質問編集履歴
3
コードを議論したい部分だけにフォーカスするように変更しました。
test
CHANGED
File without changes
|
test
CHANGED
@@ -6,12 +6,7 @@
|
|
6
6
|
import RealmSwift
|
7
7
|
|
8
8
|
class MyModel: Object {
|
9
|
-
@objc dynamic var imageURL: String = "" //画像のurl
|
10
|
-
@objc dynamic var imageX: Double = 0.0 // 画像のx座標
|
11
|
-
@objc dynamic var imageY: Double = 0.0 // 画像のy座標
|
12
|
-
@objc dynamic var imageWidth: Double = 0.0 // 画像の幅
|
13
|
-
@objc dynamic var imageHeight: Double = 0.0 // 画像の高さ
|
14
|
-
@objc dynamic var
|
9
|
+
@objc dynamic var imageURL: String = ""
|
15
10
|
@objc dynamic var id: String = UUID().uuidString
|
16
11
|
override static func primaryKey() -> String? {
|
17
12
|
return "id"
|
@@ -30,87 +25,72 @@
|
|
30
25
|
|
31
26
|
```Swift
|
32
27
|
// 保存
|
28
|
+
var documentDirectoryFileURL = FileManager.default.urls(for: .documentDirectory, in: .userDomainMask)[0]
|
29
|
+
let filePath = NSSearchPathForDirectoriesInDomains(.documentDirectory, .userDomainMask, true)[0]
|
30
|
+
|
33
31
|
func picker(_ picker: PHPickerViewController, didFinishPicking results: [PHPickerResult]) {
|
34
|
-
let widthSize = 200.0
|
35
|
-
let heightSize = 100.0
|
36
|
-
let imageViewBackground = UIImageView()
|
37
|
-
imageViewBackground.center = CGPoint(x: bgView.center.x, y: bgView.center.y)
|
38
|
-
imageViewBackground.bounds = CGRect(x: 0, y: 0, width: widthSize, height: heightSize)
|
39
|
-
imageViewBackground.accessibilityIdentifier = UUID().uuidString
|
40
|
-
|
41
|
-
let yearFormatter = DateFormatter()
|
42
|
-
yearFormatter.dateFormat = DateFormatter.dateFormat(fromTemplate: "yyy", options: 0, locale: Locale(identifier: "jp_EN"))
|
43
|
-
let monthFormatter = DateFormatter()
|
44
|
-
monthFormatter.dateFormat = DateFormatter.dateFormat(fromTemplate: "MM", options: 0, locale: Locale(identifier: "jp_EN"))
|
45
|
-
let dayFormatter = DateFormatter()
|
46
|
-
dayFormatter.dateFormat = DateFormatter.dateFormat(fromTemplate: "dd", options: 0, locale: Locale(identifier: "jp_EN"))
|
47
|
-
let year = yearFormatter.string(from: Date())
|
48
|
-
let month = monthFormatter.string(from: Date())
|
49
|
-
let day = dayFormatter.string(from: Date())
|
50
|
-
|
51
|
-
let imageModel = MyModel()
|
52
|
-
let realm = try! Realm()
|
53
|
-
do {
|
54
|
-
imageModel.imageURL = documentDirectoryFileURL.absoluteString
|
55
|
-
imageModel.imageX = imageViewBackground.center.x
|
56
|
-
imageModel.imageY = imageViewBackground.center.y
|
57
|
-
imageModel.imageWidth = widthSize
|
58
|
-
imageModel.imageHeight = heightSize
|
59
|
-
imageModel.date = "\(year).\(month).\(day)"
|
60
|
-
imageModel.id = imageViewBackground.accessibilityIdentifier!
|
61
|
-
} catch {
|
62
|
-
print("failed to save image.")
|
63
|
-
}
|
64
|
-
try! realm.write{realm.add(imageModel)}
|
65
|
-
print("imageModel: ", imageModel)
|
66
|
-
|
67
32
|
if results.count != 0 {
|
68
33
|
results[0].itemProvider.loadDataRepresentation(forTypeIdentifier: "public.image") { data, _ in
|
69
34
|
DispatchQueue.main.async {
|
70
|
-
image
|
35
|
+
if let imageData = data, let image = UIImage(data: imageData) {
|
36
|
+
self.imageView.image = image
|
37
|
+
|
38
|
+
let model = MyModel()
|
39
|
+
do {
|
40
|
+
self.saveImage()
|
71
|
-
|
41
|
+
try model.imageURL = self.documentDirectoryFileURL.absoluteString
|
42
|
+
print("successfully saved image!")
|
43
|
+
} catch {
|
44
|
+
print("Failed to save image")
|
45
|
+
}
|
46
|
+
try! self.realm.write({
|
47
|
+
self.realm.add(model)
|
48
|
+
})
|
49
|
+
print("model: ", model)
|
50
|
+
}
|
72
51
|
}
|
73
52
|
}
|
74
53
|
}
|
75
54
|
picker.dismiss(animated: true)
|
76
55
|
}
|
56
|
+
|
57
|
+
func createLocalDataFile() {
|
58
|
+
let fileName = "\(UUID().uuidString).png"
|
59
|
+
if documentDirectoryFileURL != nil {
|
60
|
+
let path = documentDirectoryFileURL.appendingPathComponent(fileName)
|
61
|
+
documentDirectoryFileURL = path
|
62
|
+
}
|
63
|
+
}
|
64
|
+
|
65
|
+
func saveImage() {
|
66
|
+
createLocalDataFile()
|
67
|
+
let pngImageData = imageView.image?.pngData()
|
68
|
+
do {
|
69
|
+
try pngImageData?.write(to: documentDirectoryFileURL)
|
70
|
+
} catch {
|
71
|
+
print("error")
|
72
|
+
}
|
73
|
+
}
|
77
74
|
```
|
78
75
|
|
79
76
|
```Swift
|
80
77
|
// 表示
|
81
|
-
var i
|
78
|
+
var itemList: Results<MyModel>!
|
82
79
|
override func viewWillAppear(_ animated: Bool) {
|
83
80
|
super.viewWillAppear(animated)
|
84
|
-
let yearFormatter = DateFormatter()
|
85
|
-
yearFormatter.dateFormat = DateFormatter.dateFormat(fromTemplate: "yyy", options: 0, locale: Locale(identifier: "jp_EN"))
|
86
|
-
|
81
|
+
itemList = realm.objects(MyModel.self)
|
87
|
-
monthFormatter.dateFormat = DateFormatter.dateFormat(fromTemplate: "MM", options: 0, locale: Locale(identifier: "jp_EN"))
|
88
|
-
let dayFormatter = DateFormatter()
|
89
|
-
dayFormatter.dateFormat = DateFormatter.dateFormat(fromTemplate: "dd", options: 0, locale: Locale(identifier: "jp_EN"))
|
90
|
-
let year = yearFormatter.string(from: Date())
|
91
|
-
let month = monthFormatter.string(from: Date())
|
92
|
-
let day = dayFormatter.string(from: Date())
|
93
|
-
yearLabel.text = "\(year)"
|
94
|
-
dateLabel.text = "\(month).\(day)"
|
95
|
-
|
96
|
-
// realm
|
97
|
-
let realm = try! Realm()
|
98
|
-
self.imageItemList = realm.objects(MyModel.self).filter("date == %@", "\(year).\(month).\(day)")
|
99
|
-
print(im
|
82
|
+
print("itemList: ", itemList!)
|
100
|
-
|
101
|
-
// ユーザーが指定したx, y座標、sizeの画像を表示する
|
102
|
-
f
|
83
|
+
if (itemList.count != 0) {
|
103
|
-
let imageView = UIImageView()
|
104
|
-
imageView.frame.size = CGSize(width: imageItemList[i].imageWidth, height: imageItemList[i].imageHeight)
|
105
|
-
imageView.center = CGPoint(x: imageItemList[i].imageX, y: imageItemList[i].imageY)
|
106
|
-
|
107
|
-
let fileURL = URL(string: i
|
84
|
+
let fileURL = URL(string: itemList[0].imageURL)
|
108
|
-
let filePath = fileURL?.path
|
109
85
|
print("fileURL: ", fileURL!)
|
86
|
+
do {
|
87
|
+
let data = try Data(contentsOf: fileURL!)
|
110
|
-
print("
|
88
|
+
print("data: ", data)
|
111
|
-
|
112
|
-
|
89
|
+
let image = UIImage(data: data)
|
113
|
-
|
90
|
+
imageView.image = image
|
91
|
+
} catch let err {
|
92
|
+
print("Error: \(err.localizedDescription)")
|
93
|
+
}
|
114
94
|
}
|
115
95
|
}
|
116
96
|
```
|
@@ -125,62 +105,31 @@
|
|
125
105
|
画像を保存した時及び表示する際の出力結果は以下のようになりました。
|
126
106
|
```
|
127
107
|
// 保存時
|
108
|
+
successfully saved image!
|
128
|
-
|
109
|
+
model: MyModel {
|
129
|
-
imageURL = file:///...
|
110
|
+
imageURL = file:///....../37AC5833-DE4F-4321-8A87-3734F28C51B9/Documents/0D41ED77-C001-45DA-814D-D79F96358C5B.png;
|
130
|
-
imageX = 214;
|
131
|
-
imageY = 354.1666666666666;
|
132
|
-
imageWidth = 200;
|
133
|
-
imageHeight = 100;
|
134
|
-
date = 2024.08.26;
|
135
|
-
id = A
|
111
|
+
id = 6EC2C4AD-8657-45C6-BC41-9BE24AB7F268;
|
136
112
|
}
|
113
|
+
|
137
114
|
```
|
138
115
|
```
|
139
116
|
// 表示 - Modelの中身
|
140
|
-
Results<MyModel> <0x7f8
|
117
|
+
itemList: Results<MyModel> <0x7f9544413830> (
|
141
118
|
[0] MyModel {
|
142
|
-
//...中略...//
|
143
|
-
},
|
144
|
-
[1] MyModel {
|
145
|
-
//...中略...//
|
146
|
-
},
|
147
|
-
[2] MyModel {
|
148
|
-
imageURL = file:///...
|
119
|
+
imageURL = file:///....../37AC5833-DE4F-4321-8A87-3734F28C51B9/Documents/0D41ED77-C001-45DA-814D-D79F96358C5B.png;
|
149
|
-
imageX = 214;
|
150
|
-
imageY = 354.1666666666666;
|
151
|
-
imageWidth = 200;
|
152
|
-
imageHeight = 100;
|
153
|
-
date = 2024.08.26;
|
154
|
-
id = A
|
120
|
+
id = 6EC2C4AD-8657-45C6-BC41-9BE24AB7F268;
|
155
121
|
}
|
156
122
|
)
|
157
|
-
// 表示 - URL
|
123
|
+
// 表示 - URL
|
158
|
-
fileURL: file:///...
|
124
|
+
fileURL: file:///.../37AC5833-DE4F-4321-8A87-3734F28C51B9/Documents/0D41ED77-C001-45DA-814D-D79F96358C5B.png
|
159
|
-
filePath: /...中略.../479967A6-E315-4C0B-8FB0-687F185DD299/data/Containers/Data/Application/4B03C2DC-2C13-421D-8F0D-41EBAF5DCA3E/Documents
|
160
125
|
```
|
161
126
|
|
162
|
-
表示する時のコードを以下のように変えたところ、File does not exist at path:... と表示されました。
|
163
|
-
```Swift
|
164
|
-
for i in 0..<imageItemList.count {
|
165
|
-
|
127
|
+
表示する際にコンソールに以下のように出力されました。
|
166
|
-
|
128
|
+
> Error: The file “0D41ED77-C001-45DA-814D-D79F96358C5B.png” couldn’t be opened because there is no such file.
|
129
|
+
|
167
|
-
|
130
|
+
また、Finderから保存されている写真を探したところ、添付写真の場所にあったのですが、これは保存してあるURLと違うフォルダ名になっていましたので、これが原因かと思います。(添付写真で選択している部分のフォルダ名が違う)
|
168
|
-
|
169
|
-
let filePath = imageItemList[i].imageURL
|
170
|
-
print("filePath: ", filePath)
|
171
|
-
|
172
|
-
if FileManager.default.fileExists(atPath: filePath) {
|
173
|
-
if let image = UIImage(contentsOfFile: filePath) {
|
174
|
-
imageView.image = image
|
175
|
-
self.bgView.insertSubview(imageView, belowSubview: dateLabel)
|
176
|
-
} else {
|
177
|
-
print("Failed to load image from path: \(filePath)")
|
178
|
-
}
|
179
|
-
} else {
|
180
|
-
|
131
|
+
該当部分のフォルダ名は実行するたびに変わってしまっているため、写真が指定されたパスに存在しないとなってしまっています。
|
181
|
-
}
|
182
|
-
}
|
183
|
-
|
132
|
+
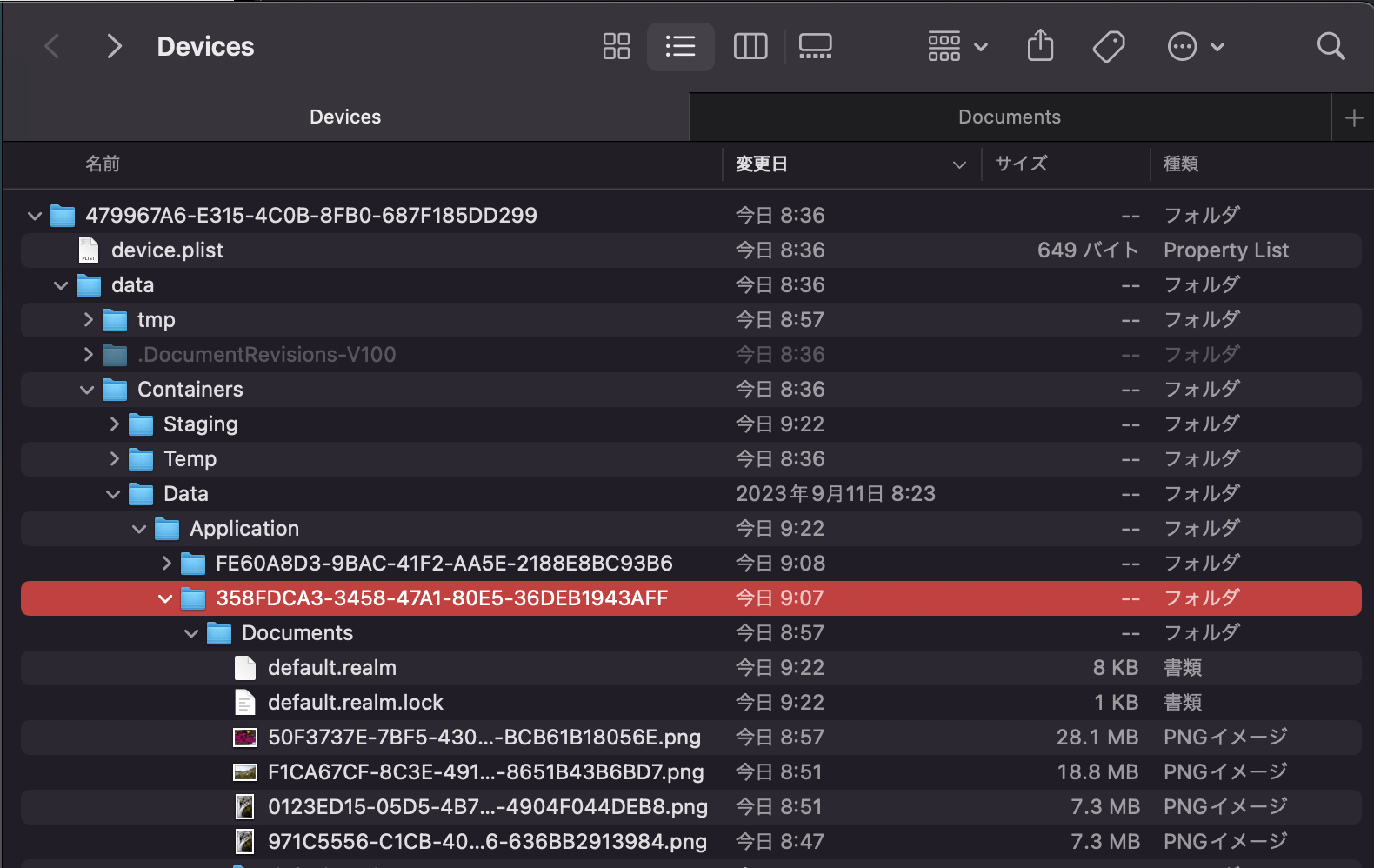
|
184
133
|
|
185
134
|
### 補足
|
186
135
|
参考にしたサイトは以下です。
|
2
書式の変更
test
CHANGED
File without changes
|
test
CHANGED
@@ -159,7 +159,7 @@
|
|
159
159
|
filePath: /...中略.../479967A6-E315-4C0B-8FB0-687F185DD299/data/Containers/Data/Application/4B03C2DC-2C13-421D-8F0D-41EBAF5DCA3E/Documents
|
160
160
|
```
|
161
161
|
|
162
|
-
表示する時のコードを以下のように変えたところ、
|
162
|
+
表示する時のコードを以下のように変えたところ、File does not exist at path:... と表示されました。
|
163
163
|
```Swift
|
164
164
|
for i in 0..<imageItemList.count {
|
165
165
|
let imageView = UIImageView()
|
1
書式の改善
test
CHANGED
File without changes
|
test
CHANGED
@@ -184,6 +184,6 @@
|
|
184
184
|
|
185
185
|
### 補足
|
186
186
|
参考にしたサイトは以下です。
|
187
|
-
- [Swift Realm- リストで画像の保存・表示・削除(https://zenn.dev/kazushino/articles/90bafb36f07fb2)
|
187
|
+
- [Swift Realm- リストで画像の保存・表示・削除](https://zenn.dev/kazushino/articles/90bafb36f07fb2)
|
188
188
|
- [【Swift】Realmを使って画像データの保存と表示を実装する](https://qiita.com/Harx02663971/items/0f18c53a9aa948fbb8cd)
|
189
189
|
- [ファイルPathとファイルURL](https://galakutaapp.blogspot.com/2017/11/pathurl.html)
|