回答編集履歴
2
追記2
test
CHANGED
@@ -210,3 +210,47 @@
|
|
210
210
|
ちなみに、`styles` にある ` container` というプロパティ名は、そのコンポーネントの最も外側にある要素を示す名前としてしばしば使われます。
|
211
211
|
|
212
212
|
|
213
|
+
### 追記2
|
214
|
+
|
215
|
+
蛇足ですが、`src/components` ディレクトリに以下のような `index.js`
|
216
|
+
|
217
|
+
### src/components/index.js
|
218
|
+
|
219
|
+
```js
|
220
|
+
import About from "./about"
|
221
|
+
import Footer from "./footer"
|
222
|
+
import Header from "./header"
|
223
|
+
import Home from "./home"
|
224
|
+
import Service from "./service"
|
225
|
+
|
226
|
+
export {
|
227
|
+
About, Footer, Header, Home, Service
|
228
|
+
}
|
229
|
+
|
230
|
+
```
|
231
|
+
|
232
|
+
を作成しておくと、`App.js` で各コンポーネントをインポートする5行
|
233
|
+
|
234
|
+
```js
|
235
|
+
import Header from './components/header';
|
236
|
+
import Home from './components/home';
|
237
|
+
import Footer from './components/footer';
|
238
|
+
import Service from './components/service';
|
239
|
+
import About from './components/about';
|
240
|
+
```
|
241
|
+
|
242
|
+
|
243
|
+
を以下のように書けます。
|
244
|
+
|
245
|
+
```js
|
246
|
+
import {
|
247
|
+
About,
|
248
|
+
Footer,
|
249
|
+
Header,
|
250
|
+
Home,
|
251
|
+
Service
|
252
|
+
} from './components';
|
253
|
+
```
|
254
|
+
|
255
|
+
|
256
|
+
|
1
追記
test
CHANGED
@@ -14,3 +14,199 @@
|
|
14
14
|
<Route path={"/"} element={<Home/>} />
|
15
15
|
<Route path={"/about"} element={<About/>} />
|
16
16
|
```
|
17
|
+
|
18
|
+
### 追記
|
19
|
+
|
20
|
+
> ちなみに一般的なWEBサイト(メニューバーがトップにあり、メニューを押すとページが遷移するようなサイト)を作成するとして、この構成(?)構築?で進めていっても問題なさそうでしょうか??
|
21
|
+
|
22
|
+
についてですが、そのまま進めても作れないことはないですが、「こうしたほうがより良いのでは?」と個人的に思う修正案としては、
|
23
|
+
- `App.js` にはスタイルを書かないこと
|
24
|
+
|
25
|
+
を目標として、これの実現のために
|
26
|
+
|
27
|
+
- `App` の`<main>` タグの中にヘッダー`<Header>`があるのは不自然なので、`<main>` タグを一番外側に持つコンポーネント`Main`を作り、各画面のコンポーネントでこれを使用する
|
28
|
+
|
29
|
+
という修正が考えられます。たとえば以下のようにします。(適宜、スタイルを追加しています)
|
30
|
+
|
31
|
+
### components/main.jsx
|
32
|
+
以下を新規作成します。
|
33
|
+
```jsx
|
34
|
+
import React from 'react'
|
35
|
+
|
36
|
+
const Main = props => {
|
37
|
+
return (
|
38
|
+
<main style={styles.container}>
|
39
|
+
{props.children}
|
40
|
+
</main>
|
41
|
+
)
|
42
|
+
}
|
43
|
+
|
44
|
+
export default Main;
|
45
|
+
|
46
|
+
const styles = {
|
47
|
+
container: {
|
48
|
+
height: 300,
|
49
|
+
backgroundColor: '#dcf3ff',
|
50
|
+
fontSize: '24pt',
|
51
|
+
fontWeight: 'bold',
|
52
|
+
display: 'flex',
|
53
|
+
alignItems: 'center',
|
54
|
+
justifyContent: 'center',
|
55
|
+
}
|
56
|
+
};
|
57
|
+
|
58
|
+
```
|
59
|
+
|
60
|
+
`About`, `Home`, `Service` で上記のMainを使います。
|
61
|
+
|
62
|
+
|
63
|
+
### components/about.jsx
|
64
|
+
```jsx
|
65
|
+
import React from 'react'
|
66
|
+
import Main from "./main";
|
67
|
+
|
68
|
+
const About = () => {
|
69
|
+
return (
|
70
|
+
<Main>about</Main>
|
71
|
+
)
|
72
|
+
}
|
73
|
+
|
74
|
+
export default About
|
75
|
+
```
|
76
|
+
|
77
|
+
### components/home.jsx
|
78
|
+
```jsx
|
79
|
+
import React from 'react'
|
80
|
+
import Main from "./main";
|
81
|
+
|
82
|
+
const Home = () => {
|
83
|
+
return (
|
84
|
+
<Main>Home</Main>
|
85
|
+
)
|
86
|
+
}
|
87
|
+
|
88
|
+
export default Home;
|
89
|
+
|
90
|
+
```
|
91
|
+
|
92
|
+
### components/service.jsx
|
93
|
+
```jsx
|
94
|
+
import React from 'react'
|
95
|
+
import Main from "./main";
|
96
|
+
|
97
|
+
const Service = () => {
|
98
|
+
return (
|
99
|
+
<Main>service</Main>
|
100
|
+
)
|
101
|
+
}
|
102
|
+
|
103
|
+
export default Service
|
104
|
+
|
105
|
+
```
|
106
|
+
|
107
|
+
`footer.jsx` と `header.jsx` にもスタイルを移動します。
|
108
|
+
|
109
|
+
### components/footer.jsx
|
110
|
+
|
111
|
+
```jsx
|
112
|
+
import React from 'react'
|
113
|
+
|
114
|
+
const Footer = () => {
|
115
|
+
return (
|
116
|
+
<div style={styles.container}>footer</div>
|
117
|
+
)
|
118
|
+
}
|
119
|
+
|
120
|
+
export default Footer
|
121
|
+
|
122
|
+
const styles = {
|
123
|
+
container: {
|
124
|
+
height: 100,
|
125
|
+
background: "#ddd",
|
126
|
+
}
|
127
|
+
}
|
128
|
+
|
129
|
+
```
|
130
|
+
|
131
|
+
|
132
|
+
### components/header.jsx
|
133
|
+
|
134
|
+
```jsx
|
135
|
+
import React from 'react';
|
136
|
+
import { Link } from "react-router-dom";
|
137
|
+
|
138
|
+
const Header = () => {
|
139
|
+
return (
|
140
|
+
<div style={styles.container}>
|
141
|
+
<h1>タイトル</h1>
|
142
|
+
<div>
|
143
|
+
<Link to={`/`}>Home</Link>
|
144
|
+
</div>
|
145
|
+
<div>
|
146
|
+
<Link to={`/about/`}>About</Link>
|
147
|
+
</div>
|
148
|
+
<div>
|
149
|
+
<Link to={`/service/`}>Service</Link>
|
150
|
+
</div>
|
151
|
+
</div>
|
152
|
+
);
|
153
|
+
};
|
154
|
+
|
155
|
+
export default Header;
|
156
|
+
|
157
|
+
const styles = {
|
158
|
+
container: {
|
159
|
+
height: 100,
|
160
|
+
background: "#ddd",
|
161
|
+
display: 'flex',
|
162
|
+
alignItems: 'center',
|
163
|
+
justifyContent: 'space-around',
|
164
|
+
}
|
165
|
+
}
|
166
|
+
|
167
|
+
|
168
|
+
|
169
|
+
```
|
170
|
+
|
171
|
+
上記のように各コンポーネントを修正すると、以下のように App がすっきりします。
|
172
|
+
|
173
|
+
|
174
|
+
### App.js
|
175
|
+
|
176
|
+
```jsx
|
177
|
+
import './App.css';
|
178
|
+
import { BrowserRouter, Routes, Route } from 'react-router-dom';
|
179
|
+
import Header from './components/header';
|
180
|
+
import Home from './components/home';
|
181
|
+
import Footer from './components/footer';
|
182
|
+
import Service from './components/service';
|
183
|
+
import About from './components/about';
|
184
|
+
|
185
|
+
const App = () => {
|
186
|
+
return (
|
187
|
+
<div className="App">
|
188
|
+
<BrowserRouter>
|
189
|
+
<Header />
|
190
|
+
<Routes>
|
191
|
+
<Route path={"/"} element={<Home/>} />
|
192
|
+
<Route path={"/about"} element={<About/>} />
|
193
|
+
<Route path={"/service"} element={<Service/>} />
|
194
|
+
</Routes>
|
195
|
+
</BrowserRouter>
|
196
|
+
<Footer />
|
197
|
+
</div>
|
198
|
+
);
|
199
|
+
}
|
200
|
+
|
201
|
+
export default App;
|
202
|
+
|
203
|
+
|
204
|
+
```
|
205
|
+
|
206
|
+
表示させると、こんな感じになるかと思います。
|
207
|
+
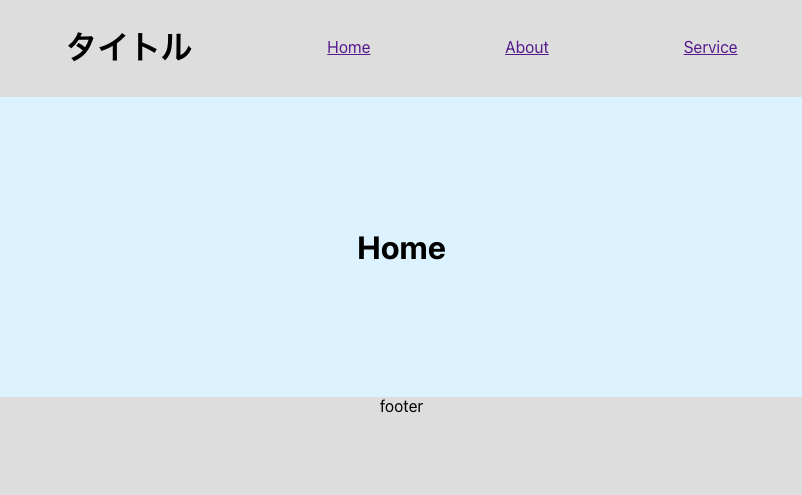
|
208
|
+
|
209
|
+
|
210
|
+
ちなみに、`styles` にある ` container` というプロパティ名は、そのコンポーネントの最も外側にある要素を示す名前としてしばしば使われます。
|
211
|
+
|
212
|
+
|