回答編集履歴
2
追記
test
CHANGED
@@ -48,3 +48,188 @@
|
|
48
48
|
}
|
49
49
|
}
|
50
50
|
```
|
51
|
+
|
52
|
+
---
|
53
|
+
|
54
|
+
**【追記】**
|
55
|
+
|
56
|
+
下のコメント欄の 2022/10/08 16:19 の私のコメントで「後で回答欄にサンプルを追記しておきます」と書いた件です。
|
57
|
+
|
58
|
+
元の SQL Server のデータベースは以下の通りです。
|
59
|
+
|
60
|
+
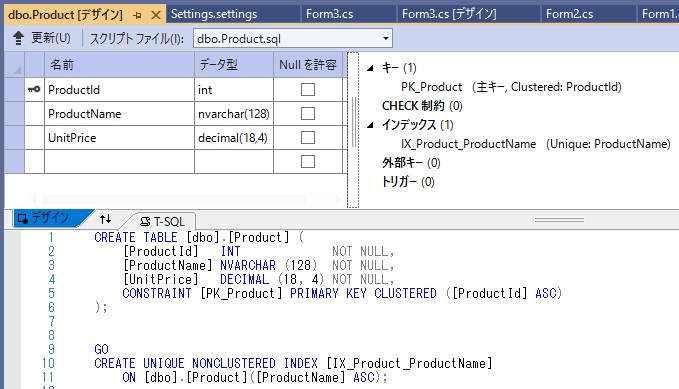
|
61
|
+
|
62
|
+
これをベースにデータソース構成ウィザードを使って型付 DataSet / DataTable を作って、それをデザイン画面で「データソース」ウィンドウから Form にドラッグ&ドロップして作ったものがベースになってます。
|
63
|
+
|
64
|
+
それに手を加えたのが以下のサンプルです。
|
65
|
+
|
66
|
+
```
|
67
|
+
using System;
|
68
|
+
using System.Data;
|
69
|
+
using System.Windows.Forms;
|
70
|
+
|
71
|
+
namespace WindowsFormsPkUnique
|
72
|
+
{
|
73
|
+
public partial class Form3 : Form
|
74
|
+
{
|
75
|
+
public Form3()
|
76
|
+
{
|
77
|
+
InitializeComponent();
|
78
|
+
|
79
|
+
this.productDataGridView.DataError += ProductDataGridView_DataError;
|
80
|
+
|
81
|
+
this.productBindingSource.PositionChanged += ProductBindingSource_PositionChanged;
|
82
|
+
}
|
83
|
+
|
84
|
+
// BindingNavigation の操作、マウスやキーボードを使ったときのポジションの変更に
|
85
|
+
// にしたがって BindingNavigator 上の ToolStripButton の Enabled プロパティの
|
86
|
+
// 設定を変更するには BindingSource.PositionChanged イベントのハンドラで設定
|
87
|
+
private void ProductBindingSource_PositionChanged(object sender, EventArgs e)
|
88
|
+
{
|
89
|
+
int count = productBindingSource.Count;
|
90
|
+
int position = productBindingSource.Position;
|
91
|
+
if (count <= 1)
|
92
|
+
{
|
93
|
+
bindingNavigatorMoveFirstItem.Enabled = false;
|
94
|
+
bindingNavigatorMovePreviousItem.Enabled = false;
|
95
|
+
bindingNavigatorMoveNextItem.Enabled = false;
|
96
|
+
bindingNavigatorMoveLastItem.Enabled = false;
|
97
|
+
if (count == 0)
|
98
|
+
bindingNavigatorDeleteItem.Enabled = false;
|
99
|
+
else
|
100
|
+
bindingNavigatorDeleteItem.Enabled = true;
|
101
|
+
}
|
102
|
+
else
|
103
|
+
{
|
104
|
+
bindingNavigatorDeleteItem.Enabled = true;
|
105
|
+
|
106
|
+
if (position == 0)
|
107
|
+
{
|
108
|
+
bindingNavigatorMoveFirstItem.Enabled = false;
|
109
|
+
bindingNavigatorMovePreviousItem.Enabled = false;
|
110
|
+
bindingNavigatorMoveNextItem.Enabled = true;
|
111
|
+
bindingNavigatorMoveLastItem.Enabled = true;
|
112
|
+
}
|
113
|
+
else if (position == count - 1)
|
114
|
+
{
|
115
|
+
bindingNavigatorMoveFirstItem.Enabled = true;
|
116
|
+
bindingNavigatorMovePreviousItem.Enabled = true;
|
117
|
+
bindingNavigatorMoveNextItem.Enabled = false;
|
118
|
+
bindingNavigatorMoveLastItem.Enabled = false;
|
119
|
+
}
|
120
|
+
else
|
121
|
+
{
|
122
|
+
bindingNavigatorMoveFirstItem.Enabled = true;
|
123
|
+
bindingNavigatorMovePreviousItem.Enabled = true;
|
124
|
+
bindingNavigatorMoveNextItem.Enabled = true;
|
125
|
+
bindingNavigatorMoveLastItem.Enabled = true;
|
126
|
+
}
|
127
|
+
}
|
128
|
+
}
|
129
|
+
|
130
|
+
private void ProductDataGridView_DataError(object sender, DataGridViewDataErrorEventArgs e)
|
131
|
+
{
|
132
|
+
if (e.Exception != null)
|
133
|
+
{
|
134
|
+
MessageBox.Show(this,
|
135
|
+
$"Index {e.RowIndex} の行でエラーが発生しました。\n\n" +
|
136
|
+
$"説明: {e.Exception.Message}",
|
137
|
+
|
138
|
+
"エラーが発生しました",
|
139
|
+
MessageBoxButtons.OK, MessageBoxIcon.Exclamation);
|
140
|
+
}
|
141
|
+
}
|
142
|
+
|
143
|
+
private void productBindingNavigatorSaveItem_Click(object sender, EventArgs e)
|
144
|
+
{
|
145
|
+
this.Validate();
|
146
|
+
this.productBindingSource.EndEdit();
|
147
|
+
this.tableAdapterManager.UpdateAll(this.productDataSet);
|
148
|
+
|
149
|
+
}
|
150
|
+
|
151
|
+
private void Form3_Load(object sender, EventArgs e)
|
152
|
+
{
|
153
|
+
// TODO: このコード行はデータを 'productDataSet.Product' テーブルに読み込みます。
|
154
|
+
// 必要に応じて移動、または削除をしてください。
|
155
|
+
this.productTableAdapter.Fill(this.productDataSet.Product);
|
156
|
+
|
157
|
+
}
|
158
|
+
|
159
|
+
private void bindingNavigatorMoveFirstItem_Click(object sender, EventArgs e)
|
160
|
+
{
|
161
|
+
try
|
162
|
+
{
|
163
|
+
productBindingSource.MoveFirst();
|
164
|
+
}
|
165
|
+
catch (NoNullAllowedException ex)
|
166
|
+
{
|
167
|
+
MessageBox.Show(ex.Message + "\n\nデータを修正するか終了してください。",
|
168
|
+
"「最初に移動」ボタン操作エラー",
|
169
|
+
MessageBoxButtons.OK, MessageBoxIcon.Exclamation);
|
170
|
+
}
|
171
|
+
}
|
172
|
+
|
173
|
+
private void bindingNavigatorMovePreviousItem_Click(object sender, EventArgs e)
|
174
|
+
{
|
175
|
+
try
|
176
|
+
{
|
177
|
+
productBindingSource.MovePrevious();
|
178
|
+
}
|
179
|
+
catch (NoNullAllowedException ex)
|
180
|
+
{
|
181
|
+
MessageBox.Show(ex.Message + "\n\nデータを修正するか終了してください。",
|
182
|
+
"「前に戻る」ボタン操作エラー",
|
183
|
+
MessageBoxButtons.OK, MessageBoxIcon.Exclamation);
|
184
|
+
}
|
185
|
+
}
|
186
|
+
|
187
|
+
private void bindingNavigatorMoveNextItem_Click(object sender, EventArgs e)
|
188
|
+
{
|
189
|
+
try
|
190
|
+
{
|
191
|
+
productBindingSource.MoveNext();
|
192
|
+
}
|
193
|
+
catch (NoNullAllowedException ex)
|
194
|
+
{
|
195
|
+
MessageBox.Show(ex.Message + "\n\nデータを修正するか終了してください。",
|
196
|
+
"「次に移動」ボタン操作エラー",
|
197
|
+
MessageBoxButtons.OK, MessageBoxIcon.Exclamation);
|
198
|
+
}
|
199
|
+
}
|
200
|
+
|
201
|
+
private void bindingNavigatorMoveLastItem_Click(object sender, EventArgs e)
|
202
|
+
{
|
203
|
+
try
|
204
|
+
{
|
205
|
+
productBindingSource.MoveLast();
|
206
|
+
}
|
207
|
+
catch (NoNullAllowedException ex)
|
208
|
+
{
|
209
|
+
MessageBox.Show(ex.Message + "\n\nデータを修正するか終了してください。",
|
210
|
+
"「最後に移動」ボタン操作エラー",
|
211
|
+
MessageBoxButtons.OK, MessageBoxIcon.Exclamation);
|
212
|
+
}
|
213
|
+
}
|
214
|
+
|
215
|
+
private void bindingNavigatorAddNewItem_Click(object sender, EventArgs e)
|
216
|
+
{
|
217
|
+
try
|
218
|
+
{
|
219
|
+
productBindingSource.AddNew();
|
220
|
+
}
|
221
|
+
catch (NoNullAllowedException ex)
|
222
|
+
{
|
223
|
+
MessageBox.Show(ex.Message + "\n\nデータを修正するか終了してください。",
|
224
|
+
"「新規追加」ボタン操作エラー",
|
225
|
+
MessageBoxButtons.OK, MessageBoxIcon.Exclamation);
|
226
|
+
}
|
227
|
+
}
|
228
|
+
|
229
|
+
private void bindingNavigatorDeleteItem_Click(object sender, EventArgs e)
|
230
|
+
{
|
231
|
+
productBindingSource.RemoveCurrent();
|
232
|
+
}
|
233
|
+
}
|
234
|
+
}
|
235
|
+
```
|
1
タイポ訂正
test
CHANGED
@@ -18,7 +18,7 @@
|
|
18
18
|
DataGridView.DataError イベント
|
19
19
|
https://learn.microsoft.com/ja-jp/dotnet/api/system.windows.forms.datagridview.dataerror?view=netframework-4.8
|
20
20
|
|
21
|
-
ただし、BindingNa
|
21
|
+
ただし、BindingNavigator の + ボタンを 2 回クリックしたり、新規行に未入力で MoveFirst ボタンをクリックしたりすると補足できないという問題があるはずです。そこまで何とかしたいということですと、かなり手を加える必要があります。具体例は以下の記事を見てください。
|
22
22
|
|
23
23
|
NoNullAllowedExceptionのエラーをチェックができない
|
24
24
|
https://social.msdn.microsoft.com/Forums/ja-JP/45d6e9b4-67cf-48b6-a564-5cddcf6a59e6/nonullallowedexception1239812456125211254012434124811245512483124631236?forum=csharpgeneralja
|