回答編集履歴
4
修正
test
CHANGED
@@ -114,7 +114,7 @@
|
|
114
114
|
|
115
115
|
</androidx.constraintlayout.widget.ConstraintLayout>
|
116
116
|
```
|
117
|
-
AndroidManifest
|
117
|
+
AndroidManifest.xml: Application タグ内アクティビティ定義
|
118
118
|
```xml
|
119
119
|
<activity
|
120
120
|
android:name=".MainActivity"
|
3
コード追加
test
CHANGED
@@ -2,3 +2,136 @@
|
|
2
2
|
任意のオブジェクトを渡す場合は [putExtra(String, Parcelable)](https://developer.android.com/reference/android/content/Intent#putExtra(java.lang.String,%20android.os.Parcelable)) か [putExtra(String, Serializable)](https://developer.android.com/reference/android/content/Intent#putExtra(java.lang.String,%20java.io.Serializable)) を利用することになります。
|
3
3
|
|
4
4
|
例えば User クラスに [Serializable](https://developer.android.com/reference/java/io/Serializable) を implements しては如何でしょうか。
|
5
|
+
|
6
|
+
---
|
7
|
+
|
8
|
+
MainActivity.java
|
9
|
+
```java
|
10
|
+
import androidx.appcompat.app.AppCompatActivity;
|
11
|
+
|
12
|
+
import android.content.Intent;
|
13
|
+
import android.os.Bundle;
|
14
|
+
import android.widget.Button;
|
15
|
+
|
16
|
+
import java.io.Serializable;
|
17
|
+
|
18
|
+
public class MainActivity extends AppCompatActivity {
|
19
|
+
public static final String EXTRA_USER = "user";
|
20
|
+
|
21
|
+
@Override
|
22
|
+
protected void onCreate(Bundle savedInstanceState) {
|
23
|
+
super.onCreate(savedInstanceState);
|
24
|
+
setContentView(R.layout.activity_main);
|
25
|
+
|
26
|
+
Button button = findViewById(R.id.button);
|
27
|
+
button.setOnClickListener(v -> {
|
28
|
+
User user = new User("id1", "name2");
|
29
|
+
Intent intent = new Intent(this, SubActivity.class);
|
30
|
+
intent.putExtra(EXTRA_USER, user);
|
31
|
+
startActivity(intent);
|
32
|
+
});
|
33
|
+
}
|
34
|
+
}
|
35
|
+
|
36
|
+
class User implements Serializable {
|
37
|
+
final String id, name;
|
38
|
+
User(String id, String name) {
|
39
|
+
this.id = id;
|
40
|
+
this.name = name;
|
41
|
+
}
|
42
|
+
@Override
|
43
|
+
public String toString() {
|
44
|
+
return getClass().getSimpleName()+"[id="+id+", name="+name+"]";
|
45
|
+
}
|
46
|
+
}
|
47
|
+
```
|
48
|
+
res/layout/activity_main.xml
|
49
|
+
```xml
|
50
|
+
<?xml version="1.0" encoding="utf-8"?>
|
51
|
+
<androidx.constraintlayout.widget.ConstraintLayout
|
52
|
+
xmlns:android="http://schemas.android.com/apk/res/android"
|
53
|
+
xmlns:app="http://schemas.android.com/apk/res-auto"
|
54
|
+
xmlns:tools="http://schemas.android.com/tools"
|
55
|
+
android:layout_width="match_parent"
|
56
|
+
android:layout_height="match_parent"
|
57
|
+
tools:context=".MainActivity">
|
58
|
+
|
59
|
+
<Button
|
60
|
+
android:id="@+id/button"
|
61
|
+
android:layout_width="wrap_content"
|
62
|
+
android:layout_height="wrap_content"
|
63
|
+
android:text="Hello World!"
|
64
|
+
app:layout_constraintBottom_toBottomOf="parent"
|
65
|
+
app:layout_constraintEnd_toEndOf="parent"
|
66
|
+
app:layout_constraintStart_toStartOf="parent"
|
67
|
+
app:layout_constraintTop_toTopOf="parent" />
|
68
|
+
|
69
|
+
</androidx.constraintlayout.widget.ConstraintLayout>
|
70
|
+
```
|
71
|
+
SubActivity.java
|
72
|
+
```java
|
73
|
+
import androidx.appcompat.app.AppCompatActivity;
|
74
|
+
|
75
|
+
import android.content.Intent;
|
76
|
+
import android.os.Bundle;
|
77
|
+
import android.widget.TextView;
|
78
|
+
|
79
|
+
public class SubActivity extends AppCompatActivity {
|
80
|
+
@Override
|
81
|
+
protected void onCreate(Bundle savedInstanceState) {
|
82
|
+
super.onCreate(savedInstanceState);
|
83
|
+
setContentView(R.layout.activity_sub);
|
84
|
+
|
85
|
+
Intent intent = getIntent();
|
86
|
+
User user = (User)intent.getSerializableExtra(MainActivity.EXTRA_USER);
|
87
|
+
|
88
|
+
TextView textView = findViewById(R.id.text);
|
89
|
+
textView.setText("" + user);
|
90
|
+
}
|
91
|
+
}
|
92
|
+
```
|
93
|
+
res/layout/activity_sub.xml
|
94
|
+
```xml
|
95
|
+
<?xml version="1.0" encoding="utf-8"?>
|
96
|
+
<androidx.constraintlayout.widget.ConstraintLayout
|
97
|
+
xmlns:android="http://schemas.android.com/apk/res/android"
|
98
|
+
xmlns:app="http://schemas.android.com/apk/res-auto"
|
99
|
+
xmlns:tools="http://schemas.android.com/tools"
|
100
|
+
android:layout_width="match_parent"
|
101
|
+
android:layout_height="match_parent"
|
102
|
+
android:background="#f0f000"
|
103
|
+
tools:context=".SubActivity">
|
104
|
+
|
105
|
+
<TextView
|
106
|
+
android:id="@+id/text"
|
107
|
+
android:layout_width="wrap_content"
|
108
|
+
android:layout_height="wrap_content"
|
109
|
+
android:text="Sub World!"
|
110
|
+
app:layout_constraintBottom_toBottomOf="parent"
|
111
|
+
app:layout_constraintEnd_toEndOf="parent"
|
112
|
+
app:layout_constraintStart_toStartOf="parent"
|
113
|
+
app:layout_constraintTop_toTopOf="parent" />
|
114
|
+
|
115
|
+
</androidx.constraintlayout.widget.ConstraintLayout>
|
116
|
+
```
|
117
|
+
AndroidManifest,xml 内 Application タグ内アクティビティ定義
|
118
|
+
```xml
|
119
|
+
<activity
|
120
|
+
android:name=".MainActivity"
|
121
|
+
android:exported="true">
|
122
|
+
<intent-filter>
|
123
|
+
<action android:name="android.intent.action.MAIN" />
|
124
|
+
|
125
|
+
<category android:name="android.intent.category.LAUNCHER" />
|
126
|
+
</intent-filter>
|
127
|
+
</activity>
|
128
|
+
<activity
|
129
|
+
android:name=".SubActivity"
|
130
|
+
android:exported="false"
|
131
|
+
android:parentActivityName=".MainActivity">
|
132
|
+
<meta-data
|
133
|
+
android:name="android.support.PARENT_ACTIVITY"
|
134
|
+
android:value=".MainActivity" />
|
135
|
+
</activity>
|
136
|
+
```
|
137
|
+
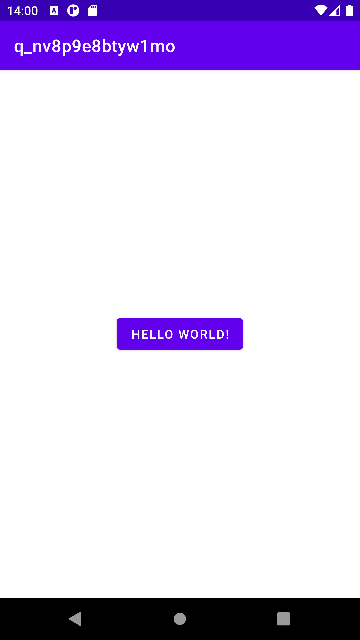 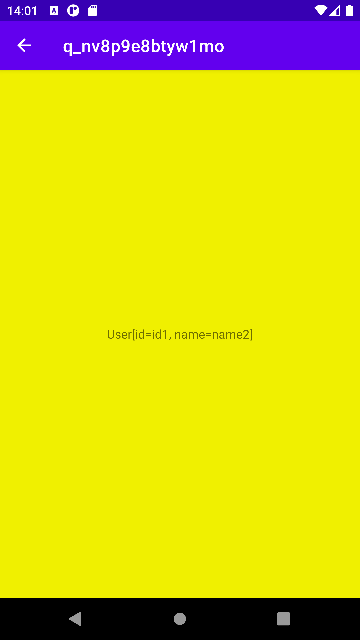
|
2
リンク追加
test
CHANGED
@@ -1,4 +1,4 @@
|
|
1
|
-
エラーメッセージの通り、 Intent に putExtra(String, User) というメソッドはありません。
|
2
|
-
任意のオブジェクトを渡す場合は putExtra(String, Parcelable) か putExtra(String, Serializable) を利用することになります。
|
1
|
+
エラーメッセージの通り、 [Intent](https://developer.android.com/reference/android/content/Intent) に putExtra(String, User) というメソッドはありません。
|
2
|
+
任意のオブジェクトを渡す場合は [putExtra(String, Parcelable)](https://developer.android.com/reference/android/content/Intent#putExtra(java.lang.String,%20android.os.Parcelable)) か [putExtra(String, Serializable)](https://developer.android.com/reference/android/content/Intent#putExtra(java.lang.String,%20java.io.Serializable)) を利用することになります。
|
3
3
|
|
4
|
-
例えば User クラスに Serializable を implements しては如何でしょうか。
|
4
|
+
例えば User クラスに [Serializable](https://developer.android.com/reference/java/io/Serializable) を implements しては如何でしょうか。
|
1
追加
test
CHANGED
@@ -1 +1,4 @@
|
|
1
|
+
エラーメッセージの通り、 Intent に putExtra(String, User) というメソッドはありません。
|
2
|
+
任意のオブジェクトを渡す場合は putExtra(String, Parcelable) か putExtra(String, Serializable) を利用することになります。
|
3
|
+
|
1
|
-
User クラスに Serializable を implements しては如何でしょうか。
|
4
|
+
例えば User クラスに Serializable を implements しては如何でしょうか。
|