 Log.d("", "受け取れてるかカード情報$cards")
68
69 LazyColumn(
70 modifier = Modifier
71 //.background(color = Color.Black)
72 .fillMaxSize()
73 ) {
74 items(cards.value) { card ->
75 Log.d("", "きているのか$cards")
76 AlarmCard(cardData = card)
77 //AlarmCard(navController = navController, cardData = card, viewModel = alarmViewModel)
78 }
79 }
80}
81
82@Composable
83fun AlarmCard(
84 cardData: CardData
85) {
86 Text(
87 "id: ${cardData.id}",
88 style = TextStyle(
89 fontSize = 16.sp
90 )
91 )
92 Text(
93 "alarmTime: ${cardData.alarmTime}",
94 style = TextStyle(
95 fontSize = 16.sp
96 )
97 )
98}
kotlin
1import android.util.Log
2import androidx.lifecycle.*
3import kotlinx.coroutines.flow.*
4import kotlinx.coroutines.launch
5import java.time.LocalTime
6
7class AlarmViewModel : ViewModel() {
8 private val _cards = MutableStateFlow<List<CardData>>(emptyList())
9 val cards = _cards.asStateFlow()
10
11 companion object {
12 const val TAG = "AlarmViewModel"
13 }
14 fun addCard(card: CardData) {
15 viewModelScope.launch {
16 val newList = _cards.value.toMutableList()
17 newList.add(card)
18 _cards.value = newList
19 Log.d(TAG, "addCard: カード追加後のリストサイズ: ${_cards.value.size}")
20 Log.d(TAG, "addCard: カード追加: ${_cards.value}")
21 Log.d(TAG, "addCard: newListはこちら: ${newList}")
22 }
23 }
24}
25
26data class CardData(
27 val id: String,
28 val isParent: Boolean,
29 val childId: String?,
30 val alarmTime: LocalTime?,
31 val switchValue: Boolean
32)
3回「セットする」をクリックした所
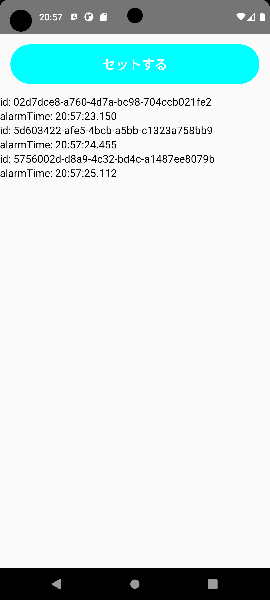
logcat
2024-02-20 20:57:23.152 30630-30630 AlarmViewModel com.teratail.q_minbpx5ux7ywho D addCard: カード追加後のリストサイズ: 1
2024-02-20 20:57:23.153 30630-30630 AlarmViewModel com.teratail.q_minbpx5ux7ywho D addCard: カード追加: [CardData(id=02d7dce8-a760-4d7a-bc98-704ccb021fe2, isParent=true, childId=null, alarmTime=20:57:23.150, switchValue=false)]
2024-02-20 20:57:23.153 30630-30630 AlarmViewModel com.teratail.q_minbpx5ux7ywho D addCard: newListはこちら: [CardData(id=02d7dce8-a760-4d7a-bc98-704ccb021fe2, isParent=true, childId=null, alarmTime=20:57:23.150, switchValue=false)]
2024-02-20 20:57:23.183 30630-30630 <no-tag> com.teratail.q_minbpx5ux7ywho D きているのかMutableState(value=[CardData(id=02d7dce8-a760-4d7a-bc98-704ccb021fe2, isParent=true, childId=null, alarmTime=20:57:23.150, switchValue=false)])@17520182
2024-02-20 20:57:24.456 30630-30630 AlarmViewModel com.teratail.q_minbpx5ux7ywho D addCard: カード追加後のリストサイズ: 2
2024-02-20 20:57:24.456 30630-30630 AlarmViewModel com.teratail.q_minbpx5ux7ywho D addCard: カード追加: [CardData(id=02d7dce8-a760-4d7a-bc98-704ccb021fe2, isParent=true, childId=null, alarmTime=20:57:23.150, switchValue=false), CardData(id=5d603422-afe5-4bcb-a5bb-c1323a758bb9, isParent=true, childId=null, alarmTime=20:57:24.455, switchValue=false)]
2024-02-20 20:57:24.456 30630-30630 AlarmViewModel com.teratail.q_minbpx5ux7ywho D addCard: newListはこちら: [CardData(id=02d7dce8-a760-4d7a-bc98-704ccb021fe2, isParent=true, childId=null, alarmTime=20:57:23.150, switchValue=false), CardData(id=5d603422-afe5-4bcb-a5bb-c1323a758bb9, isParent=true, childId=null, alarmTime=20:57:24.455, switchValue=false)]
2024-02-20 20:57:24.469 30630-30630 <no-tag> com.teratail.q_minbpx5ux7ywho D きているのかMutableState(value=[CardData(id=02d7dce8-a760-4d7a-bc98-704ccb021fe2, isParent=true, childId=null, alarmTime=20:57:23.150, switchValue=false), CardData(id=5d603422-afe5-4bcb-a5bb-c1323a758bb9, isParent=true, childId=null, alarmTime=20:57:24.455, switchValue=false)])@17520182
2024-02-20 20:57:24.481 30630-30630 <no-tag> com.teratail.q_minbpx5ux7ywho D きているのかMutableState(value=[CardData(id=02d7dce8-a760-4d7a-bc98-704ccb021fe2, isParent=true, childId=null, alarmTime=20:57:23.150, switchValue=false), CardData(id=5d603422-afe5-4bcb-a5bb-c1323a758bb9, isParent=true, childId=null, alarmTime=20:57:24.455, switchValue=false)])@17520182
2024-02-20 20:57:25.112 30630-30630 AlarmViewModel com.teratail.q_minbpx5ux7ywho D addCard: カード追加後のリストサイズ: 3
2024-02-20 20:57:25.113 30630-30630 AlarmViewModel com.teratail.q_minbpx5ux7ywho D addCard: カード追加: [CardData(id=02d7dce8-a760-4d7a-bc98-704ccb021fe2, isParent=true, childId=null, alarmTime=20:57:23.150, switchValue=false), CardData(id=5d603422-afe5-4bcb-a5bb-c1323a758bb9, isParent=true, childId=null, alarmTime=20:57:24.455, switchValue=false), CardData(id=5756002d-d8a9-4c32-bd4c-a1487ee8079b, isParent=true, childId=null, alarmTime=20:57:25.112, switchValue=false)]
2024-02-20 20:57:25.113 30630-30630 AlarmViewModel com.teratail.q_minbpx5ux7ywho D addCard: newListはこちら: [CardData(id=02d7dce8-a760-4d7a-bc98-704ccb021fe2, isParent=true, childId=null, alarmTime=20:57:23.150, switchValue=false), CardData(id=5d603422-afe5-4bcb-a5bb-c1323a758bb9, isParent=true, childId=null, alarmTime=20:57:24.455, switchValue=false), CardData(id=5756002d-d8a9-4c32-bd4c-a1487ee8079b, isParent=true, childId=null, alarmTime=20:57:25.112, switchValue=false)]
2024-02-20 20:57:25.133 30630-30630 <no-tag> com.teratail.q_minbpx5ux7ywho D きているのかMutableState(value=[CardData(id=02d7dce8-a760-4d7a-bc98-704ccb021fe2, isParent=true, childId=null, alarmTime=20:57:23.150, switchValue=false), CardData(id=5d603422-afe5-4bcb-a5bb-c1323a758bb9, isParent=true, childId=null, alarmTime=20:57:24.455, switchValue=false), CardData(id=5756002d-d8a9-4c32-bd4c-a1487ee8079b, isParent=true, childId=null, alarmTime=20:57:25.112, switchValue=false)])@17520182
2024-02-20 20:57:25.134 30630-30630 chatty com.teratail.q_minbpx5ux7ywho I uid=10146(com.teratail.q_minbpx5ux7ywho) identical 1 line
2024-02-20 20:57:25.141 30630-30630 <no-tag> com.teratail.q_minbpx5ux7ywho D きているのかMutableState(value=[CardData(id=02d7dce8-a760-4d7a-bc98-704ccb021fe2, isParent=true, childId=null, alarmTime=20:57:23.150, switchValue=false), CardData(id=5d603422-afe5-4bcb-a5bb-c1323a758bb9, isParent=true, childId=null, alarmTime=20:57:24.455, switchValue=false), CardData(id=5756002d-d8a9-4c32-bd4c-a1487ee8079b, isParent=true, childId=null, alarmTime=20:57:25.112, switchValue=false)])@17520182
参考:
build.gradle.kts(:app) - dependencies
dependencies {
implementation("androidx.core:core-ktx:1.12.0")
implementation("androidx.lifecycle:lifecycle-runtime-ktx:2.7.0")
implementation("androidx.activity:activity-compose:1.8.2")
implementation(platform("androidx.compose:compose-bom:2023.08.00"))
implementation("androidx.compose.ui:ui")
implementation("androidx.compose.ui:ui-graphics")
implementation("androidx.compose.ui:ui-tooling-preview")
implementation("androidx.compose.material3:material3")
implementation("androidx.lifecycle:lifecycle-viewmodel-compose:2.7.0")
testImplementation("junit:junit:4.13.2")
androidTestImplementation("androidx.test.ext:junit:1.1.5")
androidTestImplementation("androidx.test.espresso:espresso-core:3.5.1")
androidTestImplementation(platform("androidx.compose:compose-bom:2023.08.00"))
androidTestImplementation("androidx.compose.ui:ui-test-junit4")
debugImplementation("androidx.compose.ui:ui-tooling")
debugImplementation("androidx.compose.ui:ui-test-manifest")
}
バッドをするには、ログインかつ
こちらの条件を満たす必要があります。
2024/02/21 12:49