データは episteme さんの「質問へのコメント」から頂きました。
java
1import java.awt.event.ItemEvent;
2import java.util.HashMap;
3import java.util.Map;
4
5import javax.swing.*;
6
7public class ComboBoxTest extends JFrame {
8 public static void main(String[] args) {
9 SwingUtilities.invokeLater(() -> new ComboBoxTest().setVisible(true));
10 }
11
12 ComboBoxTest() {
13 super("ComboBoxTest");
14 setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
15 setSize(320, 200);
16 setLocationRelativeTo(null);
17
18 Map<String,String[]> map = new HashMap<String,String[]>();
19 map.put("くだもの", new String[]{"りんご","みかん","もも"});
20 map.put("やさい", new String[]{"たまねぎ","にんじん","じゃがいも"});
21
22 JComboBox<String> combobox1 = new JComboBox<String>(new DefaultComboBoxModel<String>(map.keySet().toArray(new String[map.size()])));
23 JComboBox<String> combobox2 = new JComboBox<String>();
24
25 getContentPane().setLayout(new BoxLayout(getContentPane(), BoxLayout.Y_AXIS));
26 add(combobox1);
27 add(combobox2);
28
29 combobox1.addItemListener(e -> {
30 if(e.getStateChange() == ItemEvent.SELECTED) combobox2.setModel(new DefaultComboBoxModel<String>(map.get(e.getItem())));
31 });
32 combobox1.setSelectedIndex(-1); //選択無し
33 combobox1.setSelectedIndex(0); //↑ が無いと変化無しでイベントが通知されない
34 }
35}
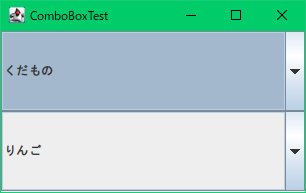