回答編集履歴
10
クリック処理を忘れてました
answer
CHANGED
@@ -38,7 +38,7 @@
|
|
38
38
|
MainActivity.java
|
39
39
|
```java
|
40
40
|
import androidx.annotation.DrawableRes;
|
41
|
-
import androidx.appcompat.app.
|
41
|
+
import androidx.appcompat.app.*;
|
42
42
|
|
43
43
|
import android.os.Bundle;
|
44
44
|
import android.view.*;
|
@@ -71,6 +71,14 @@
|
|
71
71
|
|
72
72
|
ListView listView = findViewById(R.id.listView);
|
73
73
|
listView.setAdapter(new AnimalAdapter(animals));
|
74
|
+
listView.setOnItemClickListener((parent, view, position, id) -> {
|
75
|
+
Animal animal = (Animal)listView.getItemAtPosition(position);
|
76
|
+
new AlertDialog.Builder(MainActivity.this)
|
77
|
+
.setTitle(animal.name)
|
78
|
+
.setMessage(animal.explanation)
|
79
|
+
.setPositiveButton("yes",null)
|
80
|
+
.show();
|
81
|
+
});
|
74
82
|
}
|
75
83
|
|
76
84
|
private static class AnimalAdapter extends BaseAdapter {
|
@@ -164,4 +172,4 @@
|
|
164
172
|
</androidx.constraintlayout.widget.ConstraintLayout>
|
165
173
|
```
|
166
174
|
実行結果
|
167
|
-
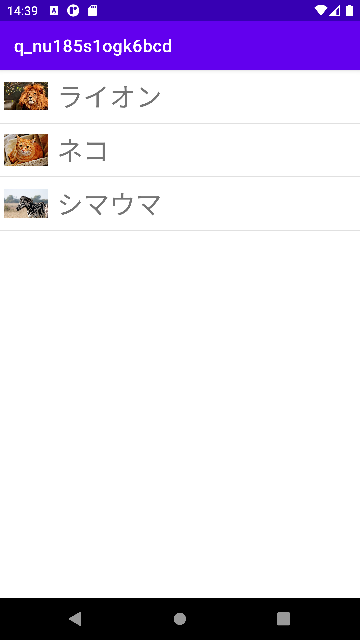
|
175
|
+
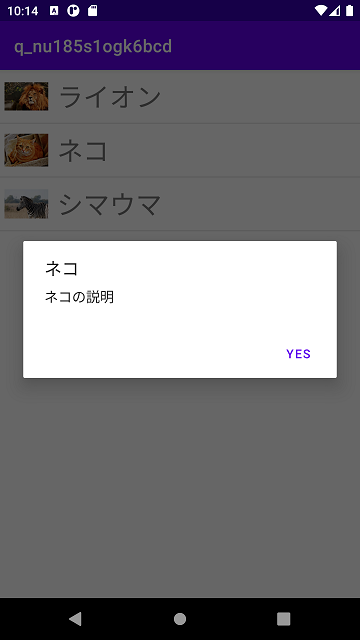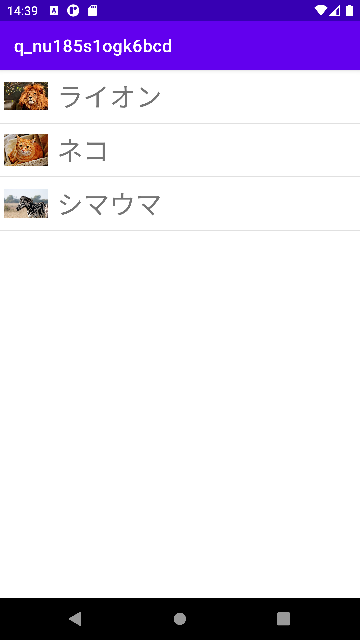
|
9
ViewHolder のフィールドを final にするのを忘れ
answer
CHANGED
@@ -75,8 +75,8 @@
|
|
75
75
|
|
76
76
|
private static class AnimalAdapter extends BaseAdapter {
|
77
77
|
private static class ViewHolder {
|
78
|
-
ImageView imageView;
|
78
|
+
final ImageView imageView;
|
79
|
-
TextView nameView;
|
79
|
+
final TextView nameView;
|
80
80
|
ViewHolder(View itemView) {
|
81
81
|
imageView = itemView.findViewById(R.id.image);
|
82
82
|
nameView = itemView.findViewById(R.id.name);
|
8
レイアウト修正
answer
CHANGED
@@ -160,8 +160,8 @@
|
|
160
160
|
app:layout_constraintBottom_toBottomOf="parent"
|
161
161
|
app:layout_constraintEnd_toEndOf="parent"
|
162
162
|
app:layout_constraintStart_toEndOf="@id/image"
|
163
|
-
app:layout_constraintTop_toTopOf="
|
163
|
+
app:layout_constraintTop_toTopOf="parent" />
|
164
164
|
</androidx.constraintlayout.widget.ConstraintLayout>
|
165
165
|
```
|
166
166
|
実行結果
|
167
|
-

|
7
修正
answer
CHANGED
@@ -1,6 +1,6 @@
|
|
1
1
|
まず、基本的な構造からです。
|
2
2
|
|
3
|
-
データ、データ2、画像の各要素が
|
3
|
+
データ、データ2、画像の各要素が1:1で関係し、例えば表にすると
|
4
4
|
|
5
5
|
|番号|データ(name)|データ2(explanation)|画像(image)|
|
6
6
|
|:--:|:--:|:--:|:--:|
|
@@ -8,7 +8,7 @@
|
|
8
8
|
|1|ネコ|ネコの説明|R.drawable.img16|
|
9
9
|
|2|シマウマ|シマウマの説明|R.drawable.img61|
|
10
10
|
|
11
|
-
を
|
11
|
+
を表す場合、"データ"の配列、"データ2"の配列、"画像"の配列という3つの配列を用意するのでは無く、"データ・データ2・画像の3つの情報を持つクラス"の配列1つを用意します。つまり縦割りでは無く横割りです。
|
12
12
|
```java
|
13
13
|
//1行分のデータが入っているクラスを新設
|
14
14
|
class Animal {
|
6
クラス名変更
answer
CHANGED
@@ -33,7 +33,7 @@
|
|
33
33
|
その上で、 ListView に表示するように構成したほうが良いと思います。
|
34
34
|
|
35
35
|
---
|
36
|
-
ArrayAdapter はテキスト1つの表示しか出来ません。同類の SimpleAdapter は Map 構造のデータで無いと使えません。ですので BaseAdapter から自作します。
|
36
|
+
ArrayAdapter はテキスト1つの表示しか出来ません。同類の SimpleAdapter は Map 構造のデータで無いと使えません。ですので BaseAdapter から自作します(AnimalAdapter)。
|
37
37
|
|
38
38
|
MainActivity.java
|
39
39
|
```java
|
@@ -70,10 +70,10 @@
|
|
70
70
|
};
|
71
71
|
|
72
72
|
ListView listView = findViewById(R.id.listView);
|
73
|
-
listView.setAdapter(new
|
73
|
+
listView.setAdapter(new AnimalAdapter(animals));
|
74
74
|
}
|
75
75
|
|
76
|
-
private static class
|
76
|
+
private static class AnimalAdapter extends BaseAdapter {
|
77
77
|
private static class ViewHolder {
|
78
78
|
ImageView imageView;
|
79
79
|
TextView nameView;
|
@@ -85,7 +85,7 @@
|
|
85
85
|
|
86
86
|
private List<Animal> animalList;
|
87
87
|
|
88
|
-
|
88
|
+
AnimalAdapter(Animal[] animals) {
|
89
89
|
this.animalList = Arrays.asList(animals);
|
90
90
|
}
|
91
91
|
|
5
追記
answer
CHANGED
@@ -33,6 +33,8 @@
|
|
33
33
|
その上で、 ListView に表示するように構成したほうが良いと思います。
|
34
34
|
|
35
35
|
---
|
36
|
+
ArrayAdapter はテキスト1つの表示しか出来ません。同類の SimpleAdapter は Map 構造のデータで無いと使えません。ですので BaseAdapter から自作します。
|
37
|
+
|
36
38
|
MainActivity.java
|
37
39
|
```java
|
38
40
|
import androidx.annotation.DrawableRes;
|
4
画像サイズ間違え
answer
CHANGED
@@ -162,4 +162,4 @@
|
|
162
162
|
</androidx.constraintlayout.widget.ConstraintLayout>
|
163
163
|
```
|
164
164
|
実行結果
|
165
|
-

|
3
コード追加
answer
CHANGED
@@ -30,4 +30,136 @@
|
|
30
30
|
```
|
31
31
|
これにより、各データの関係が明白になり、追加とか修正とかの場合にどれかの配列にやり忘れたとかも無くなります。
|
32
32
|
|
33
|
-
その上で、 ListView に表示するように構成したほうが良いと思います。
|
33
|
+
その上で、 ListView に表示するように構成したほうが良いと思います。
|
34
|
+
|
35
|
+
---
|
36
|
+
MainActivity.java
|
37
|
+
```java
|
38
|
+
import androidx.annotation.DrawableRes;
|
39
|
+
import androidx.appcompat.app.AppCompatActivity;
|
40
|
+
|
41
|
+
import android.os.Bundle;
|
42
|
+
import android.view.*;
|
43
|
+
import android.widget.*;
|
44
|
+
|
45
|
+
import java.util.*;
|
46
|
+
|
47
|
+
public class MainActivity extends AppCompatActivity {
|
48
|
+
private static class Animal {
|
49
|
+
final String name; //データ
|
50
|
+
final String explanation; //データ2
|
51
|
+
final @DrawableRes int image; //画像(リソースID)
|
52
|
+
Animal(String name, String explanation, @DrawableRes int image) {
|
53
|
+
this.name = name;
|
54
|
+
this.explanation = explanation;
|
55
|
+
this.image = image;
|
56
|
+
}
|
57
|
+
}
|
58
|
+
|
59
|
+
@Override
|
60
|
+
protected void onCreate(Bundle savedInstanceState) {
|
61
|
+
super.onCreate(savedInstanceState);
|
62
|
+
setContentView(R.layout.activity_main);
|
63
|
+
|
64
|
+
Animal[] animals = {
|
65
|
+
new Animal("ライオン", "ライオンの説明", R.drawable.img25),
|
66
|
+
new Animal("ネコ", "ネコの説明", R.drawable.img16),
|
67
|
+
new Animal("シマウマ", "シマウマの説明", R.drawable.img61)
|
68
|
+
};
|
69
|
+
|
70
|
+
ListView listView = findViewById(R.id.listView);
|
71
|
+
listView.setAdapter(new Adapter(animals));
|
72
|
+
}
|
73
|
+
|
74
|
+
private static class Adapter extends BaseAdapter {
|
75
|
+
private static class ViewHolder {
|
76
|
+
ImageView imageView;
|
77
|
+
TextView nameView;
|
78
|
+
ViewHolder(View itemView) {
|
79
|
+
imageView = itemView.findViewById(R.id.image);
|
80
|
+
nameView = itemView.findViewById(R.id.name);
|
81
|
+
}
|
82
|
+
}
|
83
|
+
|
84
|
+
private List<Animal> animalList;
|
85
|
+
|
86
|
+
Adapter(Animal[] animals) {
|
87
|
+
this.animalList = Arrays.asList(animals);
|
88
|
+
}
|
89
|
+
|
90
|
+
@Override
|
91
|
+
public int getCount() {
|
92
|
+
return animalList.size();
|
93
|
+
}
|
94
|
+
|
95
|
+
@Override
|
96
|
+
public Object getItem(int position) {
|
97
|
+
return animalList.get(position);
|
98
|
+
}
|
99
|
+
|
100
|
+
@Override
|
101
|
+
public long getItemId(int position) {
|
102
|
+
return position;
|
103
|
+
}
|
104
|
+
|
105
|
+
@Override
|
106
|
+
public View getView(int position, View convertView, ViewGroup parent) {
|
107
|
+
if(convertView == null) {
|
108
|
+
convertView = LayoutInflater.from(parent.getContext()).inflate(R.layout.list_item, parent, false);
|
109
|
+
convertView.setTag(new ViewHolder(convertView));
|
110
|
+
}
|
111
|
+
|
112
|
+
Animal animal = animalList.get(position);
|
113
|
+
ViewHolder vh = (ViewHolder)convertView.getTag();
|
114
|
+
vh.nameView.setText(animal.name);
|
115
|
+
vh.imageView.setImageResource(animal.image);
|
116
|
+
|
117
|
+
return convertView;
|
118
|
+
}
|
119
|
+
}
|
120
|
+
}
|
121
|
+
```
|
122
|
+
res/layout/activity_main.xml
|
123
|
+
```xml
|
124
|
+
<?xml version="1.0" encoding="utf-8"?>
|
125
|
+
<ListView
|
126
|
+
xmlns:android="http://schemas.android.com/apk/res/android"
|
127
|
+
xmlns:tools="http://schemas.android.com/tools"
|
128
|
+
android:id="@+id/listView"
|
129
|
+
android:layout_width="match_parent"
|
130
|
+
android:layout_height="match_parent"
|
131
|
+
tools:context=".MainActivity" />
|
132
|
+
```
|
133
|
+
res/layout/list_item.xml
|
134
|
+
```xml
|
135
|
+
<?xml version="1.0" encoding="utf-8"?>
|
136
|
+
<androidx.constraintlayout.widget.ConstraintLayout
|
137
|
+
xmlns:android="http://schemas.android.com/apk/res/android"
|
138
|
+
android:layout_width="match_parent"
|
139
|
+
android:layout_height="wrap_content"
|
140
|
+
xmlns:app="http://schemas.android.com/apk/res-auto">
|
141
|
+
|
142
|
+
<ImageView
|
143
|
+
android:id="@+id/image"
|
144
|
+
android:layout_width="50dp"
|
145
|
+
android:layout_height="50dp"
|
146
|
+
android:layout_margin="5dp"
|
147
|
+
app:layout_constraintBottom_toBottomOf="parent"
|
148
|
+
app:layout_constraintStart_toStartOf="parent"
|
149
|
+
app:layout_constraintTop_toTopOf="parent" />
|
150
|
+
<TextView
|
151
|
+
android:id="@+id/name"
|
152
|
+
android:layout_width="0dp"
|
153
|
+
android:layout_height="wrap_content"
|
154
|
+
android:textSize="30dp"
|
155
|
+
android:text="sample"
|
156
|
+
android:layout_marginStart="10dp"
|
157
|
+
android:layout_marginEnd="10dp"
|
158
|
+
app:layout_constraintBottom_toBottomOf="parent"
|
159
|
+
app:layout_constraintEnd_toEndOf="parent"
|
160
|
+
app:layout_constraintStart_toEndOf="@id/image"
|
161
|
+
app:layout_constraintTop_toTopOf="@id/image" />
|
162
|
+
</androidx.constraintlayout.widget.ConstraintLayout>
|
163
|
+
```
|
164
|
+
実行結果
|
165
|
+
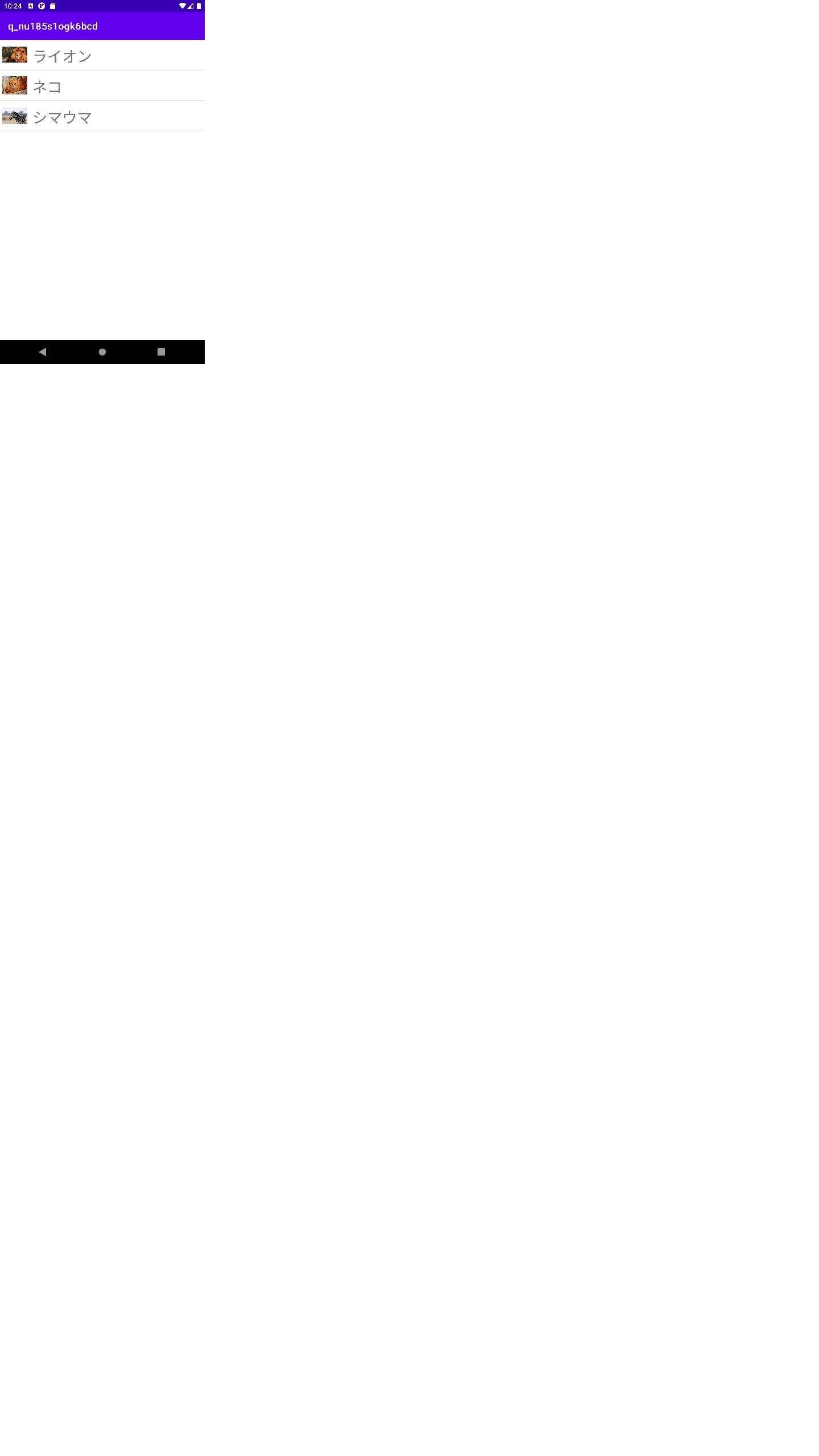
|
2
修正
answer
CHANGED
@@ -15,7 +15,7 @@
|
|
15
15
|
final String name; //データ
|
16
16
|
final String explanation; //データ2
|
17
17
|
final int image; //画像(リソースID)
|
18
|
-
|
18
|
+
Animal(String name, String explanation, int image) {
|
19
19
|
this.name = name;
|
20
20
|
this.explanation = explanation;
|
21
21
|
this.image = image;
|
1
修正
answer
CHANGED
@@ -2,7 +2,7 @@
|
|
2
2
|
|
3
3
|
データ、データ2、画像の各要素が配列のインデックス同士が関係あるという構造の場合、例えば表にすると
|
4
4
|
|
5
|
-
|番号|データ|データ2|画像|
|
5
|
+
|番号|データ(name)|データ2(explanation)|画像(image)|
|
6
6
|
|:--:|:--:|:--:|:--:|
|
7
7
|
|0|ライオン|ライオンの説明|R.drawable.img25|
|
8
8
|
|1|ネコ|ネコの説明|R.drawable.img16|
|