回答編集履歴
2
修正
answer
CHANGED
@@ -3,6 +3,7 @@
|
|
3
3
|
|
4
4
|
---
|
5
5
|
|
6
|
+
MainActivity.java
|
6
7
|
```java
|
7
8
|
import androidx.appcompat.app.AppCompatActivity;
|
8
9
|
|
1
追加
answer
CHANGED
@@ -1,2 +1,88 @@
|
|
1
1
|
考え方としては以下のようなことになります。
|
2
|
-
[AndroidのListViewにて、List上に配置したボタンのイベントを取得したい。](https://teratail.com/questions/117534)
|
2
|
+
[AndroidのListViewにて、List上に配置したボタンのイベントを取得したい。](https://teratail.com/questions/117534)
|
3
|
+
|
4
|
+
---
|
5
|
+
|
6
|
+
```java
|
7
|
+
import androidx.appcompat.app.AppCompatActivity;
|
8
|
+
|
9
|
+
import android.os.Bundle;
|
10
|
+
import android.util.Log;
|
11
|
+
import android.view.*;
|
12
|
+
import android.widget.*;
|
13
|
+
|
14
|
+
import java.util.*;
|
15
|
+
|
16
|
+
public class MainActivity extends AppCompatActivity {
|
17
|
+
|
18
|
+
@Override
|
19
|
+
protected void onCreate(Bundle savedInstanceState) {
|
20
|
+
super.onCreate(savedInstanceState);
|
21
|
+
setContentView(R.layout.activity_main);
|
22
|
+
|
23
|
+
ListView listview = findViewById(R.id.listview);
|
24
|
+
|
25
|
+
View header = getLayoutInflater().inflate(R.layout.list_header, listview, false);
|
26
|
+
header.findViewById(R.id.title).setOnClickListener(v -> Log.d("MainActivity ***", "click: header title"));
|
27
|
+
header.findViewById(R.id.updateDate).setOnClickListener(v -> Log.d("MainActivity ***", "click: header updateDate"));
|
28
|
+
listview.addHeaderView(header);
|
29
|
+
|
30
|
+
List<Map<String,String>> data = new ArrayList<Map<String,String>>();
|
31
|
+
Map<String,String> value = new HashMap<String,String>();
|
32
|
+
value.put("text1", "data1");
|
33
|
+
value.put("text2", "data2");
|
34
|
+
data.add(value);
|
35
|
+
listview.setAdapter(new SimpleAdapter(this, data, android.R.layout.simple_list_item_2, new String[]{"text1","text2"}, new int[]{android.R.id.text1,android.R.id.text2}));
|
36
|
+
}
|
37
|
+
}
|
38
|
+
```
|
39
|
+
res/layout/activity_main.xml
|
40
|
+
```xml
|
41
|
+
<?xml version="1.0" encoding="utf-8"?>
|
42
|
+
<ListView
|
43
|
+
xmlns:android="http://schemas.android.com/apk/res/android"
|
44
|
+
xmlns:tools="http://schemas.android.com/tools"
|
45
|
+
android:id="@+id/listview"
|
46
|
+
android:layout_width="match_parent"
|
47
|
+
android:layout_height="match_parent"
|
48
|
+
tools:context=".MainActivity" />
|
49
|
+
```
|
50
|
+
res/layout/list_header.xml
|
51
|
+
```xml
|
52
|
+
<?xml version="1.0" encoding="utf-8"?>
|
53
|
+
<androidx.constraintlayout.widget.ConstraintLayout
|
54
|
+
xmlns:android="http://schemas.android.com/apk/res/android"
|
55
|
+
android:layout_width="match_parent"
|
56
|
+
android:layout_height="wrap_content"
|
57
|
+
xmlns:app="http://schemas.android.com/apk/res-auto">
|
58
|
+
<TextView
|
59
|
+
android:id="@+id/title"
|
60
|
+
android:layout_width="0dp"
|
61
|
+
android:layout_height="wrap_content"
|
62
|
+
android:textSize="20sp"
|
63
|
+
android:text="タイトル"
|
64
|
+
android:clickable="true"
|
65
|
+
app:layout_constraintHorizontal_weight="6"
|
66
|
+
app:layout_constraintEnd_toStartOf="@id/updateDate"
|
67
|
+
app:layout_constraintStart_toStartOf="parent"
|
68
|
+
app:layout_constraintTop_toTopOf="parent" />
|
69
|
+
<TextView
|
70
|
+
android:id="@+id/updateDate"
|
71
|
+
android:layout_width="0dp"
|
72
|
+
android:layout_height="wrap_content"
|
73
|
+
android:textSize="20sp"
|
74
|
+
android:text="更新日時"
|
75
|
+
android:clickable="true"
|
76
|
+
app:layout_constraintHorizontal_weight="4"
|
77
|
+
app:layout_constraintEnd_toEndOf="parent"
|
78
|
+
app:layout_constraintStart_toEndOf="@id/title"
|
79
|
+
app:layout_constraintTop_toTopOf="parent" />
|
80
|
+
</androidx.constraintlayout.widget.ConstraintLayout>
|
81
|
+
```
|
82
|
+
実行結果
|
83
|
+
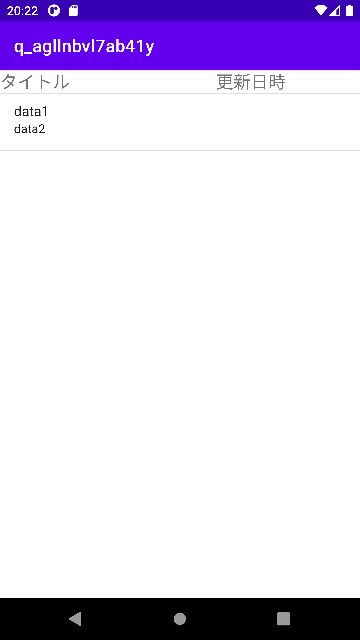
|
84
|
+
"タイトル","更新日時"をクリックした時のログ
|
85
|
+
```plain
|
86
|
+
D/MainActivity ***: click: header title
|
87
|
+
D/MainActivity ***: click: header updateDate
|
88
|
+
```
|