質問編集履歴
5
コードの修正
title
CHANGED
File without changes
|
body
CHANGED
@@ -187,150 +187,8 @@
|
|
187
187
|
|
188
188
|
|
189
189
|
|
190
|
-
|
190
|
+
|
191
|
-
/// 買い物リストをcsvファイルから読み込む
|
192
|
-
/// </summary>
|
193
|
-
/// <param name="sender"></param>
|
194
|
-
/// <param name="e"></param>
|
195
|
-
private void LoadingButton_Click(object sender, EventArgs e)
|
196
|
-
{
|
197
|
-
var dlg = new OpenFileDialog()
|
198
|
-
{
|
199
|
-
Title = "ファイルの選択",
|
200
|
-
CheckFileExists = true,
|
201
|
-
RestoreDirectory = true,
|
202
|
-
InitialDirectory = @"C:\",
|
203
|
-
FileName = @"ItemList.csv",
|
204
|
-
Filter = "CSVファイル|*.csv|すべてのファイル|*.*"
|
205
|
-
};
|
206
191
|
|
207
|
-
if (dlg.ShowDialog() == DialogResult.OK)
|
208
|
-
{
|
209
|
-
OleDbConnection connection;
|
210
|
-
OleDbCommand command;
|
211
|
-
OleDbDataAdapter adapter;
|
212
|
-
|
213
|
-
DataSet dataset = new DataSet();
|
214
|
-
DataGrid datagrid = new DataGrid();
|
215
|
-
|
216
|
-
connection = new OleDbConnection("Provider=Microsoft.Jet.OLEDB.4.0; Data Source=" + Path.GetDirectoryName(dlg.FileName) + "\\; Extended Properties=\"Text;HDR=YES;FMT=Delimited\"");
|
217
|
-
command = new OleDbCommand("SELECT [日付], [商品], [個数], [値段] FROM [" + Path.GetFileName(dlg.FileName) + "]", connection);
|
218
|
-
|
219
|
-
dataset.Clear();
|
220
|
-
|
221
|
-
adapter = new OleDbDataAdapter(command);
|
222
|
-
adapter.Fill(dataset);
|
223
|
-
|
224
|
-
|
225
|
-
|
226
|
-
DataTable table = new DataTable();
|
227
|
-
|
228
|
-
table.Columns.Add("日付");
|
229
|
-
table.Columns.Add("商品");
|
230
|
-
table.Columns.Add("個数");
|
231
|
-
table.Columns.Add("値段");
|
232
|
-
|
233
|
-
|
234
|
-
|
235
|
-
for (int line = 0; line < dataset.Tables[0].Rows.Count; line++)
|
236
|
-
{
|
237
|
-
DataRow data = dataset.Tables[0].Rows[line];
|
238
|
-
Object[] new_row = new Object[]
|
239
|
-
{
|
240
|
-
String.Format(@"{0} ({1})", data["日付"], data["商品"]), data["個数"], data["値段"]
|
241
|
-
};
|
242
|
-
table.Rows.Add(new_row);
|
243
|
-
|
244
|
-
}
|
245
|
-
|
246
|
-
datagrid.DataSource = table;
|
247
|
-
}
|
248
|
-
|
249
|
-
else
|
250
|
-
{
|
251
|
-
MessageBox.Show("ファイルが見つかりません");
|
252
|
-
return;
|
253
|
-
}
|
254
|
-
}
|
255
|
-
|
256
|
-
|
257
|
-
/// <summary>
|
258
|
-
/// 買い物リストを削除する
|
259
|
-
/// </summary>
|
260
|
-
/// <param name="sender"></param>
|
261
|
-
/// <param name="e"></param>
|
262
|
-
private void DeletionButton_Click(object sender, EventArgs e)
|
263
|
-
{
|
264
|
-
foreach (DataGridViewRow r in ItemListDataGridView.SelectedRows)
|
265
|
-
{
|
266
|
-
if (!r.IsNewRow)
|
267
|
-
{
|
268
|
-
ItemListDataGridView.Rows.Remove(r);
|
269
|
-
}
|
270
|
-
}
|
271
|
-
|
272
|
-
}
|
273
|
-
|
274
|
-
|
275
|
-
/// <summary>
|
276
|
-
/// 買い物リストを編集する
|
277
|
-
/// </summary>
|
278
|
-
/// <param name="sender"></param>
|
279
|
-
/// <param name="e"></param>
|
280
|
-
private void EditingButton_Click(object sender, EventArgs e)
|
281
|
-
{
|
282
|
-
|
283
|
-
int count = (int)CountNumericUpDown.Value;
|
284
|
-
int ListPrice = (int)ListPriceNumericUpDown.Value;
|
285
|
-
int price = ListPrice * count;
|
286
|
-
string date = dateTimePicker.Value.ToLongDateString();
|
287
|
-
string itemLine = ItemNameComboBox.Text;
|
288
|
-
|
289
|
-
if (ItemListDataGridView.CurrentCell == ItemListDataGridView[0, 0])
|
290
|
-
{
|
291
|
-
foreach (DataGridViewRow r in ItemListDataGridView.SelectedRows)
|
292
|
-
{
|
293
|
-
if (!r.IsNewRow)
|
294
|
-
{
|
295
|
-
ItemListDataGridView.Rows.Remove(r);
|
296
|
-
ItemListDataGridView.Rows.Add(date, itemLine, count, price);
|
297
|
-
}
|
298
|
-
}
|
299
|
-
|
300
|
-
}
|
301
|
-
|
302
|
-
else
|
303
|
-
{
|
304
|
-
foreach (DataGridViewRow r in ItemListDataGridView.SelectedRows)
|
305
|
-
{
|
306
|
-
if (!r.IsNewRow)
|
307
|
-
{
|
308
|
-
ItemListDataGridView.Rows.Remove(r);
|
309
|
-
ItemListDataGridView.Rows.Add(" ", itemLine, count, price);
|
310
|
-
}
|
311
|
-
}
|
312
|
-
|
313
|
-
|
314
|
-
}
|
315
|
-
|
316
|
-
}
|
317
|
-
|
318
|
-
|
319
|
-
/// <summary>
|
320
|
-
/// アプリケーションを終了する
|
321
|
-
/// </summary>
|
322
|
-
/// <param name="sender"></param>
|
323
|
-
/// <param name="e"></param>
|
324
|
-
private void CloseButton_Click(object sender, EventArgs e)
|
325
|
-
{
|
326
|
-
Application.Exit();
|
327
|
-
}
|
328
|
-
|
329
|
-
}
|
330
|
-
|
331
|
-
|
332
|
-
}
|
333
|
-
|
334
192
|
```
|
335
193
|
|
336
194
|
|
4
コードの編集
title
CHANGED
File without changes
|
body
CHANGED
@@ -22,9 +22,49 @@
|
|
22
22
|
ソースコード
|
23
23
|
|
24
24
|
```
|
25
|
+
using System;
|
26
|
+
using System.IO;
|
27
|
+
using System.Windows.Forms;
|
28
|
+
using System.Data;
|
29
|
+
using System.Text;
|
30
|
+
using System.Collections.Generic;
|
31
|
+
using System.Security.Cryptography;
|
32
|
+
using System.Data.OleDb;
|
33
|
+
using System.Runtime.InteropServices.ComTypes;
|
34
|
+
using System.Windows.Forms.VisualStyles;
|
35
|
+
|
36
|
+
namespace recruitPGwork
|
37
|
+
{
|
38
|
+
public partial class LunchBoxMemoForm : Form
|
39
|
+
{
|
40
|
+
private const string NewLine = "\r\n";
|
41
|
+
private readonly DataTable dt;
|
42
|
+
|
43
|
+
/// <summary>
|
25
|
-
|
44
|
+
/// コンストラクタ
|
26
|
-
|
45
|
+
/// </summary>
|
46
|
+
public LunchBoxMemoForm()
|
27
47
|
{
|
48
|
+
InitializeComponent();
|
49
|
+
|
50
|
+
DataTable dt = new DataTable();
|
51
|
+
dt.TableName = "ItemList";
|
52
|
+
|
53
|
+
dt.Columns.Add("日付", typeof(string));
|
54
|
+
dt.Columns.Add("商品", typeof(string));
|
55
|
+
dt.Columns.Add("個数", typeof(int));
|
56
|
+
dt.Columns.Add("値段", typeof(int));
|
57
|
+
|
58
|
+
ItemListDataGridView.DataSource = dt;
|
59
|
+
}
|
60
|
+
|
61
|
+
|
62
|
+
// 合計金額を求める
|
63
|
+
private void CalculateButton_Click(object sender, EventArgs e)
|
64
|
+
{
|
65
|
+
//DataTable作成
|
66
|
+
|
67
|
+
|
28
68
|
// 品物が選択されているか判定する
|
29
69
|
if (string.IsNullOrWhiteSpace(ItemNameComboBox.Text))
|
30
70
|
{
|
@@ -53,99 +93,244 @@
|
|
53
93
|
|
54
94
|
|
55
95
|
// 今回の金額
|
56
|
-
|
96
|
+
var price = ListPrice * count;
|
57
97
|
|
58
98
|
|
59
99
|
// 買い物リスト用の文字列を作成する
|
60
|
-
|
100
|
+
var date = dateTimePicker.Value.ToLongDateString();
|
61
|
-
|
101
|
+
var item = ItemNameComboBox.Text;
|
62
102
|
|
63
103
|
|
64
|
-
//DataTable作成
|
65
|
-
DataTable dt = new DataTable();
|
66
|
-
dt.TableName = "ItemList";
|
67
104
|
|
68
|
-
dt.Columns.Add("日付", typeof(string));
|
69
|
-
dt.Columns.Add("商品", typeof(string));
|
70
|
-
dt.Columns.Add("個数", typeof(int));
|
71
|
-
dt.Columns.Add("値段", typeof(int));
|
72
|
-
|
73
|
-
|
74
|
-
|
75
105
|
// 買い物リストに追加する
|
76
106
|
|
77
|
-
if (
|
107
|
+
if (0 == dt.Rows.Count)
|
78
108
|
{
|
79
|
-
|
80
109
|
DataRow row = dt.NewRow();
|
81
110
|
row["日付"] = date;
|
82
111
|
row["商品"] = item;
|
83
112
|
row["個数"] = count;
|
84
113
|
row["値段"] = price;
|
85
|
-
|
86
114
|
dt.Rows.Add(row);
|
87
|
-
|
88
|
-
ItemListDataGridView.DataSource = dt;
|
89
115
|
}
|
90
116
|
|
91
|
-
|
117
|
+
|
92
|
-
if (
|
118
|
+
else if (dt.Rows[0].Field<string>("日付") == date)
|
93
119
|
{
|
94
|
-
|
120
|
+
date = " ";
|
95
121
|
|
96
122
|
DataRow row = dt.NewRow();
|
97
123
|
row["日付"] = date;
|
98
124
|
row["商品"] = item;
|
99
125
|
row["個数"] = count;
|
100
126
|
row["値段"] = price;
|
101
|
-
|
102
127
|
dt.Rows.Add(row);
|
103
|
-
|
104
|
-
ItemListDataGridView.DataSource = dt;
|
105
128
|
}
|
106
129
|
|
107
130
|
else
|
108
131
|
{
|
132
|
+
dt.Clear();
|
133
|
+
|
109
134
|
DataRow row = dt.NewRow();
|
110
|
-
row["日付"] =
|
135
|
+
row["日付"] = date;
|
111
136
|
row["商品"] = item;
|
112
137
|
row["個数"] = count;
|
113
138
|
row["値段"] = price;
|
114
|
-
|
115
139
|
dt.Rows.Add(row);
|
116
|
-
|
117
|
-
ItemListDataGridView.DataSource = dt;
|
118
140
|
}
|
141
|
+
|
142
|
+
|
143
|
+
// グラフ用の文字列を作成する
|
144
|
+
string graphLine = new string('■', count);
|
119
145
|
|
120
146
|
|
121
|
-
// グラフ
|
147
|
+
// グラフを表示する
|
148
|
+
GraphTextBox.Text += graphLine + Environment.NewLine;
|
149
|
+
|
150
|
+
|
151
|
+
// 合計金額を表示する
|
152
|
+
_ = int.TryParse(TotalTextBox.Text, out int total);
|
122
|
-
|
153
|
+
TotalTextBox.Text = $"{total + price}";
|
154
|
+
|
155
|
+
}
|
156
|
+
|
157
|
+
|
158
|
+
|
123
|
-
|
159
|
+
/// <summary>
|
160
|
+
/// 買い物リストをcsvファイルとして保存する
|
161
|
+
/// </summary>
|
162
|
+
/// <param name="sender"></param>
|
163
|
+
/// <param name="e"></param>
|
164
|
+
private void SaveButton_Click(object sender, EventArgs e)
|
165
|
+
{
|
166
|
+
SaveFileDialog dlg = new SaveFileDialog
|
124
167
|
{
|
168
|
+
Title = "ファイルを保存する",
|
125
|
-
|
169
|
+
FileName = @"ItemList.csv",
|
170
|
+
Filter = "CSV(カンマ区切り)|*.csv"
|
171
|
+
};
|
172
|
+
|
173
|
+
if (dlg.ShowDialog() == DialogResult.Cancel)
|
174
|
+
{
|
175
|
+
MessageBox.Show("キャンセルされました");
|
176
|
+
return;
|
126
177
|
}
|
127
178
|
|
179
|
+
|
180
|
+
using (StreamWriter writer = new StreamWriter(dlg.FileName, false, Encoding.GetEncoding("shift_jis")))
|
181
|
+
foreach (DataRow row in dt.Rows)
|
182
|
+
{
|
183
|
+
writer.WriteLine(string.Join(",", row.ItemArray));
|
184
|
+
}
|
185
|
+
}
|
186
|
+
|
187
|
+
|
188
|
+
|
189
|
+
|
128
|
-
|
190
|
+
/// <summary>
|
191
|
+
/// 買い物リストをcsvファイルから読み込む
|
192
|
+
/// </summary>
|
193
|
+
/// <param name="sender"></param>
|
194
|
+
/// <param name="e"></param>
|
129
|
-
|
195
|
+
private void LoadingButton_Click(object sender, EventArgs e)
|
196
|
+
{
|
197
|
+
var dlg = new OpenFileDialog()
|
130
198
|
{
|
199
|
+
Title = "ファイルの選択",
|
200
|
+
CheckFileExists = true,
|
201
|
+
RestoreDirectory = true,
|
202
|
+
InitialDirectory = @"C:\",
|
203
|
+
FileName = @"ItemList.csv",
|
204
|
+
Filter = "CSVファイル|*.csv|すべてのファイル|*.*"
|
205
|
+
};
|
206
|
+
|
207
|
+
if (dlg.ShowDialog() == DialogResult.OK)
|
208
|
+
{
|
209
|
+
OleDbConnection connection;
|
210
|
+
OleDbCommand command;
|
211
|
+
OleDbDataAdapter adapter;
|
212
|
+
|
131
|
-
|
213
|
+
DataSet dataset = new DataSet();
|
214
|
+
DataGrid datagrid = new DataGrid();
|
215
|
+
|
216
|
+
connection = new OleDbConnection("Provider=Microsoft.Jet.OLEDB.4.0; Data Source=" + Path.GetDirectoryName(dlg.FileName) + "\\; Extended Properties=\"Text;HDR=YES;FMT=Delimited\"");
|
217
|
+
command = new OleDbCommand("SELECT [日付], [商品], [個数], [値段] FROM [" + Path.GetFileName(dlg.FileName) + "]", connection);
|
218
|
+
|
219
|
+
dataset.Clear();
|
220
|
+
|
221
|
+
adapter = new OleDbDataAdapter(command);
|
222
|
+
adapter.Fill(dataset);
|
223
|
+
|
224
|
+
|
225
|
+
|
226
|
+
DataTable table = new DataTable();
|
227
|
+
|
228
|
+
table.Columns.Add("日付");
|
229
|
+
table.Columns.Add("商品");
|
230
|
+
table.Columns.Add("個数");
|
231
|
+
table.Columns.Add("値段");
|
232
|
+
|
233
|
+
|
234
|
+
|
235
|
+
for (int line = 0; line < dataset.Tables[0].Rows.Count; line++)
|
236
|
+
{
|
237
|
+
DataRow data = dataset.Tables[0].Rows[line];
|
238
|
+
Object[] new_row = new Object[]
|
239
|
+
{
|
240
|
+
String.Format(@"{0} ({1})", data["日付"], data["商品"]), data["個数"], data["値段"]
|
241
|
+
};
|
242
|
+
table.Rows.Add(new_row);
|
243
|
+
|
244
|
+
}
|
245
|
+
|
246
|
+
datagrid.DataSource = table;
|
132
247
|
}
|
248
|
+
|
133
249
|
else
|
134
250
|
{
|
135
|
-
|
251
|
+
MessageBox.Show("ファイルが見つかりません");
|
252
|
+
return;
|
136
253
|
}
|
254
|
+
}
|
137
255
|
|
256
|
+
|
257
|
+
/// <summary>
|
138
|
-
|
258
|
+
/// 買い物リストを削除する
|
259
|
+
/// </summary>
|
260
|
+
/// <param name="sender"></param>
|
261
|
+
/// <param name="e"></param>
|
139
|
-
|
262
|
+
private void DeletionButton_Click(object sender, EventArgs e)
|
263
|
+
{
|
264
|
+
foreach (DataGridViewRow r in ItemListDataGridView.SelectedRows)
|
140
265
|
{
|
141
|
-
|
266
|
+
if (!r.IsNewRow)
|
267
|
+
{
|
142
|
-
|
268
|
+
ItemListDataGridView.Rows.Remove(r);
|
269
|
+
}
|
143
270
|
}
|
271
|
+
|
272
|
+
}
|
273
|
+
|
274
|
+
|
275
|
+
/// <summary>
|
276
|
+
/// 買い物リストを編集する
|
277
|
+
/// </summary>
|
278
|
+
/// <param name="sender"></param>
|
279
|
+
/// <param name="e"></param>
|
280
|
+
private void EditingButton_Click(object sender, EventArgs e)
|
281
|
+
{
|
282
|
+
|
283
|
+
int count = (int)CountNumericUpDown.Value;
|
284
|
+
int ListPrice = (int)ListPriceNumericUpDown.Value;
|
285
|
+
int price = ListPrice * count;
|
286
|
+
string date = dateTimePicker.Value.ToLongDateString();
|
287
|
+
string itemLine = ItemNameComboBox.Text;
|
288
|
+
|
289
|
+
if (ItemListDataGridView.CurrentCell == ItemListDataGridView[0, 0])
|
290
|
+
{
|
291
|
+
foreach (DataGridViewRow r in ItemListDataGridView.SelectedRows)
|
292
|
+
{
|
293
|
+
if (!r.IsNewRow)
|
294
|
+
{
|
295
|
+
ItemListDataGridView.Rows.Remove(r);
|
296
|
+
ItemListDataGridView.Rows.Add(date, itemLine, count, price);
|
297
|
+
}
|
298
|
+
}
|
299
|
+
|
300
|
+
}
|
301
|
+
|
144
302
|
else
|
145
303
|
{
|
304
|
+
foreach (DataGridViewRow r in ItemListDataGridView.SelectedRows)
|
305
|
+
{
|
306
|
+
if (!r.IsNewRow)
|
307
|
+
{
|
146
|
-
|
308
|
+
ItemListDataGridView.Rows.Remove(r);
|
309
|
+
ItemListDataGridView.Rows.Add(" ", itemLine, count, price);
|
310
|
+
}
|
311
|
+
}
|
312
|
+
|
313
|
+
|
147
314
|
}
|
315
|
+
|
148
316
|
}
|
317
|
+
|
318
|
+
|
319
|
+
/// <summary>
|
320
|
+
/// アプリケーションを終了する
|
321
|
+
/// </summary>
|
322
|
+
/// <param name="sender"></param>
|
323
|
+
/// <param name="e"></param>
|
324
|
+
private void CloseButton_Click(object sender, EventArgs e)
|
325
|
+
{
|
326
|
+
Application.Exit();
|
327
|
+
}
|
328
|
+
|
329
|
+
}
|
330
|
+
|
331
|
+
|
332
|
+
}
|
333
|
+
|
149
334
|
```
|
150
335
|
|
151
336
|
|
3
画像の挿入
title
CHANGED
File without changes
|
body
CHANGED
@@ -13,9 +13,12 @@
|
|
13
13
|
現状では、条件分の入力方法が分からないのと、ボタンクリックしても何も起きません。
|
14
14
|
エラーメッセージはありません。
|
15
15
|
|
16
|
+
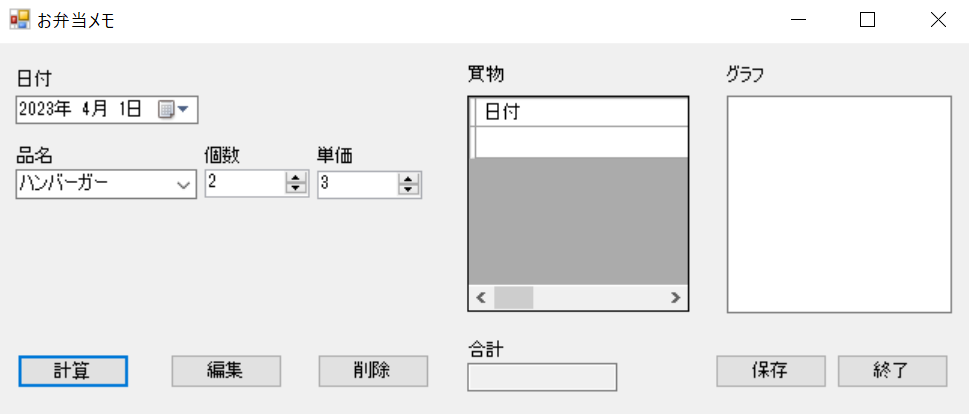
|
17
|
+
|
16
18
|
### 該当のソースコード
|
17
19
|
VIsualStudio2022 C# .NET6 フォームデザイナーを用いています。
|
18
20
|
|
21
|
+
|
19
22
|
ソースコード
|
20
23
|
|
21
24
|
```
|
2
ソースコード編集
title
CHANGED
File without changes
|
body
CHANGED
@@ -18,6 +18,7 @@
|
|
18
18
|
|
19
19
|
ソースコード
|
20
20
|
|
21
|
+
```
|
21
22
|
// ボタンクリックする
|
22
23
|
private void CalculateButton_Click(object sender, EventArgs e)
|
23
24
|
{
|
@@ -142,7 +143,7 @@
|
|
142
143
|
TotalTextBox.Text = price.ToString();
|
143
144
|
}
|
144
145
|
}
|
146
|
+
```
|
145
147
|
|
146
|
-
|
147
148
|
|
148
149
|
|
1
誤字、使用
title
CHANGED
@@ -1,1 +1,1 @@
|
|
1
|
-
VIsualStudio2022でDataTableと
|
1
|
+
VIsualStudio2022でDataTableとDataGridViewのバインドをしたいです。
|
body
CHANGED
@@ -1,5 +1,5 @@
|
|
1
1
|
### 実現したいこと
|
2
|
-
①DataTableの
|
2
|
+
①DataTableのDataGridViewの相互でバインドをする
|
3
3
|
②ボタンクリックでコンボボックス等の情報をDataTableにいれる。
|
4
4
|
③この時、DataTable.Row[0][0]が空白であれば、4列入力する。入れようとしている文字列と同じであれば[1][1]から3列いれる。入れようと文字列と違うのであれば、DataTableをクリアして新しくDataTable作りなおす。
|
5
5
|
|
@@ -14,10 +14,11 @@
|
|
14
14
|
エラーメッセージはありません。
|
15
15
|
|
16
16
|
### 該当のソースコード
|
17
|
-
VIsualStudio2022 C# フォームデザイナーを用いています。
|
17
|
+
VIsualStudio2022 C# .NET6 フォームデザイナーを用いています。
|
18
18
|
|
19
19
|
ソースコード
|
20
20
|
|
21
|
+
// ボタンクリックする
|
21
22
|
private void CalculateButton_Click(object sender, EventArgs e)
|
22
23
|
{
|
23
24
|
// 品物が選択されているか判定する
|
@@ -142,3 +143,6 @@
|
|
142
143
|
}
|
143
144
|
}
|
144
145
|
|
146
|
+
|
147
|
+
|
148
|
+
|