主キー項目は自動生成されない と書いてあるのですが、
MVC5 でもその通りだと思いましたが、念のため自分の環境の Visual Studio Community 2015 の MVC5 で確認してみました。やはり @IT の記事の通りの結果(View に Isbn 関係のコードは含まれない)となります。
質問者さんのケースで何故違う結果になったのかは分かりませんが、自動生成されたのならそれはそれで問題ないので、そのまま使えば良いと思いますが。
参考までに自分の環境での結果を書いておきます。
Model
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.ComponentModel.DataAnnotations;
using System.Globalization;
using System.Web.Mvc;
namespace Mvc5App.Models
{
public class Book
{
[Key]
[Display(Name = "ISBNコード")]
[Required(ErrorMessage = "{0}は必須です。")]
[RegularExpression("[0-9]{3}-[0-9]{1}-[0-9]{3,5}-[0-9]{3,5}-[0-9A-Z]{1}",
ErrorMessage = "{0}はISBNの形式で入力してください。")]
public string Isbn { get; set; }
[Display(Name = "書名")]
[Required(ErrorMessage = "{0}は必須です。")]
[StringLength(100, ErrorMessage = "{0}は{1}文字以内で入力してください。")]
public string Title { get; set; }
[Display(Name = "価格")]
[Range(100, 10000, ErrorMessage = "{0}は{1}~{2}の間で入力してください。")]
public int? Price { get; set; }
[Display(Name = "出版社")]
[StringLength(30, ErrorMessage = "{0}は{1}文字以内で入力してください。")]
[InArray("翔泳社,技術評論社,秀和システム,毎日コミュニケーションズ,日経BP社,インプレスジャパン")]
public string Publish { get; set; }
[Display(Name = "刊行日")]
[Required(ErrorMessage = "{0}は必須です。")]
public DateTime Published { get; set; }
public virtual ICollection<Review> Reviews { get; set; }
}
public class Review
{
public int ReviewId { get; set; }
public string Title { get; set; }
public string Body { get; set; }
public DateTime CreatedAt { get; set; }
}
// ・・・InArrayAttribute 関係のコード(省略)・・・
}
Controller / Action Method
Create2 を新たに追加しそれからスキャフォールディング機能を使って View を自動生成
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
using Mvc5App.Models;
namespace Mvc5App.Controllers
{
public class BookController : Controller
{
// ・・・既存の Create のコード(省略)・・・
// 入力フォームを生成するためのCreateアクション
public ActionResult Create2()
{
return View();
}
// [Create]ボタンをクリックしたときに呼び出されるCreateアクション
[HttpPost]
public ActionResult Create2(Book book)
{
if (ModelState.IsValid)
{
//db.Books.Add(book);
//db.SaveChanges();
return RedirectToAction("Index", "Home");
}
return View(book);
}
}
}
Add View ダイアログの設定
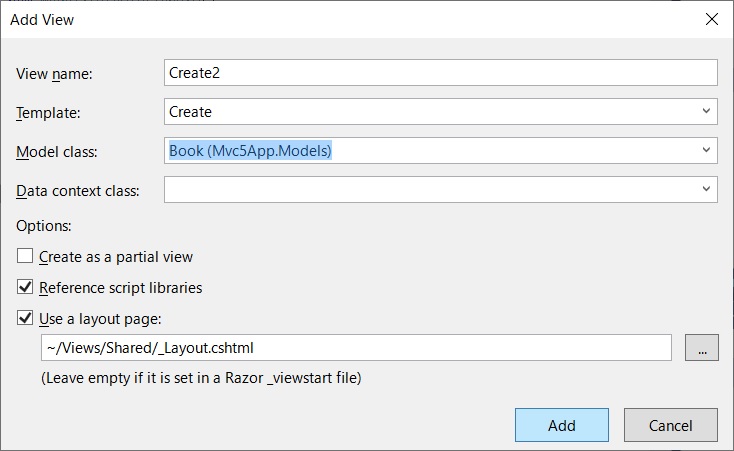
View
自動生成されたもの。@IT の記事に書いてある通り、Isbn 関係のコードは含まれない。
@model Mvc5App.Models.Book
@{
ViewBag.Title = "Create2";
Layout = "~/Views/Shared/_Layout.cshtml";
}
<h2>Create2</h2>
@using (Html.BeginForm())
{
@Html.AntiForgeryToken()
<div class="form-horizontal">
<h4>Book</h4>
<hr />
@Html.ValidationSummary(true, "", new { @class = "text-danger" })
<div class="form-group">
@Html.LabelFor(model => model.Title, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Title, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.Title, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.Price, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Price, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.Price, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.Publish, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Publish, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.Publish, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.Published, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Published, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.Published, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
<div class="col-md-offset-2 col-md-10">
<input type="submit" value="Create" class="btn btn-default" />
</div>
</div>
</div>
}
<div>
@Html.ActionLink("Back to List", "Index")
</div>
@section Scripts {
@Scripts.Render("~/bundles/jqueryval")
}
実行結果
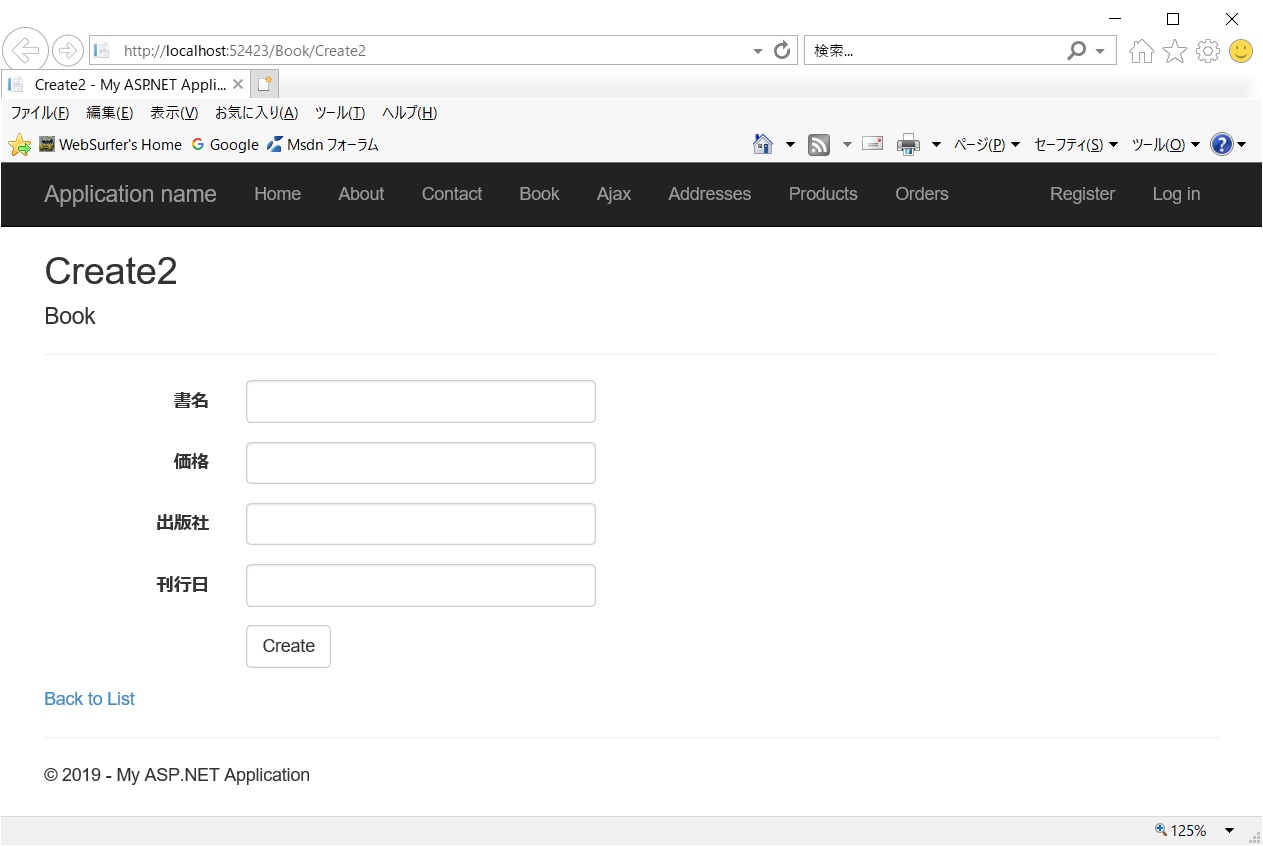