回答編集履歴
5
修正
test
CHANGED
@@ -7,6 +7,7 @@
|
|
7
7
|
|
8
8
|
----
|
9
9
|
|
10
|
+
MainActivity.kt
|
10
11
|
```kotlin
|
11
12
|
import androidx.appcompat.app.AppCompatActivity
|
12
13
|
import android.os.Bundle
|
@@ -63,23 +64,13 @@
|
|
63
64
|
res/layout/activity_main.xml
|
64
65
|
```xml
|
65
66
|
<?xml version="1.0" encoding="utf-8"?>
|
66
|
-
<and
|
67
|
+
<ExpandableListView
|
67
68
|
xmlns:android="http://schemas.android.com/apk/res/android"
|
68
|
-
xmlns:app="http://schemas.android.com/apk/res-auto"
|
69
69
|
xmlns:tools="http://schemas.android.com/tools"
|
70
|
+
tools:context=".MainActivity"
|
71
|
+
android:id="@+id/exList"
|
70
72
|
android:layout_width="match_parent"
|
71
|
-
android:layout_height="match_parent"
|
73
|
+
android:layout_height="match_parent" />
|
72
|
-
tools:context=".MainActivity">
|
73
|
-
<ExpandableListView
|
74
|
-
android:id="@+id/exList"
|
75
|
-
android:layout_width="0dp"
|
76
|
-
android:layout_height="0dp"
|
77
|
-
android:text="Hello World!"
|
78
|
-
app:layout_constraintBottom_toBottomOf="parent"
|
79
|
-
app:layout_constraintEnd_toEndOf="parent"
|
80
|
-
app:layout_constraintStart_toStartOf="parent"
|
81
|
-
app:layout_constraintTop_toTopOf="parent" />
|
82
|
-
</androidx.constraintlayout.widget.ConstraintLayout>
|
83
74
|
```
|
84
75
|
res/layout/test1.xml
|
85
76
|
```xml
|
4
コード追加
test
CHANGED
@@ -4,3 +4,101 @@
|
|
4
4
|
[公式ドキュメント](https://developer.android.com/reference/android/widget/ExpandableListAdapter)に真っ先に
|
5
5
|
>An adapter that links a [ExpandableListView](https://developer.android.com/reference/android/widget/ExpandableListView) with the underlying data.
|
6
6
|
と書いてあります。
|
7
|
+
|
8
|
+
----
|
9
|
+
|
10
|
+
```kotlin
|
11
|
+
import androidx.appcompat.app.AppCompatActivity
|
12
|
+
import android.os.Bundle
|
13
|
+
import android.view.LayoutInflater
|
14
|
+
import android.view.View
|
15
|
+
import android.view.ViewGroup
|
16
|
+
import android.widget.BaseExpandableListAdapter
|
17
|
+
import android.widget.ExpandableListView
|
18
|
+
|
19
|
+
class MainActivity : AppCompatActivity() {
|
20
|
+
override fun onCreate(savedInstanceState: Bundle?) {
|
21
|
+
super.onCreate(savedInstanceState)
|
22
|
+
setContentView(R.layout.activity_main)
|
23
|
+
|
24
|
+
var exList = findViewById<ExpandableListView>(R.id.exList)
|
25
|
+
exList.setAdapter(ExListAdapter())
|
26
|
+
}
|
27
|
+
}
|
28
|
+
|
29
|
+
class ExListAdapter : BaseExpandableListAdapter() {
|
30
|
+
override fun getGroupCount(): Int = 1
|
31
|
+
override fun getGroupId(groupPosition: Int): Long = 0
|
32
|
+
override fun getGroup(groupPosition: Int): Any = ""
|
33
|
+
override fun getGroupView(groupPosition: Int,
|
34
|
+
isExpanded: Boolean,
|
35
|
+
convertView: View?,
|
36
|
+
parent: ViewGroup): View {
|
37
|
+
var view = convertView
|
38
|
+
if(view == null) {
|
39
|
+
view = LayoutInflater.from(parent.context).inflate(R.layout.test1, parent, false)
|
40
|
+
}
|
41
|
+
return view!!
|
42
|
+
}
|
43
|
+
|
44
|
+
override fun getChildrenCount(groupPosition: Int): Int = 1
|
45
|
+
override fun getChild(groupPosition: Int, childPosition: Int): Any = ""
|
46
|
+
override fun getChildId(groupPosition: Int, childPosition: Int): Long = 0
|
47
|
+
override fun getChildView(groupPosition: Int,
|
48
|
+
childPosition: Int,
|
49
|
+
isLastChild: Boolean,
|
50
|
+
convertView: View?,
|
51
|
+
parent: ViewGroup): View {
|
52
|
+
var view = convertView
|
53
|
+
if(view == null) {
|
54
|
+
view = LayoutInflater.from(parent.context).inflate(R.layout.test2, parent, false)
|
55
|
+
}
|
56
|
+
return view!!
|
57
|
+
}
|
58
|
+
override fun isChildSelectable(groupPosition: Int, childPosition: Int): Boolean = false
|
59
|
+
|
60
|
+
override fun hasStableIds(): Boolean = true
|
61
|
+
}
|
62
|
+
```
|
63
|
+
res/layout/activity_main.xml
|
64
|
+
```xml
|
65
|
+
<?xml version="1.0" encoding="utf-8"?>
|
66
|
+
<androidx.constraintlayout.widget.ConstraintLayout
|
67
|
+
xmlns:android="http://schemas.android.com/apk/res/android"
|
68
|
+
xmlns:app="http://schemas.android.com/apk/res-auto"
|
69
|
+
xmlns:tools="http://schemas.android.com/tools"
|
70
|
+
android:layout_width="match_parent"
|
71
|
+
android:layout_height="match_parent"
|
72
|
+
tools:context=".MainActivity">
|
73
|
+
<ExpandableListView
|
74
|
+
android:id="@+id/exList"
|
75
|
+
android:layout_width="0dp"
|
76
|
+
android:layout_height="0dp"
|
77
|
+
android:text="Hello World!"
|
78
|
+
app:layout_constraintBottom_toBottomOf="parent"
|
79
|
+
app:layout_constraintEnd_toEndOf="parent"
|
80
|
+
app:layout_constraintStart_toStartOf="parent"
|
81
|
+
app:layout_constraintTop_toTopOf="parent" />
|
82
|
+
</androidx.constraintlayout.widget.ConstraintLayout>
|
83
|
+
```
|
84
|
+
res/layout/test1.xml
|
85
|
+
```xml
|
86
|
+
<?xml version="1.0" encoding="utf-8"?>
|
87
|
+
<TextView
|
88
|
+
xmlns:android="http://schemas.android.com/apk/res/android"
|
89
|
+
android:layout_width="match_parent"
|
90
|
+
android:layout_height="match_parent"
|
91
|
+
android:text="Text 1"
|
92
|
+
android:paddingStart="30dp"/>
|
93
|
+
```
|
94
|
+
res/layout/test2.xml
|
95
|
+
```xml
|
96
|
+
<?xml version="1.0" encoding="utf-8"?>
|
97
|
+
<TextView
|
98
|
+
xmlns:android="http://schemas.android.com/apk/res/android"
|
99
|
+
android:layout_width="match_parent"
|
100
|
+
android:layout_height="match_parent"
|
101
|
+
android:text="Text 2"
|
102
|
+
android:paddingStart="35dp"/>
|
103
|
+
```
|
104
|
+
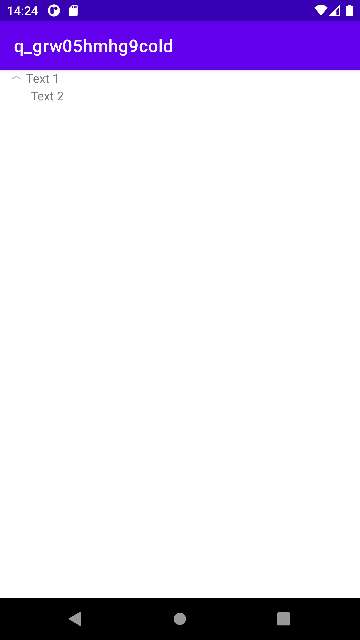
|
3
追加
test
CHANGED
@@ -1,2 +1,6 @@
|
|
1
1
|
ExpandableListAdapter なのですから、 ExpandableListView で使うのではないでしょうか。
|
2
|
+
|
2
3
|
なぜ ExpandableListAdapter に辿り着いて ExpandableListView に辿り着かないのかが不思議な感じがしますが。
|
4
|
+
[公式ドキュメント](https://developer.android.com/reference/android/widget/ExpandableListAdapter)に真っ先に
|
5
|
+
>An adapter that links a [ExpandableListView](https://developer.android.com/reference/android/widget/ExpandableListView) with the underlying data.
|
6
|
+
と書いてあります。
|
2
修正
test
CHANGED
@@ -1,2 +1,2 @@
|
|
1
|
-
ExpandableListAdapter なのですから、 ExpandableList で使うのではないでしょうか。
|
1
|
+
ExpandableListAdapter なのですから、 ExpandableListView で使うのではないでしょうか。
|
2
|
-
なぜ ExpandableListAdapter に辿り着いて ExpandableList に辿り着かないのかが不思議な感じがしますが。
|
2
|
+
なぜ ExpandableListAdapter に辿り着いて ExpandableListView に辿り着かないのかが不思議な感じがしますが。
|
1
追加
test
CHANGED
@@ -1 +1,2 @@
|
|
1
1
|
ExpandableListAdapter なのですから、 ExpandableList で使うのではないでしょうか。
|
2
|
+
なぜ ExpandableListAdapter に辿り着いて ExpandableList に辿り着かないのかが不思議な感じがしますが。
|