回答編集履歴
6
修正
test
CHANGED
@@ -110,8 +110,8 @@
|
|
110
110
|
public void onCreate(SQLiteDatabase db) {
|
111
111
|
db.execSQL("CREATE TABLE table1 (_id INTEGER PRIMARY KEY, name TEXT, toggle INTEGER)");
|
112
112
|
|
113
|
-
db.execSQL("INSERT INTO table1 (_id,name,toggle)
|
113
|
+
db.execSQL("INSERT INTO table1 (_id,name,toggle) VALUES (1,'ABC',0)");
|
114
|
-
db.execSQL("INSERT INTO table1 (_id,name,toggle)
|
114
|
+
db.execSQL("INSERT INTO table1 (_id,name,toggle) VALUES (2,'XYZ',1)");
|
115
115
|
}
|
116
116
|
|
117
117
|
@Override
|
5
コード追加
test
CHANGED
@@ -7,3 +7,119 @@
|
|
7
7
|
→[Y.A.M の 雑記帳 - Android SimpleCursorAdapter で ViewBinder を使う](http://y-anz-m.blogspot.com/2011/10/simplecursoradapter-viewbinder.html?m=0)
|
8
8
|
|
9
9
|
getView を上書きしてどうにかしたいのでしたら、 SimpleCursorAdapter のソースコードを確認してどのようにすれば良いのかを調べるべきに思います。
|
10
|
+
|
11
|
+
----
|
12
|
+
|
13
|
+
MainActivity.java
|
14
|
+
```java
|
15
|
+
import androidx.appcompat.app.AppCompatActivity;
|
16
|
+
import androidx.appcompat.widget.SwitchCompat;
|
17
|
+
import androidx.cursoradapter.widget.SimpleCursorAdapter;
|
18
|
+
|
19
|
+
import android.database.Cursor;
|
20
|
+
import android.database.sqlite.*;
|
21
|
+
import android.os.Bundle;
|
22
|
+
import android.widget.ListView;
|
23
|
+
|
24
|
+
public class MainActivity extends AppCompatActivity {
|
25
|
+
@Override
|
26
|
+
protected void onCreate(Bundle savedInstanceState) {
|
27
|
+
super.onCreate(savedInstanceState);
|
28
|
+
setContentView(R.layout.activity_main);
|
29
|
+
|
30
|
+
SQLiteOpenHelper helper = new DatabaseHelper(this);
|
31
|
+
SQLiteDatabase db = helper.getReadableDatabase();
|
32
|
+
Cursor cursor = db.rawQuery("SELECT _id,name,toggle FROM table1", null);
|
33
|
+
|
34
|
+
SimpleCursorAdapter adapter = new SimpleCursorAdapter(this, R.layout.list_item, cursor,
|
35
|
+
new String[]{"_id", "name", "toggle"},
|
36
|
+
new int[]{R.id.id, R.id.name, R.id.toggle},
|
37
|
+
0);
|
38
|
+
adapter.setViewBinder((v, c, i) -> {
|
39
|
+
if(i == c.getColumnIndex("toggle")) {
|
40
|
+
((SwitchCompat)v).setChecked(c.getInt(i) == 1);
|
41
|
+
return true;
|
42
|
+
}
|
43
|
+
return false;
|
44
|
+
});
|
45
|
+
|
46
|
+
ListView listview = findViewById(R.id.listview);
|
47
|
+
listview.setAdapter(adapter);
|
48
|
+
}
|
49
|
+
}
|
50
|
+
```
|
51
|
+
res/layout/activity_main.xml
|
52
|
+
```xml
|
53
|
+
<?xml version="1.0" encoding="utf-8"?>
|
54
|
+
<ListView
|
55
|
+
android:id="@+id/listview"
|
56
|
+
xmlns:android="http://schemas.android.com/apk/res/android"
|
57
|
+
xmlns:tools="http://schemas.android.com/tools"
|
58
|
+
android:layout_width="match_parent"
|
59
|
+
android:layout_height="match_parent"
|
60
|
+
tools:context=".MainActivity" />
|
61
|
+
```
|
62
|
+
res/layout/list_item.xml
|
63
|
+
```xml
|
64
|
+
<?xml version="1.0" encoding="utf-8"?>
|
65
|
+
<androidx.constraintlayout.widget.ConstraintLayout
|
66
|
+
xmlns:android="http://schemas.android.com/apk/res/android"
|
67
|
+
xmlns:app="http://schemas.android.com/apk/res-auto"
|
68
|
+
android:layout_width="match_parent"
|
69
|
+
android:layout_height="wrap_content">
|
70
|
+
|
71
|
+
<androidx.appcompat.widget.SwitchCompat
|
72
|
+
android:id="@+id/toggle"
|
73
|
+
android:layout_width="wrap_content"
|
74
|
+
android:layout_height="0dp"
|
75
|
+
android:paddingEnd="10dp"
|
76
|
+
app:layout_constraintBottom_toBottomOf="parent"
|
77
|
+
app:layout_constraintStart_toStartOf="parent"
|
78
|
+
app:layout_constraintTop_toTopOf="parent" />
|
79
|
+
<TextView
|
80
|
+
android:id="@+id/id"
|
81
|
+
android:layout_width="0dp"
|
82
|
+
android:layout_height="wrap_content"
|
83
|
+
android:text="_id"
|
84
|
+
app:layout_constraintEnd_toEndOf="parent"
|
85
|
+
app:layout_constraintStart_toEndOf="@id/toggle"
|
86
|
+
app:layout_constraintTop_toTopOf="parent" />
|
87
|
+
<TextView
|
88
|
+
android:id="@+id/name"
|
89
|
+
android:layout_width="0dp"
|
90
|
+
android:layout_height="wrap_content"
|
91
|
+
android:text="name"
|
92
|
+
app:layout_constraintEnd_toEndOf="parent"
|
93
|
+
app:layout_constraintStart_toEndOf="@id/toggle"
|
94
|
+
app:layout_constraintTop_toBottomOf="@id/id" />
|
95
|
+
</androidx.constraintlayout.widget.ConstraintLayout>
|
96
|
+
```
|
97
|
+
DatabaseHelper.java
|
98
|
+
```java
|
99
|
+
import android.content.Context;
|
100
|
+
import android.database.sqlite.*;
|
101
|
+
|
102
|
+
import androidx.annotation.*;
|
103
|
+
|
104
|
+
public class DatabaseHelper extends SQLiteOpenHelper {
|
105
|
+
public DatabaseHelper(@Nullable Context context) {
|
106
|
+
super(context, "testdb", null, 1);
|
107
|
+
}
|
108
|
+
|
109
|
+
@Override
|
110
|
+
public void onCreate(SQLiteDatabase db) {
|
111
|
+
db.execSQL("CREATE TABLE table1 (_id INTEGER PRIMARY KEY, name TEXT, toggle INTEGER)");
|
112
|
+
|
113
|
+
db.execSQL("INSERT INTO table1 (_id,name,toggle) values (1,'ABC',0)");
|
114
|
+
db.execSQL("INSERT INTO table1 (_id,name,toggle) values (2,'XYZ',1)");
|
115
|
+
}
|
116
|
+
|
117
|
+
@Override
|
118
|
+
public void onUpgrade(SQLiteDatabase db, int oldVersion, int newVersion) {
|
119
|
+
db.execSQL("DROP TABLE IF EXISTS table1");
|
120
|
+
onCreate(db);
|
121
|
+
}
|
122
|
+
}
|
123
|
+
```
|
124
|
+
実行結果
|
125
|
+
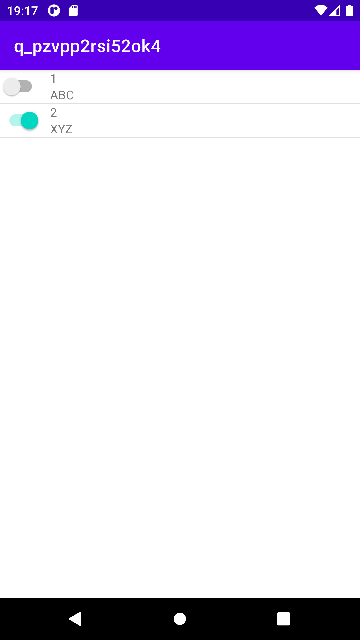
|
4
リンク修正
test
CHANGED
@@ -4,6 +4,6 @@
|
|
4
4
|
|
5
5
|
と書かれていますので、トグルボタンには対応していません。
|
6
6
|
対応していないビューに対して動作させたい場合は、 [SimpleCursorAdapter.ViewBinder](https://developer.android.com/reference/android/widget/SimpleCursorAdapter.ViewBinder) を用いるようです。
|
7
|
-
→[Y.A.M の 雑記帳 - Android SimpleCursorAdapter で ViewBinder を使う](http://y-anz-m.blogspot.com/2011/10/simplecursoradapter-viewbinder.html?m=
|
7
|
+
→[Y.A.M の 雑記帳 - Android SimpleCursorAdapter で ViewBinder を使う](http://y-anz-m.blogspot.com/2011/10/simplecursoradapter-viewbinder.html?m=0)
|
8
8
|
|
9
9
|
getView を上書きしてどうにかしたいのでしたら、 SimpleCursorAdapter のソースコードを確認してどのようにすれば良いのかを調べるべきに思います。
|
3
追加
test
CHANGED
@@ -4,5 +4,6 @@
|
|
4
4
|
|
5
5
|
と書かれていますので、トグルボタンには対応していません。
|
6
6
|
対応していないビューに対して動作させたい場合は、 [SimpleCursorAdapter.ViewBinder](https://developer.android.com/reference/android/widget/SimpleCursorAdapter.ViewBinder) を用いるようです。
|
7
|
+
→[Y.A.M の 雑記帳 - Android SimpleCursorAdapter で ViewBinder を使う](http://y-anz-m.blogspot.com/2011/10/simplecursoradapter-viewbinder.html?m=1)
|
7
8
|
|
8
9
|
getView を上書きしてどうにかしたいのでしたら、 SimpleCursorAdapter のソースコードを確認してどのようにすれば良いのかを調べるべきに思います。
|
2
追加
test
CHANGED
@@ -3,5 +3,6 @@
|
|
3
3
|
「カーソルから XML ファイルで定義された TextViews または ImageViews に列をマップするための簡単なアダプター。」by Google翻訳
|
4
4
|
|
5
5
|
と書かれていますので、トグルボタンには対応していません。
|
6
|
+
対応していないビューに対して動作させたい場合は、 [SimpleCursorAdapter.ViewBinder](https://developer.android.com/reference/android/widget/SimpleCursorAdapter.ViewBinder) を用いるようです。
|
6
7
|
|
7
|
-
getView を上書きして
|
8
|
+
getView を上書きしてどうにかしたいのでしたら、 SimpleCursorAdapter のソースコードを確認してどのようにすれば良いのかを調べるべきに思います。
|
1
追加
test
CHANGED
@@ -3,3 +3,5 @@
|
|
3
3
|
「カーソルから XML ファイルで定義された TextViews または ImageViews に列をマップするための簡単なアダプター。」by Google翻訳
|
4
4
|
|
5
5
|
と書かれていますので、トグルボタンには対応していません。
|
6
|
+
|
7
|
+
getView を上書きしてでもどうにかしたいのでしたら、 SimpleCursorAdapter のソースコードを確認してどのようにすれば良いのかを調べるべきに思います。
|