回答編集履歴
1
追記(より絞った例&コメント追加)
test
CHANGED
@@ -65,3 +65,82 @@
|
|
65
65
|
|
66
66
|
コード片だけではどこに書いたらいいのかわかりませんよね^^;
|
67
67
|
なので私は全文あげる派です^^
|
68
|
+
|
69
|
+
---
|
70
|
+
|
71
|
+
追記(より絞った例&コメント追加)
|
72
|
+
|
73
|
+
### 1. Panelをフォームに追加する
|
74
|
+
### 2. Panelのプロパティを変更
|
75
|
+
`Panel`が可変サイズになればなんでもいいがAnchorにした。
|
76
|
+
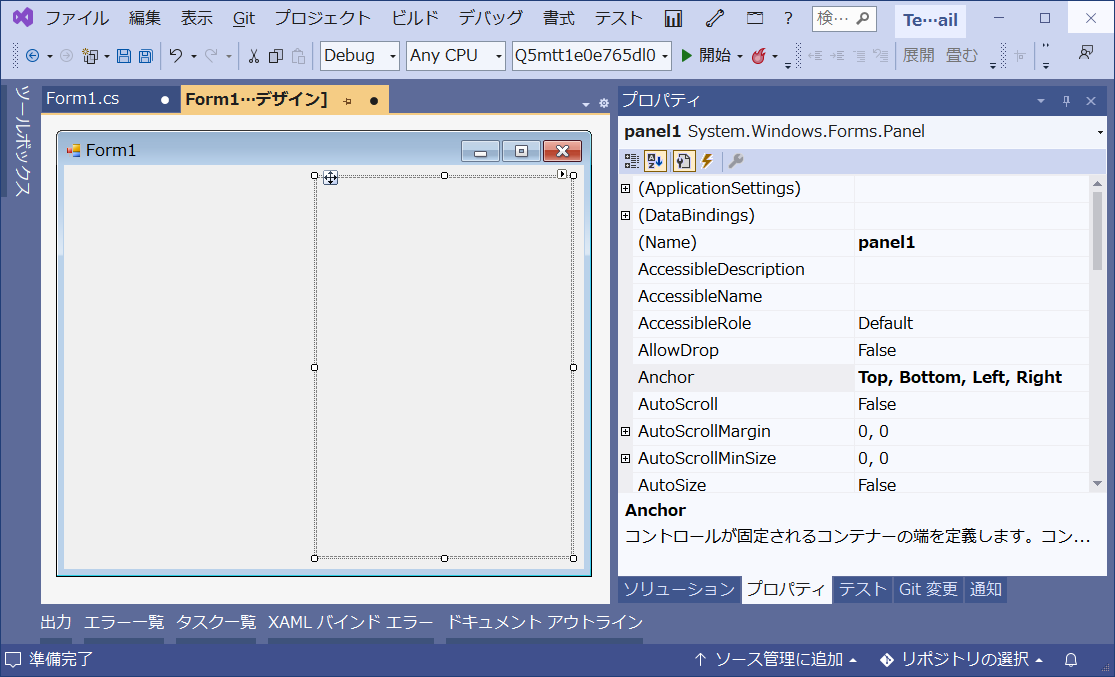
|
77
|
+
### 3. Panelのイベントを追加する
|
78
|
+
`Paint`・`Resize`イベントを追加
|
79
|
+
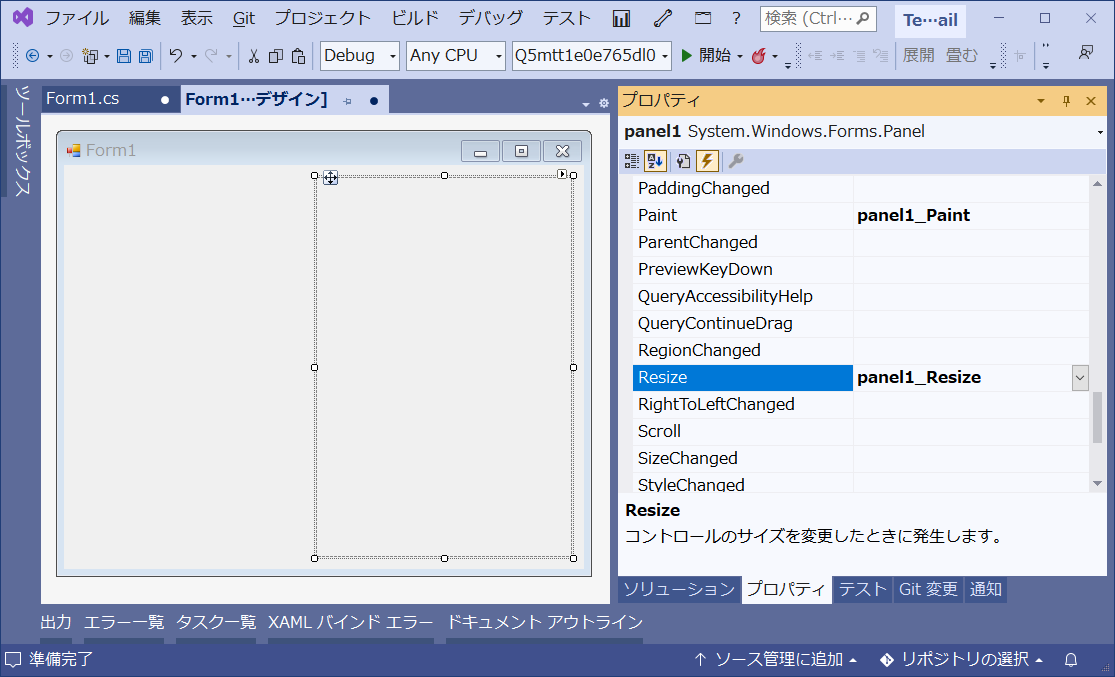
|
80
|
+
### 4. コード
|
81
|
+
```cs
|
82
|
+
using System;
|
83
|
+
using System.Collections.Generic;
|
84
|
+
using System.ComponentModel;
|
85
|
+
using System.Data;
|
86
|
+
using System.Drawing;
|
87
|
+
using System.Drawing.Drawing2D;
|
88
|
+
using System.Linq;
|
89
|
+
using System.Text;
|
90
|
+
using System.Threading.Tasks;
|
91
|
+
using System.Windows.Forms;
|
92
|
+
|
93
|
+
namespace Q5mtt1e0e765dl0
|
94
|
+
{
|
95
|
+
public partial class Form1 : Form
|
96
|
+
{
|
97
|
+
public Form1()
|
98
|
+
{
|
99
|
+
InitializeComponent();
|
100
|
+
}
|
101
|
+
|
102
|
+
// パネルを描画するときに呼び出される
|
103
|
+
private void panel1_Paint(object sender, PaintEventArgs e)
|
104
|
+
{
|
105
|
+
// ブロック({})を抜けたら自動的に Dispose するC#構文
|
106
|
+
using (
|
107
|
+
// グラデーションブラシ作成
|
108
|
+
var gb = new LinearGradientBrush(
|
109
|
+
// グラデーション範囲(表示クリッピング領域)
|
110
|
+
e.Graphics.VisibleClipBounds,
|
111
|
+
// グラデーション開始色(紺色)
|
112
|
+
Color.DarkBlue,
|
113
|
+
// グラデーション終了色(赤紫)
|
114
|
+
Color.MediumVioletRed,
|
115
|
+
// グラデーション方向(縦)
|
116
|
+
LinearGradientMode.Vertical))
|
117
|
+
{
|
118
|
+
// 四角形の内部を塗りつぶす(表示クリッピング領域)
|
119
|
+
e.Graphics.FillRectangle(gb, e.Graphics.VisibleClipBounds);
|
120
|
+
}
|
121
|
+
// using構文を使用したため Dispose を書く必要はない
|
122
|
+
//リソースを解放する
|
123
|
+
//gb.Dispose();
|
124
|
+
}
|
125
|
+
|
126
|
+
// パネルサイズが変わったときに呼び出される
|
127
|
+
private void panel1_Resize(object sender, EventArgs e)
|
128
|
+
{
|
129
|
+
// パネルの表面全体を無効化してパネルを再描画する
|
130
|
+
panel1.Invalidate();
|
131
|
+
}
|
132
|
+
}
|
133
|
+
}
|
134
|
+
```
|
135
|
+
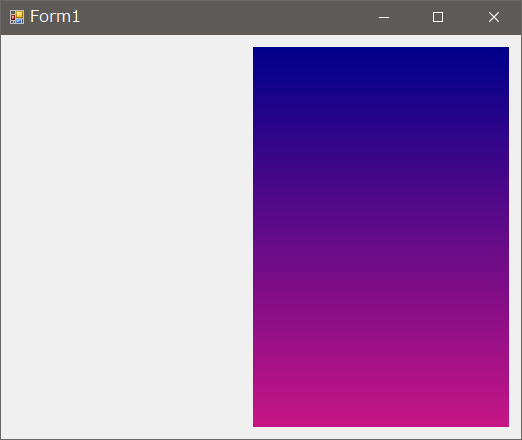
|
136
|
+
|
137
|
+
### 参考
|
138
|
+
[using ステートメント - C# リファレンス | Microsoft Learn](https://learn.microsoft.com/ja-jp/dotnet/csharp/language-reference/keywords/using-statement)
|
139
|
+
|
140
|
+
[LinearGradientBrush クラス (System.Drawing.Drawing2D) | Microsoft Learn](https://learn.microsoft.com/ja-jp/dotnet/api/system.drawing.drawing2d.lineargradientbrush?view=netframework-4.8)
|
141
|
+
|
142
|
+
[Graphics.VisibleClipBounds プロパティ (System.Drawing) | Microsoft Learn](https://learn.microsoft.com/ja-jp/dotnet/api/system.drawing.graphics.visibleclipbounds?view=netframework-4.8)
|
143
|
+
|
144
|
+
[Graphics.FillRectangle メソッド (System.Drawing) | Microsoft Learn](https://learn.microsoft.com/ja-jp/dotnet/api/system.drawing.graphics.fillrectangle?view=netframework-4.8#system-drawing-graphics-fillrectangle(system-drawing-brush-system-drawing-rectanglef))
|
145
|
+
|
146
|
+
[Control.Invalidate メソッド (System.Windows.Forms) | Microsoft Learn](https://learn.microsoft.com/ja-jp/dotnet/api/system.windows.forms.control.invalidate?view=netframework-4.8#system-windows-forms-control-invalidate)
|