回答編集履歴
4
コードバグ修正
test
CHANGED
@@ -94,12 +94,12 @@
|
|
94
94
|
private final List<Member> memberList = new ArrayList<>();
|
95
95
|
|
96
96
|
void addMember(@DrawableRes int id, String name) {
|
97
|
+
int position = dummyList.size() + memberList.size() / 2;
|
97
98
|
memberList.add(new Member(id, name));
|
98
|
-
int position = dummyList.size() + memberList.size() / 2;
|
99
|
-
if(memberList.size() % 2 ==
|
99
|
+
if(memberList.size() % 2 == 1) {
|
100
|
+
notifyItemInserted(position);
|
101
|
+
} else {
|
100
102
|
notifyItemChanged(position);
|
101
|
-
} else {
|
102
|
-
notifyItemInserted(position);
|
103
103
|
}
|
104
104
|
}
|
105
105
|
|
3
コード誤字等
test
CHANGED
@@ -48,8 +48,8 @@
|
|
48
48
|
*/
|
49
49
|
private static class RecyclerAdapter extends RecyclerView.Adapter<RecyclerAdapter.ViewHolder> {
|
50
50
|
private static abstract class ViewHolder extends RecyclerView.ViewHolder {
|
51
|
-
ViewHolder(@NonNull ViewGroup parent, @LayoutRes int re
|
51
|
+
ViewHolder(@NonNull ViewGroup parent, @LayoutRes int resource) {
|
52
|
-
super(LayoutInflater.from(parent.getContext()).inflate(re
|
52
|
+
super(LayoutInflater.from(parent.getContext()).inflate(resource, parent, false));
|
53
53
|
}
|
54
54
|
abstract void bind(int position);
|
55
55
|
}
|
@@ -143,7 +143,7 @@
|
|
143
143
|
case 0: return new DummyViewHolder(parent);
|
144
144
|
case 1: return new MemberViewHolder(parent);
|
145
145
|
}
|
146
|
-
|
146
|
+
throw new IllegalArgumentException("viewType=" + viewType);
|
147
147
|
}
|
148
148
|
|
149
149
|
@Override
|
2
コード等変更
test
CHANGED
@@ -1,39 +1,249 @@
|
|
1
1
|
少なくとも、 GridView は自前でスクロールしますので ScrollView は必要ありません。
|
2
2
|
|
3
|
+
---
|
4
|
+
|
5
|
+
500dp に当たる ( 仮に Dummy) 部分と、 GridView 的に 2 列で画像を表示する Member 部分を含めて全体がスクロールするような構造を RecyclerView で作ってみます。
|
6
|
+
Dummy 部分はテキトウにテキスト2行のデータとしています。
|
7
|
+
文字列から画像リソースIDを求める処理は関係無いので直接書いています。
|
8
|
+
```java
|
9
|
+
import androidx.annotation.*;
|
10
|
+
import androidx.appcompat.app.AppCompatActivity;
|
11
|
+
import androidx.recyclerview.widget.RecyclerView;
|
12
|
+
|
13
|
+
import android.os.Bundle;
|
14
|
+
import android.view.*;
|
15
|
+
import android.widget.*;
|
16
|
+
|
17
|
+
import java.util.ArrayList;
|
18
|
+
import java.util.List;
|
19
|
+
|
20
|
+
public class MainActivity extends AppCompatActivity {
|
21
|
+
@Override
|
22
|
+
protected void onCreate(Bundle savedInstanceState) {
|
23
|
+
super.onCreate(savedInstanceState);
|
24
|
+
setContentView(R.layout.activity_main);
|
25
|
+
|
26
|
+
RecyclerAdapter adapter = new RecyclerAdapter();
|
27
|
+
|
28
|
+
adapter.addDummy("Aaa", "111-1");
|
29
|
+
adapter.addDummy("Bbb", "222-22");
|
30
|
+
adapter.addDummy("Ccc", "333-333");
|
31
|
+
adapter.addDummy("Ddd", "444-4444");
|
32
|
+
adapter.addDummy("Eee", "555-55555");
|
33
|
+
adapter.addDummy("Fff", "666-666666");
|
34
|
+
adapter.addDummy("Ggg", "777-7777777");
|
35
|
+
|
36
|
+
adapter.addMember(R.drawable.sake, "酒");
|
37
|
+
adapter.addMember(R.drawable.store, "店");
|
38
|
+
adapter.addMember(R.drawable.group, "グループ");
|
39
|
+
adapter.addMember(R.drawable.group, "グループ2");
|
40
|
+
adapter.addMember(R.drawable.sample, "サンプル");
|
41
|
+
|
42
|
+
RecyclerView recyclerview = findViewById(R.id.recycler_view);
|
43
|
+
recyclerview.setAdapter(adapter);
|
44
|
+
}
|
45
|
+
|
46
|
+
/**
|
47
|
+
* Dummy (1行1件)・ Member (1行2件)を表示するアダプタ.
|
48
|
+
*/
|
49
|
+
private static class RecyclerAdapter extends RecyclerView.Adapter<RecyclerAdapter.ViewHolder> {
|
50
|
+
private static abstract class ViewHolder extends RecyclerView.ViewHolder {
|
51
|
+
ViewHolder(@NonNull ViewGroup parent, @LayoutRes int recource) {
|
52
|
+
super(LayoutInflater.from(parent.getContext()).inflate(recource, parent, false));
|
53
|
+
}
|
54
|
+
abstract void bind(int position);
|
55
|
+
}
|
56
|
+
|
57
|
+
private static class Dummy {
|
58
|
+
final String text1, text2;
|
59
|
+
Dummy(String text1, String text2) {
|
60
|
+
this.text1 = text1;
|
61
|
+
this.text2 = text2;
|
62
|
+
}
|
63
|
+
}
|
64
|
+
private final List<Dummy> dummyList = new ArrayList<>();
|
65
|
+
|
66
|
+
void addDummy(String text1, String text2) {
|
67
|
+
dummyList.add(new Dummy(text1, text2));
|
68
|
+
notifyItemInserted(dummyList.size() - 1);
|
69
|
+
}
|
70
|
+
|
71
|
+
private class DummyViewHolder extends ViewHolder {
|
72
|
+
private final TextView text1, text2;
|
73
|
+
DummyViewHolder(@NonNull ViewGroup parent) {
|
74
|
+
super(parent, R.layout.dummy_row);
|
75
|
+
text1 = itemView.findViewById(R.id.name1);
|
76
|
+
text2 = itemView.findViewById(R.id.name2);
|
77
|
+
}
|
78
|
+
@Override
|
79
|
+
void bind(int position) {
|
80
|
+
Dummy dummy = dummyList.get(position);
|
81
|
+
text1.setText(dummy.text1);
|
82
|
+
text2.setText(dummy.text2);
|
83
|
+
}
|
84
|
+
}
|
85
|
+
|
86
|
+
private static class Member {
|
87
|
+
final @DrawableRes int id;
|
88
|
+
final String name;
|
89
|
+
Member(@DrawableRes int id, String name) {
|
90
|
+
this.id = id;
|
91
|
+
this.name = name;
|
92
|
+
}
|
93
|
+
}
|
94
|
+
private final List<Member> memberList = new ArrayList<>();
|
95
|
+
|
96
|
+
void addMember(@DrawableRes int id, String name) {
|
97
|
+
memberList.add(new Member(id, name));
|
98
|
+
int position = dummyList.size() + memberList.size() / 2;
|
99
|
+
if(memberList.size() % 2 == 0) {
|
100
|
+
notifyItemChanged(position);
|
101
|
+
} else {
|
102
|
+
notifyItemInserted(position);
|
103
|
+
}
|
104
|
+
}
|
105
|
+
|
106
|
+
private class MemberViewHolder extends ViewHolder {
|
107
|
+
private final ImageView image1, image2;
|
108
|
+
private final TextView name1, name2;
|
109
|
+
MemberViewHolder(@NonNull ViewGroup parent) {
|
110
|
+
super(parent, R.layout.member_row);
|
111
|
+
image1 = itemView.findViewById(R.id.image1);
|
112
|
+
image2 = itemView.findViewById(R.id.image2);
|
113
|
+
name1 = itemView.findViewById(R.id.name1);
|
114
|
+
name2 = itemView.findViewById(R.id.name2);
|
115
|
+
}
|
116
|
+
@Override
|
117
|
+
void bind(int position) {
|
118
|
+
int index = (position - dummyList.size()) * 2;
|
119
|
+
Member member = memberList.get(index);
|
120
|
+
image1.setImageResource(member.id);
|
121
|
+
name1.setText(member.name);
|
122
|
+
|
123
|
+
if(++index < memberList.size()) {
|
124
|
+
member = memberList.get(index);
|
125
|
+
image2.setImageResource(member.id);
|
126
|
+
name2.setText(member.name);
|
127
|
+
} else {
|
128
|
+
image2.setImageResource(0);
|
129
|
+
name2.setText("");
|
130
|
+
}
|
131
|
+
}
|
132
|
+
}
|
133
|
+
|
134
|
+
@Override
|
135
|
+
public int getItemViewType(int position) {
|
136
|
+
return position < dummyList.size() ? 0 : 1;
|
137
|
+
}
|
138
|
+
|
139
|
+
@NonNull
|
140
|
+
@Override
|
141
|
+
public ViewHolder onCreateViewHolder(@NonNull ViewGroup parent, int viewType) {
|
142
|
+
switch(viewType) {
|
143
|
+
case 0: return new DummyViewHolder(parent);
|
144
|
+
case 1: return new MemberViewHolder(parent);
|
145
|
+
}
|
146
|
+
return null;
|
147
|
+
}
|
148
|
+
|
149
|
+
@Override
|
150
|
+
public void onBindViewHolder(@NonNull ViewHolder holder, int position) {
|
151
|
+
holder.bind(position);
|
152
|
+
}
|
153
|
+
|
154
|
+
@Override
|
155
|
+
public int getItemCount() {
|
156
|
+
return dummyList.size() + (memberList.size() + 1) / 2;
|
157
|
+
}
|
158
|
+
}
|
159
|
+
}
|
160
|
+
```
|
161
|
+
res/layout/activity_main.xml
|
162
|
+
```xml
|
163
|
+
<?xml version="1.0" encoding="utf-8"?>
|
164
|
+
<androidx.recyclerview.widget.RecyclerView xmlns:android="http://schemas.android.com/apk/res/android"
|
165
|
+
xmlns:tools="http://schemas.android.com/tools"
|
166
|
+
xmlns:app="http://schemas.android.com/apk/res-auto"
|
167
|
+
android:id="@+id/recycler_view"
|
168
|
+
android:layout_width="match_parent"
|
169
|
+
android:layout_height="match_parent"
|
170
|
+
app:layoutManager="androidx.recyclerview.widget.LinearLayoutManager"
|
171
|
+
tools:context=".MainActivity" />
|
172
|
+
```
|
173
|
+
res/layout/dummy_row.xml
|
3
174
|
```xml
|
4
175
|
<?xml version="1.0" encoding="utf-8"?>
|
5
176
|
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
|
6
177
|
xmlns:app="http://schemas.android.com/apk/res-auto"
|
7
|
-
xmlns:tools="http://schemas.android.com/tools"
|
8
178
|
android:layout_width="match_parent"
|
9
|
-
android:layout_height="
|
179
|
+
android:layout_height="wrap_content"
|
10
|
-
|
180
|
+
android:padding="3dp">
|
181
|
+
|
11
|
-
|
182
|
+
<TextView
|
12
|
-
|
183
|
+
android:id="@+id/name1"
|
13
|
-
android:layout_width="0dp"
|
184
|
+
android:layout_width="0dp"
|
14
|
-
android:layout_height="
|
185
|
+
android:layout_height="wrap_content"
|
15
|
-
android:
|
186
|
+
android:textSize="30dp"
|
16
|
-
app:layout_constraintBottom_toBottomOf="parent"
|
17
187
|
app:layout_constraintEnd_toEndOf="parent"
|
18
188
|
app:layout_constraintStart_toStartOf="parent"
|
19
|
-
app:layout_constraintTop_toTopOf="parent">
|
189
|
+
app:layout_constraintTop_toTopOf="parent" />
|
20
|
-
|
190
|
+
|
21
|
-
|
191
|
+
<TextView
|
22
|
-
|
192
|
+
android:id="@+id/name2"
|
23
|
-
|
193
|
+
android:layout_width="0dp"
|
24
|
-
|
194
|
+
android:layout_height="wrap_content"
|
25
|
-
android:gravity="center"
|
26
|
-
android:horizontalSpacing="1dp"
|
27
|
-
android:numColumns="2"
|
28
|
-
android:stretchMode="columnWidth"
|
29
|
-
|
195
|
+
android:textAlignment="textEnd"
|
30
|
-
app:layout_constraintBottom_toBottomOf="parent"
|
31
|
-
|
196
|
+
app:layout_constraintEnd_toEndOf="parent"
|
32
|
-
|
197
|
+
app:layout_constraintStart_toStartOf="parent"
|
33
|
-
|
198
|
+
app:layout_constraintTop_toBottomOf="@id/name1" />
|
34
|
-
|
35
|
-
</androidx.constraintlayout.widget.ConstraintLayout>
|
36
|
-
|
37
199
|
</androidx.constraintlayout.widget.ConstraintLayout>
|
38
200
|
```
|
201
|
+
res/layout/member_row.xml
|
202
|
+
```xml
|
203
|
+
<?xml version="1.0" encoding="utf-8"?>
|
204
|
+
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
|
205
|
+
xmlns:app="http://schemas.android.com/apk/res-auto"
|
206
|
+
android:layout_width="match_parent"
|
207
|
+
android:layout_height="wrap_content"
|
208
|
+
android:padding="3dp">
|
209
|
+
|
210
|
+
<ImageView
|
211
|
+
android:id="@+id/image1"
|
212
|
+
android:layout_width="0dp"
|
213
|
+
android:layout_height="140dp"
|
214
|
+
android:scaleType="centerCrop"
|
215
|
+
android:layout_margin="1dp"
|
216
|
+
app:layout_constraintEnd_toStartOf="@id/image2"
|
217
|
+
app:layout_constraintStart_toStartOf="parent"
|
218
|
+
app:layout_constraintTop_toTopOf="parent" />
|
219
|
+
<TextView
|
220
|
+
android:id="@+id/name1"
|
221
|
+
android:layout_width="0dp"
|
222
|
+
android:layout_height="wrap_content"
|
223
|
+
android:layout_marginTop="8dp"
|
224
|
+
android:gravity="center"
|
225
|
+
app:layout_constraintEnd_toEndOf="@id/image1"
|
226
|
+
app:layout_constraintStart_toStartOf="@id/image1"
|
227
|
+
app:layout_constraintTop_toBottomOf="@+id/image1" />
|
228
|
+
|
229
|
+
<ImageView
|
230
|
+
android:id="@+id/image2"
|
231
|
+
android:layout_width="0dp"
|
232
|
+
android:layout_height="140dp"
|
233
|
+
android:scaleType="centerCrop"
|
234
|
+
android:layout_margin="1dp"
|
235
|
+
app:layout_constraintEnd_toEndOf="parent"
|
236
|
+
app:layout_constraintStart_toEndOf="@id/image1"
|
237
|
+
app:layout_constraintTop_toTopOf="parent" />
|
238
|
+
<TextView
|
239
|
+
android:id="@+id/name2"
|
240
|
+
android:layout_width="0dp"
|
241
|
+
android:layout_height="wrap_content"
|
242
|
+
android:layout_marginTop="8dp"
|
243
|
+
android:gravity="center"
|
244
|
+
app:layout_constraintEnd_toEndOf="@id/image2"
|
245
|
+
app:layout_constraintStart_toStartOf="@id/image2"
|
246
|
+
app:layout_constraintTop_toBottomOf="@+id/image2" />
|
247
|
+
</androidx.constraintlayout.widget.ConstraintLayout>
|
248
|
+
```
|
39
|
-
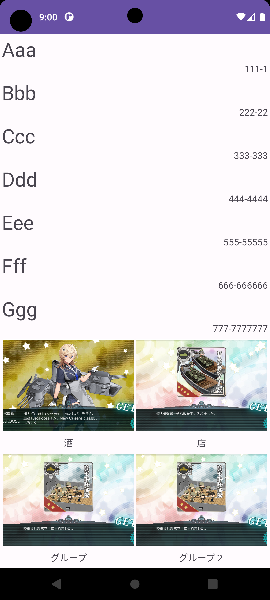
|
1
コード・SS追加
test
CHANGED
@@ -1 +1,39 @@
|
|
1
1
|
少なくとも、 GridView は自前でスクロールしますので ScrollView は必要ありません。
|
2
|
+
|
3
|
+
```xml
|
4
|
+
<?xml version="1.0" encoding="utf-8"?>
|
5
|
+
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
|
6
|
+
xmlns:app="http://schemas.android.com/apk/res-auto"
|
7
|
+
xmlns:tools="http://schemas.android.com/tools"
|
8
|
+
android:layout_width="match_parent"
|
9
|
+
android:layout_height="match_parent"
|
10
|
+
tools:context=".MainActivity">
|
11
|
+
|
12
|
+
<androidx.constraintlayout.widget.ConstraintLayout
|
13
|
+
android:layout_width="0dp"
|
14
|
+
android:layout_height="0dp"
|
15
|
+
android:layout_marginTop="500dp"
|
16
|
+
app:layout_constraintBottom_toBottomOf="parent"
|
17
|
+
app:layout_constraintEnd_toEndOf="parent"
|
18
|
+
app:layout_constraintStart_toStartOf="parent"
|
19
|
+
app:layout_constraintTop_toTopOf="parent">
|
20
|
+
|
21
|
+
<GridView
|
22
|
+
android:id="@+id/gridview"
|
23
|
+
android:layout_width="0dp"
|
24
|
+
android:layout_height="0dp"
|
25
|
+
android:gravity="center"
|
26
|
+
android:horizontalSpacing="1dp"
|
27
|
+
android:numColumns="2"
|
28
|
+
android:stretchMode="columnWidth"
|
29
|
+
android:verticalSpacing="1dp"
|
30
|
+
app:layout_constraintBottom_toBottomOf="parent"
|
31
|
+
app:layout_constraintEnd_toEndOf="parent"
|
32
|
+
app:layout_constraintStart_toStartOf="parent"
|
33
|
+
app:layout_constraintTop_toTopOf="parent" />
|
34
|
+
|
35
|
+
</androidx.constraintlayout.widget.ConstraintLayout>
|
36
|
+
|
37
|
+
</androidx.constraintlayout.widget.ConstraintLayout>
|
38
|
+
```
|
39
|
+
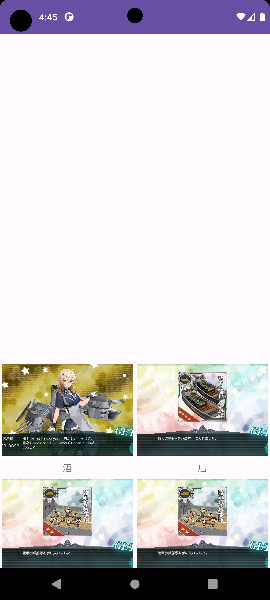
|