質問編集履歴
2
コードを削除しました。
test
CHANGED
File without changes
|
test
CHANGED
@@ -3,155 +3,4 @@
|
|
3
3
|
至らぬ点が多いため、コード不足や補足事項必要でしたらご指摘よろしくお願いいたします。
|
4
4
|
文字数の制限のため、預金処理以降のコードしか載せられませんでした。
|
5
5
|
|
6
|
-
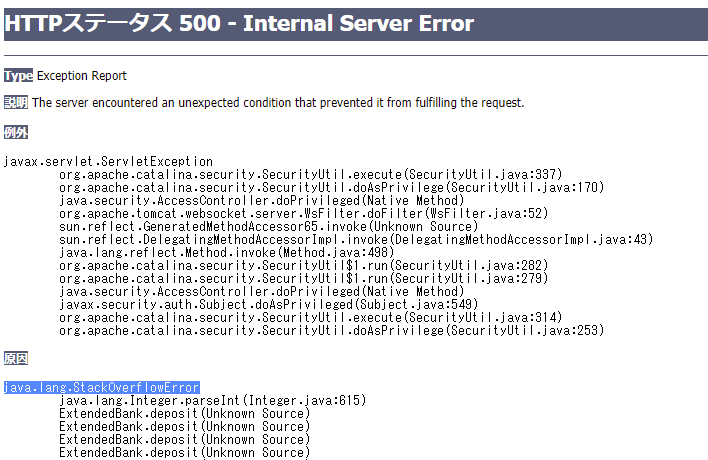
|
7
6
|
|
8
|
-
```java
|
9
|
-
import java.util.Hashtable;
|
10
|
-
|
11
|
-
public class ExtendedBank{
|
12
|
-
private Hashtable<String,Account>customer; // 口座リスト
|
13
|
-
private int balance; // 残高格納用
|
14
|
-
|
15
|
-
public ExtendedBank(){ // 口座リスト初期化
|
16
|
-
customer = new Hashtable<String,Account>();
|
17
|
-
}
|
18
|
-
|
19
|
-
public int open(String name){ // 口座開設
|
20
|
-
Account myaccount = customer.get(name);
|
21
|
-
if(myaccount != null) return -7;
|
22
|
-
else{
|
23
|
-
myaccount=new Account(name);
|
24
|
-
customer.put(name,myaccount);
|
25
|
-
return 0;
|
26
|
-
}
|
27
|
-
}
|
28
|
-
|
29
|
-
public int close(String name){ // 口座解約
|
30
|
-
Account myaccount = customer.get(name);
|
31
|
-
if(myaccount != null){
|
32
|
-
if(myaccount.showBalance() == 0){
|
33
|
-
customer.remove(name);
|
34
|
-
return 0;
|
35
|
-
}else return -1;
|
36
|
-
}else return -7;
|
37
|
-
}
|
38
|
-
|
39
|
-
|
40
|
-
public int withdraw(String name,int amount){ // 引き出し
|
41
|
-
Account myaccount = customer.get(name);
|
42
|
-
balance = myaccount.showBalance();
|
43
|
-
if(customer.get(name) != null){
|
44
|
-
if(amount <= 0)
|
45
|
-
return -3;
|
46
|
-
else if(amount > balance)
|
47
|
-
return -1;
|
48
|
-
else{
|
49
|
-
myaccount.withdraw(amount);
|
50
|
-
return 0;
|
51
|
-
}
|
52
|
-
}else return -7;
|
53
|
-
}
|
54
|
-
|
55
|
-
|
56
|
-
public int deposit(String name,int amount){ // 預金
|
57
|
-
Account myaccount = customer.get(name);
|
58
|
-
if(customer.get(name) == null)
|
59
|
-
return -7;
|
60
|
-
else if(amount <= 0)
|
61
|
-
return -3;
|
62
|
-
else{
|
63
|
-
myaccount.deposit(amount);
|
64
|
-
return 0;
|
65
|
-
}
|
66
|
-
}
|
67
|
-
|
68
|
-
|
69
|
-
public int showBalance(String name){ // 残高照会
|
70
|
-
Account myaccount = customer.get(name);
|
71
|
-
if(customer.get(name) != null) return myaccount.showBalance();
|
72
|
-
else return -7;
|
73
|
-
}
|
74
|
-
|
75
|
-
// ExtendedBank要素
|
76
|
-
// 預金
|
77
|
-
public int deposit(String name,String amount){ // name:口座名
|
78
|
-
int result;
|
79
|
-
int d_result;
|
80
|
-
try{
|
81
|
-
result = Integer.parseInt(amount);
|
82
|
-
}catch(NumberFormatException e){
|
83
|
-
if(showBalance(name) == -7)
|
84
|
-
return -7;
|
85
|
-
else
|
86
|
-
return -4;
|
87
|
-
}
|
88
|
-
|
89
|
-
result = Integer.parseInt(amount);
|
90
|
-
if(result <= 0)
|
91
|
-
return -3;
|
92
|
-
else{
|
93
|
-
d_result=deposit(name,amount);
|
94
|
-
return d_result;
|
95
|
-
}
|
96
|
-
}
|
97
|
-
|
98
|
-
// 引き出し
|
99
|
-
public int withdraw(String name,String amount){ // name:口座名
|
100
|
-
int result;
|
101
|
-
int w_result;
|
102
|
-
try{
|
103
|
-
result = Integer.parseInt(amount);
|
104
|
-
}catch(NumberFormatException e){
|
105
|
-
if(showBalance(name) == -7)
|
106
|
-
return -7;
|
107
|
-
else
|
108
|
-
return -4;
|
109
|
-
}
|
110
|
-
|
111
|
-
result = Integer.parseInt(amount);
|
112
|
-
if(result <= 0)
|
113
|
-
return -3;
|
114
|
-
else if(result > balance)
|
115
|
-
return -1;
|
116
|
-
else{
|
117
|
-
w_result=withdraw(name,amount);
|
118
|
-
return w_result;
|
119
|
-
}
|
120
|
-
}
|
121
|
-
}
|
122
|
-
```
|
123
|
-
```java
|
124
|
-
public class Account{
|
125
|
-
private String name; //口座名
|
126
|
-
private int balance; //口座の残高
|
127
|
-
|
128
|
-
public Account(String myName){ //口座名
|
129
|
-
name = myName;
|
130
|
-
balance = 0;
|
131
|
-
}
|
132
|
-
|
133
|
-
public int deposit(int amount){ //預金額
|
134
|
-
if(amount <= 0)
|
135
|
-
return -3;
|
136
|
-
else
|
137
|
-
balance += amount;
|
138
|
-
return 0;
|
139
|
-
}
|
140
|
-
|
141
|
-
public int withdraw(int amount){ //出金額
|
142
|
-
if(amount <= 0)
|
143
|
-
return -3;
|
144
|
-
else if(amount > balance)
|
145
|
-
return -1;
|
146
|
-
else
|
147
|
-
balance -= amount;
|
148
|
-
return 0;
|
149
|
-
}
|
150
|
-
|
151
|
-
public int showBalance(){ //残高照会
|
152
|
-
return balance;
|
153
|
-
}
|
154
|
-
}
|
155
|
-
|
156
|
-
```
|
157
|
-
|
1
サーブレットのコードを削除し、ExtendedBank,Accountのコードを載せました。
test
CHANGED
File without changes
|
test
CHANGED
@@ -6,223 +6,152 @@
|
|
6
6
|
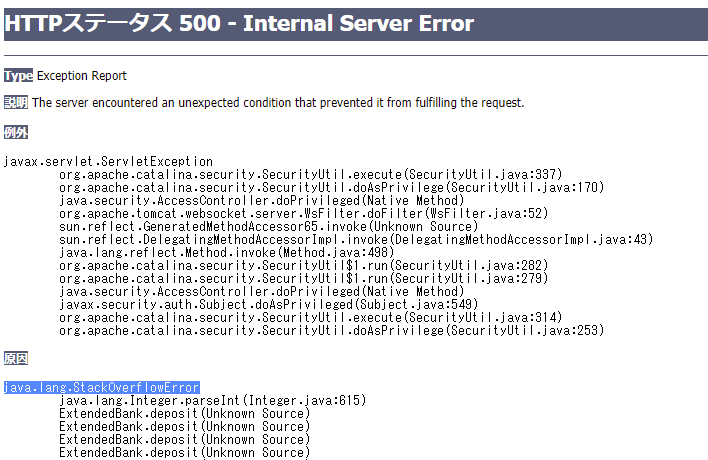
|
7
7
|
|
8
8
|
```java
|
9
|
-
import java.io.IOException;
|
10
|
-
import java.i
|
9
|
+
import java.util.Hashtable;
|
11
|
-
import javax.servlet.ServletException;
|
12
|
-
import javax.servlet.http.HttpServlet;
|
13
|
-
import javax.servlet.http.HttpServletRequest;
|
14
|
-
import javax.servlet.http.HttpServletResponse;
|
15
10
|
|
16
|
-
public class
|
17
|
-
private
|
18
|
-
p
|
19
|
-
|
20
|
-
|
21
|
-
|
22
|
-
|
23
|
-
|
24
|
-
|
25
|
-
|
26
|
-
|
27
|
-
|
28
|
-
|
29
|
-
|
30
|
-
|
31
|
-
|
32
|
-
|
33
|
-
|
34
|
-
|
35
|
-
|
36
|
-
|
37
|
-
|
38
|
-
|
39
|
-
|
40
|
-
|
41
|
-
|
42
|
-
|
43
|
-
|
44
|
-
|
45
|
-
|
46
|
-
|
47
|
-
|
48
|
-
|
49
|
-
|
50
|
-
|
51
|
-
|
52
|
-
|
53
|
-
|
54
|
-
|
55
|
-
|
56
|
-
+"<body>"
|
57
|
-
+"<div class=\"main\">"
|
58
|
-
+"<h1>預金失敗</h1>"
|
59
|
-
+"<h2>"+ b_name +"様の口座に"+ amount +"円の預金に失敗しました。</h2>"
|
60
|
-
+"<h2>0円以下の金額は預金できません。</h2>"
|
61
|
-
+"<h3>口座解約の際は残高を0円にしてから解約手続きを行ってください。</h3>"
|
62
|
-
+"</div>"
|
63
|
-
+"<a class=\"success\" href=\"index.html\">メインメニューに戻る</a>"
|
64
|
-
+"</body>"
|
65
|
-
+"</html>");
|
66
|
-
}
|
67
|
-
else if(result == -4){
|
68
|
-
pw.println(
|
69
|
-
"<!DOCTYPE html>"
|
70
|
-
+"<html>"
|
71
|
-
+"<head>"
|
72
|
-
+"<meta charset=\"UTF-8\">"
|
73
|
-
+"</head>"
|
74
|
-
+"<body>"
|
75
|
-
+"<div class=\"main\">"
|
76
|
-
+"<h1>預金失敗</h1>"
|
77
|
-
+"<h2>"+ b_name +"様の口座に"+ amount +"円の預金に失敗しました。</h2>"
|
78
|
-
+"<h2>整数以外の入力を確認しました。0以上の整数を入力してください。</h2>"
|
79
|
-
+"<h3>口座解約の際は残高を0円にしてから解約手続きを行ってください。</h3>"
|
80
|
-
+"</div>"
|
81
|
-
+"<a class=\"success\" href=\"index.html\">メインメニューに戻る</a>"
|
82
|
-
+"</body>"
|
83
|
-
+"</html>");
|
84
|
-
}
|
85
|
-
else if(result == -7){
|
86
|
-
pw.println(
|
87
|
-
"<!DOCTYPE html>"
|
88
|
-
+"<html>"
|
89
|
-
+"<head>"
|
90
|
-
+"<meta charset=\"UTF-8\">"
|
91
|
-
+"</head>"
|
92
|
-
+"<body>"
|
93
|
-
+"<div class=\"main\">"
|
94
|
-
+"<h1>預金失敗</h1>"
|
95
|
-
+"<h2>"+ b_name +"様の口座は存在しません。</h2>"
|
96
|
-
+"</div>"
|
97
|
-
+"<a class=\"success\" href=\"index.html\">メインメニューに戻る</a>"
|
98
|
-
+"</body>"
|
99
|
-
+"</html>");
|
100
|
-
}
|
11
|
+
public class ExtendedBank{
|
12
|
+
private Hashtable<String,Account>customer; // 口座リスト
|
13
|
+
private int balance; // 残高格納用
|
14
|
+
|
15
|
+
public ExtendedBank(){ // 口座リスト初期化
|
16
|
+
customer = new Hashtable<String,Account>();
|
17
|
+
}
|
18
|
+
|
19
|
+
public int open(String name){ // 口座開設
|
20
|
+
Account myaccount = customer.get(name);
|
21
|
+
if(myaccount != null) return -7;
|
22
|
+
else{
|
23
|
+
myaccount=new Account(name);
|
24
|
+
customer.put(name,myaccount);
|
25
|
+
return 0;
|
26
|
+
}
|
27
|
+
}
|
28
|
+
|
29
|
+
public int close(String name){ // 口座解約
|
30
|
+
Account myaccount = customer.get(name);
|
31
|
+
if(myaccount != null){
|
32
|
+
if(myaccount.showBalance() == 0){
|
33
|
+
customer.remove(name);
|
34
|
+
return 0;
|
35
|
+
}else return -1;
|
36
|
+
}else return -7;
|
37
|
+
}
|
38
|
+
|
39
|
+
|
40
|
+
public int withdraw(String name,int amount){ // 引き出し
|
41
|
+
Account myaccount = customer.get(name);
|
42
|
+
balance = myaccount.showBalance();
|
43
|
+
if(customer.get(name) != null){
|
44
|
+
if(amount <= 0)
|
45
|
+
return -3;
|
46
|
+
else if(amount > balance)
|
47
|
+
return -1;
|
48
|
+
else{
|
49
|
+
myaccount.withdraw(amount);
|
50
|
+
return 0;
|
101
51
|
}
|
102
|
-
|
103
|
-
|
104
|
-
|
105
|
-
|
106
|
-
|
107
|
-
|
108
|
-
|
109
|
-
|
110
|
-
|
111
|
-
|
112
|
-
|
113
|
-
|
114
|
-
|
115
|
-
|
116
|
-
|
117
|
-
|
118
|
-
|
119
|
-
|
120
|
-
|
121
|
-
|
122
|
-
|
123
|
-
|
124
|
-
|
125
|
-
|
126
|
-
|
127
|
-
|
128
|
-
|
129
|
-
|
130
|
-
|
131
|
-
|
132
|
-
|
133
|
-
|
134
|
-
|
135
|
-
|
136
|
-
|
137
|
-
|
138
|
-
|
139
|
-
|
140
|
-
|
141
|
-
|
142
|
-
|
143
|
-
|
144
|
-
|
145
|
-
|
146
|
-
|
147
|
-
|
148
|
-
|
149
|
-
|
150
|
-
|
151
|
-
|
152
|
-
|
153
|
-
|
154
|
-
|
155
|
-
|
156
|
-
|
157
|
-
|
158
|
-
|
159
|
-
|
160
|
-
|
161
|
-
|
162
|
-
|
163
|
-
|
164
|
-
|
165
|
-
|
166
|
-
|
167
|
-
|
168
|
-
|
169
|
-
|
170
|
-
+"</html>");
|
171
|
-
}
|
172
|
-
else if(result == -7){
|
173
|
-
pw.println(
|
174
|
-
"<!DOCTYPE html>"
|
175
|
-
+"<html>"
|
176
|
-
+"<head>"
|
177
|
-
+"<meta charset=\"UTF-8\">"
|
178
|
-
+"</head>"
|
179
|
-
+"<body>"
|
180
|
-
+"<div class=\"main\">"
|
181
|
-
+"<h1>引出失敗</h1>"
|
182
|
-
+"<h2>"+ b_name +"様の口座は存在しません。</h2>"
|
183
|
-
+"</div>"
|
184
|
-
+"<a class=\"success\" href=\"index.html\">メインメニューに戻る</a>"
|
185
|
-
+"</body>"
|
186
|
-
+"</html>");
|
187
|
-
}
|
188
|
-
}
|
189
|
-
// 残高照会処理
|
190
|
-
if(value.equals("balance")){
|
191
|
-
int result = bank.showBalance(b_name);
|
192
|
-
if(result >= 0){
|
193
|
-
pw.println(
|
194
|
-
"<!DOCTYPE html>"
|
195
|
-
+"<html>"
|
196
|
-
+"<head>"
|
197
|
-
+"<meta charset=\"UTF-8\">"
|
198
|
-
+"</head>"
|
199
|
-
+"<body>"
|
200
|
-
+"<div class=\"main\">"
|
201
|
-
+"<h1>残高照会成功</h1>"
|
202
|
-
+"<h2>"+ b_name +"様の口座残高は"+ result +"。</h2>"
|
203
|
-
+"</div>"
|
204
|
-
+"<a class=\"success\" href=\"index.html\">メインメニューに戻る</a>"
|
205
|
-
+"</body>"
|
206
|
-
+"</html>");
|
207
|
-
}
|
208
|
-
else{
|
209
|
-
pw.println(
|
210
|
-
"<!DOCTYPE html>"
|
211
|
-
+"<html>"
|
212
|
-
+"<head>"
|
213
|
-
+"<meta charset=\"UTF-8\">"
|
214
|
-
+"</head>"
|
215
|
-
+"<body>"
|
216
|
-
+"<div class=\"main\">"
|
217
|
-
+"<h1>残高照会失敗</h1>"
|
218
|
-
+"<h2>"+ b_name +"様の口座残高照会に失敗しました。</h2>"
|
219
|
-
+"</div>"
|
220
|
-
+"<a class=\"success\" href=\"index.html\">メインメニューに戻る</a>"
|
221
|
-
+"</body>"
|
222
|
-
+"</html>");
|
223
|
-
}
|
224
|
-
}
|
52
|
+
}else return -7;
|
53
|
+
}
|
54
|
+
|
55
|
+
|
56
|
+
public int deposit(String name,int amount){ // 預金
|
57
|
+
Account myaccount = customer.get(name);
|
58
|
+
if(customer.get(name) == null)
|
59
|
+
return -7;
|
60
|
+
else if(amount <= 0)
|
61
|
+
return -3;
|
62
|
+
else{
|
63
|
+
myaccount.deposit(amount);
|
64
|
+
return 0;
|
65
|
+
}
|
66
|
+
}
|
67
|
+
|
68
|
+
|
69
|
+
public int showBalance(String name){ // 残高照会
|
70
|
+
Account myaccount = customer.get(name);
|
71
|
+
if(customer.get(name) != null) return myaccount.showBalance();
|
72
|
+
else return -7;
|
73
|
+
}
|
74
|
+
|
75
|
+
// ExtendedBank要素
|
76
|
+
// 預金
|
77
|
+
public int deposit(String name,String amount){ // name:口座名
|
78
|
+
int result;
|
79
|
+
int d_result;
|
80
|
+
try{
|
81
|
+
result = Integer.parseInt(amount);
|
82
|
+
}catch(NumberFormatException e){
|
83
|
+
if(showBalance(name) == -7)
|
84
|
+
return -7;
|
85
|
+
else
|
86
|
+
return -4;
|
87
|
+
}
|
88
|
+
|
89
|
+
result = Integer.parseInt(amount);
|
90
|
+
if(result <= 0)
|
91
|
+
return -3;
|
92
|
+
else{
|
93
|
+
d_result=deposit(name,amount);
|
94
|
+
return d_result;
|
95
|
+
}
|
96
|
+
}
|
97
|
+
|
98
|
+
// 引き出し
|
99
|
+
public int withdraw(String name,String amount){ // name:口座名
|
100
|
+
int result;
|
101
|
+
int w_result;
|
102
|
+
try{
|
103
|
+
result = Integer.parseInt(amount);
|
104
|
+
}catch(NumberFormatException e){
|
105
|
+
if(showBalance(name) == -7)
|
106
|
+
return -7;
|
107
|
+
else
|
108
|
+
return -4;
|
109
|
+
}
|
110
|
+
|
111
|
+
result = Integer.parseInt(amount);
|
112
|
+
if(result <= 0)
|
113
|
+
return -3;
|
114
|
+
else if(result > balance)
|
115
|
+
return -1;
|
116
|
+
else{
|
117
|
+
w_result=withdraw(name,amount);
|
118
|
+
return w_result;
|
119
|
+
}
|
225
120
|
}
|
226
121
|
}
|
227
122
|
```
|
123
|
+
```java
|
124
|
+
public class Account{
|
125
|
+
private String name; //口座名
|
126
|
+
private int balance; //口座の残高
|
228
127
|
|
128
|
+
public Account(String myName){ //口座名
|
129
|
+
name = myName;
|
130
|
+
balance = 0;
|
131
|
+
}
|
132
|
+
|
133
|
+
public int deposit(int amount){ //預金額
|
134
|
+
if(amount <= 0)
|
135
|
+
return -3;
|
136
|
+
else
|
137
|
+
balance += amount;
|
138
|
+
return 0;
|
139
|
+
}
|
140
|
+
|
141
|
+
public int withdraw(int amount){ //出金額
|
142
|
+
if(amount <= 0)
|
143
|
+
return -3;
|
144
|
+
else if(amount > balance)
|
145
|
+
return -1;
|
146
|
+
else
|
147
|
+
balance -= amount;
|
148
|
+
return 0;
|
149
|
+
}
|
150
|
+
|
151
|
+
public int showBalance(){ //残高照会
|
152
|
+
return balance;
|
153
|
+
}
|
154
|
+
}
|
155
|
+
|
156
|
+
```
|
157
|
+
|