質問編集履歴
3
レイアウト、アクティビティのコードを記載しました。
test
CHANGED
File without changes
|
test
CHANGED
@@ -15,3 +15,258 @@
|
|
15
15
|
絵コンテを雑ながら追加してみました。
|
16
16
|
|
17
17
|
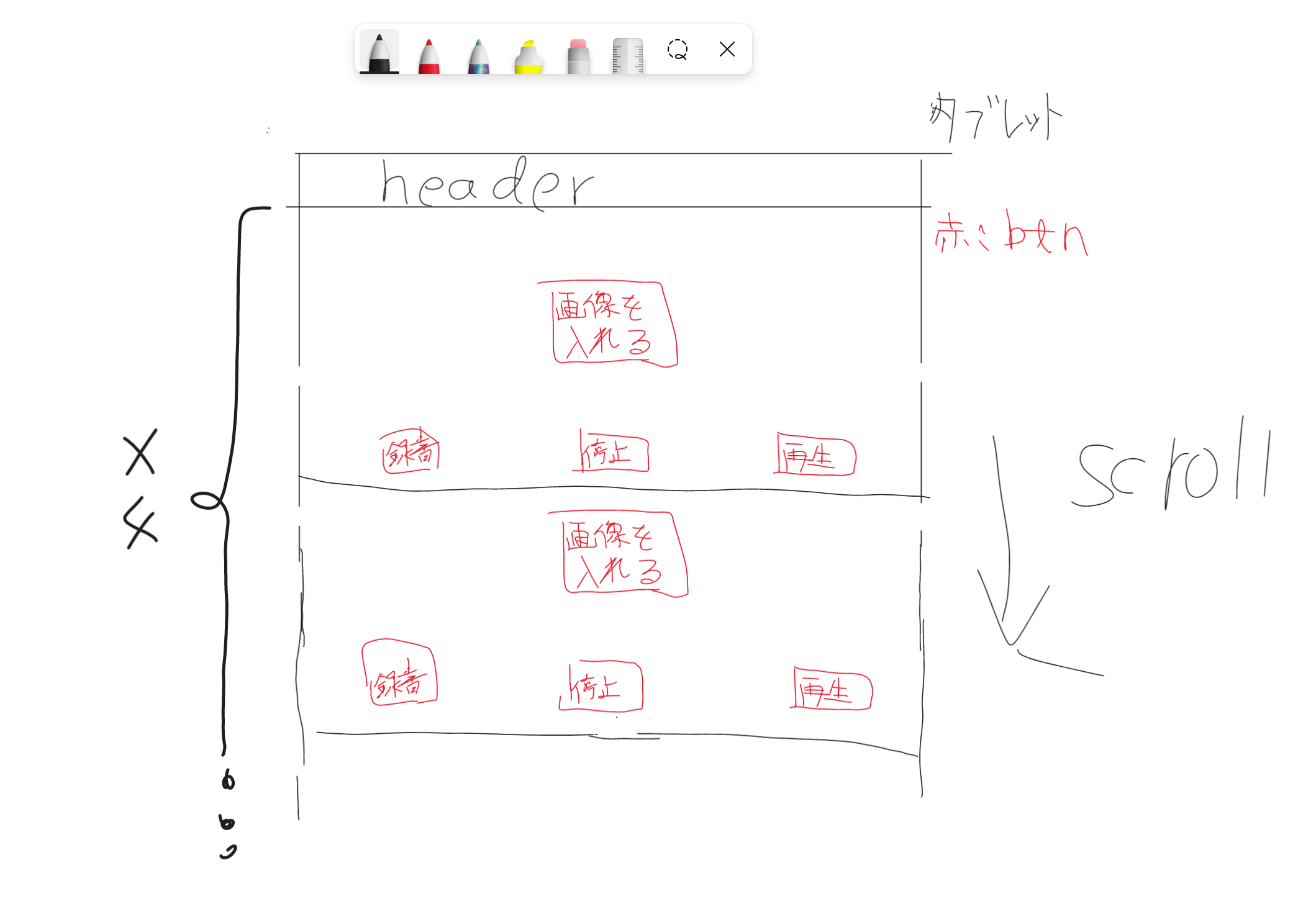
|
18
|
+
|
19
|
+
```レイアウトです。
|
20
|
+
<?xml version="1.0" encoding="utf-8"?>
|
21
|
+
|
22
|
+
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
|
23
|
+
xmlns:app="http://schemas.android.com/apk/res-auto"
|
24
|
+
xmlns:tools="http://schemas.android.com/tools"
|
25
|
+
android:layout_width="match_parent"
|
26
|
+
android:layout_height="match_parent"
|
27
|
+
tools:context=".SecondActivity">
|
28
|
+
|
29
|
+
<LinearLayout
|
30
|
+
android:id="@+id/linearLayout3"
|
31
|
+
android:layout_width="wrap_content"
|
32
|
+
android:layout_height="wrap_content"
|
33
|
+
android:orientation="horizontal"
|
34
|
+
app:layout_constraintBottom_toBottomOf="parent"
|
35
|
+
app:layout_constraintEnd_toEndOf="parent"
|
36
|
+
app:layout_constraintStart_toStartOf="parent">
|
37
|
+
|
38
|
+
<Button
|
39
|
+
android:id="@+id/record"
|
40
|
+
android:layout_width="wrap_content"
|
41
|
+
android:layout_height="wrap_content"
|
42
|
+
android:layout_marginLeft="50dp"
|
43
|
+
android:layout_marginRight="50dp"
|
44
|
+
android:text="録音"
|
45
|
+
android:textSize="20sp" />
|
46
|
+
|
47
|
+
<Button
|
48
|
+
android:id="@+id/stop"
|
49
|
+
android:layout_width="wrap_content"
|
50
|
+
android:layout_height="wrap_content"
|
51
|
+
android:layout_marginLeft="50dp"
|
52
|
+
android:layout_marginRight="50dp"
|
53
|
+
android:text="停止"
|
54
|
+
android:textSize="20sp" />
|
55
|
+
|
56
|
+
<Button
|
57
|
+
android:id="@+id/playback"
|
58
|
+
android:layout_width="wrap_content"
|
59
|
+
android:layout_height="wrap_content"
|
60
|
+
android:layout_marginLeft="50dp"
|
61
|
+
android:layout_marginRight="50dp"
|
62
|
+
android:text="再生"
|
63
|
+
android:textSize="20sp" />
|
64
|
+
</LinearLayout>
|
65
|
+
|
66
|
+
<ImageView
|
67
|
+
android:id="@+id/imageView"
|
68
|
+
android:layout_width="wrap_content"
|
69
|
+
android:layout_height="wrap_content"
|
70
|
+
app:layout_constraintBottom_toTopOf="@+id/linearLayout3"
|
71
|
+
app:layout_constraintEnd_toEndOf="parent"
|
72
|
+
app:layout_constraintStart_toStartOf="parent"
|
73
|
+
app:layout_constraintTop_toBottomOf="@+id/linearLayout2"
|
74
|
+
tools:srcCompat="@tools:sample/avatars" />
|
75
|
+
|
76
|
+
<Button
|
77
|
+
android:id="@+id/picturebutton"
|
78
|
+
android:layout_width="wrap_content"
|
79
|
+
android:layout_height="wrap_content"
|
80
|
+
android:text="画像を挿入する"
|
81
|
+
app:layout_constraintBottom_toTopOf="@+id/linearLayout3"
|
82
|
+
app:layout_constraintEnd_toEndOf="parent"
|
83
|
+
app:layout_constraintStart_toStartOf="parent"
|
84
|
+
app:layout_constraintTop_toBottomOf="@+id/linearLayout2" />
|
85
|
+
|
86
|
+
</androidx.constraintlayout.widget.ConstraintLayout>
|
87
|
+
|
88
|
+
|
89
|
+
```
|
90
|
+
|
91
|
+
```
|
92
|
+
|
93
|
+
private const val LOG_TAG = "AudioRecordTest"
|
94
|
+
private const val REQUEST_RECORD_AUDIO_PERMISSION = 200
|
95
|
+
|
96
|
+
class SecondActivity : AppCompatActivity() {
|
97
|
+
private var recorder: MediaRecorder? = null
|
98
|
+
private var fileName: String = ""
|
99
|
+
|
100
|
+
private var player: MediaPlayer? = null
|
101
|
+
|
102
|
+
|
103
|
+
private var permissionToRecordAccepted = false
|
104
|
+
private var permissions: Array<String> = arrayOf(Manifest.permission.RECORD_AUDIO)
|
105
|
+
|
106
|
+
override fun onRequestPermissionsResult(
|
107
|
+
requestCode: Int,
|
108
|
+
permissions: Array<String>,
|
109
|
+
grantResults: IntArray
|
110
|
+
) {
|
111
|
+
super.onRequestPermissionsResult(requestCode, permissions, grantResults)
|
112
|
+
permissionToRecordAccepted = if (requestCode == REQUEST_RECORD_AUDIO_PERMISSION) {
|
113
|
+
grantResults[0] == PackageManager.PERMISSION_GRANTED
|
114
|
+
} else {
|
115
|
+
false
|
116
|
+
}
|
117
|
+
if (!permissionToRecordAccepted) finish()
|
118
|
+
}
|
119
|
+
|
120
|
+
private fun onRecord(start: Boolean) = if (start) {
|
121
|
+
startRecording()
|
122
|
+
} else {
|
123
|
+
stopRecording()
|
124
|
+
}
|
125
|
+
|
126
|
+
private fun onPlay(start: Boolean) = if (start) {
|
127
|
+
startPlaying()
|
128
|
+
} else {
|
129
|
+
stopPlaying()
|
130
|
+
}
|
131
|
+
|
132
|
+
private fun startPlaying() {
|
133
|
+
player = MediaPlayer().apply {
|
134
|
+
try {
|
135
|
+
setDataSource(fileName)
|
136
|
+
prepare()
|
137
|
+
start()
|
138
|
+
} catch (e: IOException) {
|
139
|
+
Log.e(LOG_TAG, "prepare() failed")
|
140
|
+
}
|
141
|
+
}
|
142
|
+
}
|
143
|
+
|
144
|
+
private fun stopPlaying() {
|
145
|
+
player?.release()
|
146
|
+
player = null
|
147
|
+
}
|
148
|
+
|
149
|
+
|
150
|
+
|
151
|
+
|
152
|
+
|
153
|
+
private fun startRecording() {
|
154
|
+
recorder = MediaRecorder().apply {
|
155
|
+
setAudioSource(MediaRecorder.AudioSource.MIC)
|
156
|
+
setOutputFormat(MediaRecorder.OutputFormat.THREE_GPP)
|
157
|
+
setOutputFile(fileName)
|
158
|
+
setAudioEncoder(MediaRecorder.AudioEncoder.AMR_NB)
|
159
|
+
|
160
|
+
try {
|
161
|
+
prepare()
|
162
|
+
} catch (e: IOException) {
|
163
|
+
Log.e(LOG_TAG, "prepare() failed")
|
164
|
+
}
|
165
|
+
|
166
|
+
start()
|
167
|
+
}
|
168
|
+
}
|
169
|
+
|
170
|
+
private fun stopRecording() {
|
171
|
+
recorder?.apply {
|
172
|
+
stop()
|
173
|
+
release()
|
174
|
+
}
|
175
|
+
recorder = null
|
176
|
+
}
|
177
|
+
|
178
|
+
|
179
|
+
|
180
|
+
|
181
|
+
override fun onCreate(savedInstanceState: Bundle?) {
|
182
|
+
|
183
|
+
fileName = "${externalCacheDir?.absolutePath}/audiorecordtest.3gp"
|
184
|
+
super.onCreate(savedInstanceState)
|
185
|
+
setContentView(R.layout.activity_second2)
|
186
|
+
|
187
|
+
var pictureButton : Button = findViewById(R.id.picturebutton)
|
188
|
+
|
189
|
+
pictureButton.setOnClickListener {
|
190
|
+
val intent = Intent(Intent.ACTION_OPEN_DOCUMENT).apply {
|
191
|
+
addCategory(Intent.CATEGORY_OPENABLE)
|
192
|
+
type = "image/*"
|
193
|
+
}
|
194
|
+
startActivityForResult(intent, READ_REQUEST_CODE)
|
195
|
+
}
|
196
|
+
|
197
|
+
ActivityCompat.requestPermissions(this, permissions, REQUEST_RECORD_AUDIO_PERMISSION)
|
198
|
+
|
199
|
+
val record = findViewById<Button>(R.id.record) //録音オブジェクト取得
|
200
|
+
val stop = findViewById<Button>(R.id.stop) //録音停止オブジェクト取得
|
201
|
+
val playback = findViewById<Button>(R.id.playback) //再生オブジェクト取得
|
202
|
+
|
203
|
+
val listener = RecordButton() //レコードボタンリスナのインスタンス生成
|
204
|
+
|
205
|
+
record.setOnClickListener(listener) //レコードボタンリスナの設定
|
206
|
+
stop.setOnClickListener(listener)
|
207
|
+
playback.setOnClickListener(listener)
|
208
|
+
|
209
|
+
val btnFinish: Button = findViewById(R.id.btnFinish)
|
210
|
+
|
211
|
+
btnFinish.setOnClickListener {
|
212
|
+
finish()
|
213
|
+
}
|
214
|
+
}
|
215
|
+
|
216
|
+
companion object{
|
217
|
+
private const val READ_REQUEST_CODE: Int = 42
|
218
|
+
}
|
219
|
+
|
220
|
+
//クリックイベントの設定
|
221
|
+
private inner class RecordButton : View.OnClickListener {
|
222
|
+
override fun onClick(v: View?) {
|
223
|
+
Log.i(LOG_TAG, "クリック成功")
|
224
|
+
Log.i(LOG_TAG, fileName)
|
225
|
+
|
226
|
+
if(v != null){
|
227
|
+
when(v.id){
|
228
|
+
//録音開始ボタン
|
229
|
+
R.id.record -> {
|
230
|
+
onRecord(true)
|
231
|
+
Log.i(LOG_TAG, "録音開始")
|
232
|
+
}
|
233
|
+
//録音停止ボタン
|
234
|
+
R.id.stop -> {
|
235
|
+
onRecord(false)
|
236
|
+
Log.i(LOG_TAG, "録音終了")
|
237
|
+
}
|
238
|
+
|
239
|
+
R.id.playback -> {
|
240
|
+
onPlay(true)
|
241
|
+
Log.i(LOG_TAG, "再生中")
|
242
|
+
}
|
243
|
+
}
|
244
|
+
}
|
245
|
+
}
|
246
|
+
}
|
247
|
+
|
248
|
+
override fun onActivityResult(requestCode: Int, resultCode: Int, resultData: Intent?) {
|
249
|
+
super.onActivityResult(requestCode, resultCode, resultData)
|
250
|
+
|
251
|
+
if (resultCode != RESULT_OK) {
|
252
|
+
return
|
253
|
+
}
|
254
|
+
when (requestCode){
|
255
|
+
READ_REQUEST_CODE -> {
|
256
|
+
try {
|
257
|
+
resultData?.data?.also { uri ->
|
258
|
+
val inputStream = contentResolver?.openInputStream(uri)
|
259
|
+
val image = BitmapFactory.decodeStream(inputStream)
|
260
|
+
val imageView = findViewById<ImageView>(R.id.imageView)
|
261
|
+
imageView.setImageBitmap(image)
|
262
|
+
}
|
263
|
+
}catch (e: Exception){
|
264
|
+
Toast.makeText(this, "エラーが発生しました", Toast.LENGTH_LONG).show()
|
265
|
+
}
|
266
|
+
}
|
267
|
+
}
|
268
|
+
}
|
269
|
+
}
|
270
|
+
```
|
271
|
+
|
272
|
+
|
2
4コマ漫画ではなくアニメーションのようなものです
test
CHANGED
File without changes
|
test
CHANGED
@@ -1,4 +1,4 @@
|
|
1
|
-
kotlinで画像と音声を用いた
|
1
|
+
kotlinで画像と音声を用いたアニメーション作成アプリを作ろうとしています。
|
2
2
|
|
3
3
|
イメージとしてはプレゼンテーションの簡易版で、画像を挿入し、音声を入れ、4つを組み合わせ動画にしたいと考えています。
|
4
4
|
|
@@ -11,3 +11,7 @@
|
|
11
11
|
また、recycler viewで繰り返す方法も調べてみましたがネットには同じものを繰り返すものが見つからず、こちらも進められずにいます。
|
12
12
|
|
13
13
|
どのようなレイアウトで作成していくのが良いのか、お力添えよろしくお願いいたします。
|
14
|
+
|
15
|
+
絵コンテを雑ながら追加してみました。
|
16
|
+
|
17
|
+
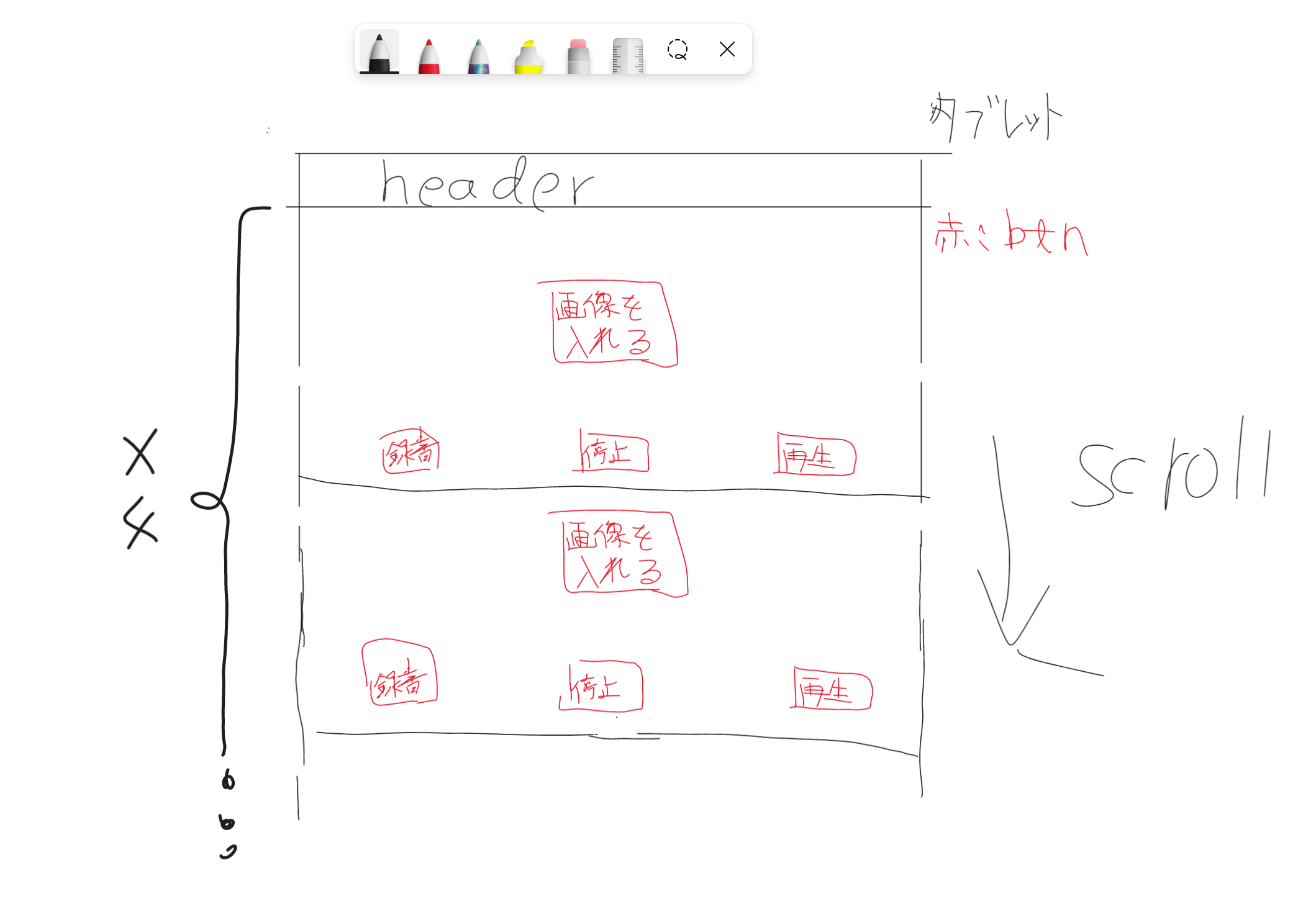
|
1
タグを付け加えました
test
CHANGED
File without changes
|
test
CHANGED
File without changes
|